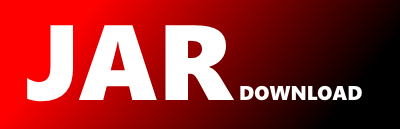
io.qt.gui.QPageRanges Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Represents a collection of page ranges
* Java wrapper for Qt class QPageRanges
*/
public class QPageRanges extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* QPageRanges::Range struct holds the from and to endpoints of a range
* Java wrapper for Qt class QPageRanges::Range
*/
public static class Range extends io.qt.QtObject
implements java.lang.Comparable,
java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
public Range(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(Range instance);
/**
* See QPageRanges::Range::contains(int)const
*/
@io.qt.QtUninvokable
public final boolean contains(int pageNumber){
return contains_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), pageNumber);
}
@io.qt.QtUninvokable
private native boolean contains_native_int_constfct(long __this__nativeId, int pageNumber);
@io.qt.QtUninvokable
private final boolean operator_less(io.qt.gui.QPageRanges.Range rhs){
return operator_less_native_QPageRanges_Range(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(rhs));
}
@io.qt.QtUninvokable
private native boolean operator_less_native_QPageRanges_Range(long __this__nativeId, long rhs);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QPageRanges.Range rhs){
return operator_equal_native_QPageRanges_Range(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(rhs));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_QPageRanges_Range(long __this__nativeId, long rhs);
/**
* The lower endpoint of the range
*
*/
@io.qt.QtUninvokable
public final void setFrom(int from){
setFrom_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), from);
}
@io.qt.QtUninvokable
private native void setFrom_native_int(long __this__nativeId, int from);
/**
* The lower endpoint of the range
*
*/
@io.qt.QtUninvokable
public final int from(){
return from_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int from_native(long __this__nativeId);
/**
* The upper endpoint of the range
*
*/
@io.qt.QtUninvokable
public final void setTo(int to){
setTo_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), to);
}
@io.qt.QtUninvokable
private native void setTo_native_int(long __this__nativeId, int to);
/**
* The upper endpoint of the range
*
*/
@io.qt.QtUninvokable
public final int to(){
return to_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int to_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected Range(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QPageRanges.Range) {
return operator_equal((io.qt.gui.QPageRanges.Range) other);
}
return false;
}
@io.qt.QtUninvokable
public int compareTo(io.qt.gui.QPageRanges.Range other) {
if (equals(other)) return 0;
else if (operator_less(other)) return -1;
else return 1;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
public Range clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native Range clone_native(long __this_nativeId);
}
/**
* See QPageRanges::QPageRanges()
*/
public QPageRanges(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPageRanges instance);
/**
* See QPageRanges::QPageRanges(QPageRanges)
*/
public QPageRanges(io.qt.gui.QPageRanges other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QPageRanges instance, io.qt.gui.QPageRanges other);
/**
*
*/
@io.qt.QtUninvokable
public final void addPage(int pageNumber){
addPage_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), pageNumber);
}
@io.qt.QtUninvokable
private native void addPage_native_int(long __this__nativeId, int pageNumber);
/**
* See QPageRanges::addRange(int,int)
*/
@io.qt.QtUninvokable
public final void addRange(int from, int to){
addRange_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), from, to);
}
@io.qt.QtUninvokable
private native void addRange_native_int_int(long __this__nativeId, int from, int to);
/**
*
*/
@io.qt.QtUninvokable
public final void clear(){
clear_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void clear_native(long __this__nativeId);
/**
* See QPageRanges::contains(int)const
*/
@io.qt.QtUninvokable
public final boolean contains(int pageNumber){
return contains_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), pageNumber);
}
@io.qt.QtUninvokable
private native boolean contains_native_int_constfct(long __this__nativeId, int pageNumber);
@io.qt.QtUninvokable
public final void detach(){
detach_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void detach_native(long __this__nativeId);
/**
* See QPageRanges::firstPage()const
*/
@io.qt.QtUninvokable
public final int firstPage(){
return firstPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int firstPage_native_constfct(long __this__nativeId);
/**
* See QPageRanges::isEmpty()const
*/
@io.qt.QtUninvokable
public final boolean isEmpty(){
return isEmpty_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isEmpty_native_constfct(long __this__nativeId);
/**
* See QPageRanges::lastPage()const
*/
@io.qt.QtUninvokable
public final int lastPage(){
return lastPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int lastPage_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public void writeTo(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
writeTo_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void writeTo_native_ref_QDataStream(long __this__nativeId, long arg__1);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QPageRanges rhs){
return operator_equal_native_cref_QPageRanges(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(rhs));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QPageRanges(long __this__nativeId, long rhs);
@io.qt.QtUninvokable
public void readFrom(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
readFrom_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void readFrom_native_ref_QDataStream(long __this__nativeId, long arg__1);
@io.qt.QtUninvokable
public final void swap(io.qt.gui.QPageRanges other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QPageRanges(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void swap_native_ref_QPageRanges(long __this__nativeId, long other);
/**
* See QPageRanges::toRangeList()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList toRangeList(){
return toRangeList_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList toRangeList_native_constfct(long __this__nativeId);
/**
* See QPageRanges::toString()const
*/
@io.qt.QtUninvokable
public final java.lang.String toString(){
return toString_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String toString_native_constfct(long __this__nativeId);
/**
* See QPageRanges::fromString(QString)
*/
public native static io.qt.gui.QPageRanges fromString(java.lang.String ranges);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPageRanges(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QPageRanges) {
return operator_equal((io.qt.gui.QPageRanges) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
public QPageRanges clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QPageRanges clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy