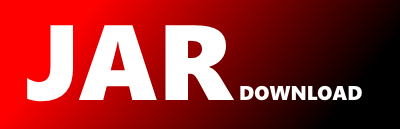
io.qt.gui.QNativeGestureEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Contains parameters that describe a gesture event
* Java wrapper for Qt class QNativeGestureEvent
*/
public class QNativeGestureEvent extends io.qt.gui.QSinglePointEvent
{
/**
* @deprecated Use the other constructor
*/
@Deprecated
public QNativeGestureEvent(io.qt.core.Qt.NativeGestureType type, io.qt.gui.QPointingDevice dev, io.qt.core.QPointF localPos, io.qt.core.QPointF scenePos, io.qt.core.QPointF globalPos, double value, long sequenceId, long intArgument){
super((QPrivateConstructor)null);
initialize_native(this, type, dev, localPos, scenePos, globalPos, value, sequenceId, intArgument);
}
@Deprecated
private native static void initialize_native(QNativeGestureEvent instance, io.qt.core.Qt.NativeGestureType type, io.qt.gui.QPointingDevice dev, io.qt.core.QPointF localPos, io.qt.core.QPointF scenePos, io.qt.core.QPointF globalPos, double value, long sequenceId, long intArgument);
/**
* Overloaded constructor for {@link #QNativeGestureEvent(io.qt.core.Qt.NativeGestureType, io.qt.gui.QPointingDevice, int, io.qt.core.QPointF, io.qt.core.QPointF, io.qt.core.QPointF, double, io.qt.core.QPointF, long)}
* with sequenceId = Long.MAX_VALUE
.
*/
public QNativeGestureEvent(io.qt.core.Qt.NativeGestureType type, io.qt.gui.QPointingDevice dev, int fingerCount, io.qt.core.QPointF localPos, io.qt.core.QPointF scenePos, io.qt.core.QPointF globalPos, double value, io.qt.core.QPointF delta) {
this(type, dev, fingerCount, localPos, scenePos, globalPos, value, delta, (long)Long.MAX_VALUE);
}
/**
*
*/
public QNativeGestureEvent(io.qt.core.Qt.NativeGestureType type, io.qt.gui.QPointingDevice dev, int fingerCount, io.qt.core.QPointF localPos, io.qt.core.QPointF scenePos, io.qt.core.QPointF globalPos, double value, io.qt.core.QPointF delta, long sequenceId){
super((QPrivateConstructor)null);
initialize_native(this, type, dev, fingerCount, localPos, scenePos, globalPos, value, delta, sequenceId);
}
private native static void initialize_native(QNativeGestureEvent instance, io.qt.core.Qt.NativeGestureType type, io.qt.gui.QPointingDevice dev, int fingerCount, io.qt.core.QPointF localPos, io.qt.core.QPointF scenePos, io.qt.core.QPointF globalPos, double value, io.qt.core.QPointF delta, long sequenceId);
protected QNativeGestureEvent(io.qt.gui.QNativeGestureEvent arg__1){
super((QPrivateConstructor)null);
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
initialize_native(this, arg__1);
}
private native static void initialize_native(QNativeGestureEvent instance, io.qt.gui.QNativeGestureEvent arg__1);
/**
* See QNativeGestureEvent::delta()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF delta(){
return delta_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF delta_native_constfct(long __this__nativeId);
/**
* See QNativeGestureEvent::fingerCount()const
*/
@io.qt.QtUninvokable
public final int fingerCount(){
return fingerCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int fingerCount_native_constfct(long __this__nativeId);
/**
* See QNativeGestureEvent::gestureType()const
*/
@io.qt.QtUninvokable
public final io.qt.core.Qt.NativeGestureType gestureType(){
return io.qt.core.Qt.NativeGestureType.resolve(gestureType_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int gestureType_native_constfct(long __this__nativeId);
/**
* @deprecated Use globalPosition().toPoint()
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QPoint globalPos(){
return globalPos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native io.qt.core.QPoint globalPos_native_constfct(long __this__nativeId);
/**
* @deprecated Use {@link io.qt.gui.QSinglePointEvent#position()} instead
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QPointF localPos(){
return localPos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native io.qt.core.QPointF localPos_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void set(io.qt.gui.QNativeGestureEvent other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
set_native_cref_QNativeGestureEvent(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void set_native_cref_QNativeGestureEvent(long __this__nativeId, long other);
/**
* @deprecated Use position().toPoint()
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QPoint pos(){
return pos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native io.qt.core.QPoint pos_native_constfct(long __this__nativeId);
/**
* @deprecated Use {@link io.qt.gui.QSinglePointEvent#globalPosition()} instead
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QPointF screenPos(){
return screenPos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native io.qt.core.QPointF screenPos_native_constfct(long __this__nativeId);
/**
* See QNativeGestureEvent::value()const
*/
@io.qt.QtUninvokable
public final double value(){
return value_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double value_native_constfct(long __this__nativeId);
/**
* @deprecated Use {@link io.qt.gui.QSinglePointEvent#scenePosition()} instead
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QPointF windowPos(){
return windowPos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native io.qt.core.QPointF windowPos_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public io.qt.gui.QNativeGestureEvent clone(){
return clone_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QNativeGestureEvent clone_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void setRealValue(double realValue){
setRealValue_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), realValue);
}
@io.qt.QtUninvokable
private native void setRealValue_native_qtjambireal(long __this__nativeId, double realValue);
@io.qt.QtUninvokable
protected final double realValue(){
return realValue_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double realValue_native(long __this__nativeId);
@io.qt.QtUninvokable
protected final void setSequenceId(long sequenceId){
setSequenceId_native_unsigned_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), sequenceId);
}
@io.qt.QtUninvokable
private native void setSequenceId_native_unsigned_long_long(long __this__nativeId, long sequenceId);
@io.qt.QtUninvokable
protected final long sequenceId(){
return sequenceId_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long sequenceId_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QNativeGestureEvent(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public String toString() {
return toString_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private static native String toString_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy