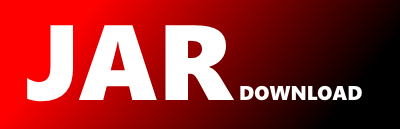
io.qt.gui.QMatrix3x3 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Java wrapper for Qt class QMatrix3x3
*/
public class QMatrix3x3 extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
public QMatrix3x3(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QMatrix3x3 instance);
public QMatrix3x3(io.qt.gui.QMatrix3x3 other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QMatrix3x3 instance, io.qt.gui.QMatrix3x3 other);
public QMatrix3x3(java.nio.FloatBuffer values){
super((QPrivateConstructor)null);
initialize_native(this, values);
}
private native static void initialize_native(QMatrix3x3 instance, java.nio.FloatBuffer values);
@io.qt.QtUninvokable
public final void copyDataTo(java.nio.FloatBuffer values){
copyDataTo_native_float_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), values);
}
@io.qt.QtUninvokable
private native void copyDataTo_native_float_ptr_constfct(long __this__nativeId, java.nio.FloatBuffer values);
@io.qt.QtUninvokable
public final java.nio.FloatBuffer data(){
return data_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.nio.FloatBuffer data_native(long __this__nativeId);
@io.qt.QtUninvokable
public final float[] array(){
return array_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native float[] array_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final void fill(float value){
fill_native_float(QtJambi_LibraryUtilities.internal.nativeId(this), value);
}
@io.qt.QtUninvokable
private native void fill_native_float(long __this__nativeId, float value);
@io.qt.QtUninvokable
public final boolean isIdentity(){
return isIdentity_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isIdentity_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final void setValue(int row, int column, float value){
setValue_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), row, column, value);
}
@io.qt.QtUninvokable
private native void setValue_native_int_int(long __this__nativeId, int row, int column, float value);
@io.qt.QtUninvokable
public final float value(int row, int column){
return value_native_int_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row, column);
}
@io.qt.QtUninvokable
private native float value_native_int_int_constfct(long __this__nativeId, int row, int column);
@io.qt.QtUninvokable
public final QMatrix3x3 multiply(float factor){
multiply_native_float(QtJambi_LibraryUtilities.internal.nativeId(this), factor);
return this;
}
@io.qt.QtUninvokable
private native QMatrix3x3 multiply_native_float(long __this__nativeId, float factor);
@io.qt.QtUninvokable
public final io.qt.gui.QMatrix3x3 add(io.qt.gui.QMatrix3x3 other){
add_native_cref_QMatrix3x3(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
return this;
}
@io.qt.QtUninvokable
private native io.qt.gui.QMatrix3x3 add_native_cref_QMatrix3x3(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final io.qt.gui.QMatrix3x3 subtract(io.qt.gui.QMatrix3x3 other){
subtract_native_cref_QMatrix3x3(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
return this;
}
@io.qt.QtUninvokable
private native io.qt.gui.QMatrix3x3 subtract_native_cref_QMatrix3x3(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final QMatrix3x3 divide(float divisor){
divide_native_float(QtJambi_LibraryUtilities.internal.nativeId(this), divisor);
return this;
}
@io.qt.QtUninvokable
private native QMatrix3x3 divide_native_float(long __this__nativeId, float divisor);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QMatrix3x3 other){
return operator_equal_native_cref_QMatrix3x3_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QMatrix3x3_constfct(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final void setToIdentity(){
setToIdentity_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void setToIdentity_native(long __this__nativeId);
@io.qt.QtUninvokable
public final io.qt.gui.QMatrix3x3 transposed(){
return transposed_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QMatrix3x3 transposed_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QMatrix3x3(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QMatrix3x3) {
return operator_equal((io.qt.gui.QMatrix3x3) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
// TEMPLATE - gui.matrix_constructor - START
private static java.nio.FloatBuffer wrap(float[] values){
if(values.length < 9)
throw new ArrayIndexOutOfBoundsException(9);
return java.nio.FloatBuffer.wrap(values);
}
public QMatrix3x3(float[] values){
this(wrap(values));
}
@io.qt.QtUninvokable
public final void copyDataTo(float[] values) {
copyDataTo(wrap(values));
}
// TEMPLATE - gui.matrix_constructor - END
@Override
public QMatrix3x3 clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QMatrix3x3 clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy