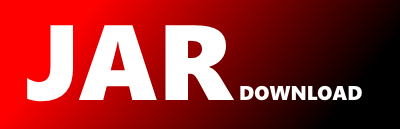
io.qt.gui.QPixelFormat Maven / Gradle / Ivy
Show all versions of qtjambi Show documentation
package io.qt.gui;
/**
* Class for describing different pixel layouts in graphics buffers
* Java wrapper for Qt class QPixelFormat
*/
public class QPixelFormat extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QPixelFormat::AlphaPosition
*/
public enum AlphaPosition implements io.qt.QtEnumerator {
AtBeginning(0),
AtEnd(1);
private AlphaPosition(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AlphaPosition resolve(int value) {
switch (value) {
case 0: return AtBeginning;
case 1: return AtEnd;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::AlphaPremultiplied
*/
public enum AlphaPremultiplied implements io.qt.QtEnumerator {
NotPremultiplied(0),
Premultiplied(1);
private AlphaPremultiplied(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AlphaPremultiplied resolve(int value) {
switch (value) {
case 0: return NotPremultiplied;
case 1: return Premultiplied;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::AlphaUsage
*/
public enum AlphaUsage implements io.qt.QtEnumerator {
UsesAlpha(0),
IgnoresAlpha(1);
private AlphaUsage(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AlphaUsage resolve(int value) {
switch (value) {
case 0: return UsesAlpha;
case 1: return IgnoresAlpha;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::ByteOrder
*/
public enum ByteOrder implements io.qt.QtEnumerator {
LittleEndian(0),
BigEndian(1),
CurrentSystemEndian(2);
private ByteOrder(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ByteOrder resolve(int value) {
switch (value) {
case 0: return LittleEndian;
case 1: return BigEndian;
case 2: return CurrentSystemEndian;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::ColorModel
*/
public enum ColorModel implements io.qt.QtEnumerator {
RGB(0),
BGR(1),
Indexed(2),
Grayscale(3),
CMYK(4),
HSL(5),
HSV(6),
YUV(7),
Alpha(8);
private ColorModel(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ColorModel resolve(int value) {
switch (value) {
case 0: return RGB;
case 1: return BGR;
case 2: return Indexed;
case 3: return Grayscale;
case 4: return CMYK;
case 5: return HSL;
case 6: return HSV;
case 7: return YUV;
case 8: return Alpha;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::TypeInterpretation
*/
public enum TypeInterpretation implements io.qt.QtEnumerator {
UnsignedInteger(0),
UnsignedShort(1),
UnsignedByte(2),
FloatingPoint(3);
private TypeInterpretation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TypeInterpretation resolve(int value) {
switch (value) {
case 0: return UnsignedInteger;
case 1: return UnsignedShort;
case 2: return UnsignedByte;
case 3: return FloatingPoint;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPixelFormat::YUVLayout
*/
public enum YUVLayout implements io.qt.QtEnumerator {
YUV444(0),
YUV422(1),
YUV411(2),
YUV420P(3),
YUV420SP(4),
YV12(5),
UYVY(6),
YUYV(7),
NV12(8),
NV21(9),
IMC1(10),
IMC2(11),
IMC3(12),
IMC4(13),
Y8(14),
Y16(15);
private YUVLayout(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static YUVLayout resolve(int value) {
switch (value) {
case 0: return YUV444;
case 1: return YUV422;
case 2: return YUV411;
case 3: return YUV420P;
case 4: return YUV420SP;
case 5: return YV12;
case 6: return UYVY;
case 7: return YUYV;
case 8: return NV12;
case 9: return NV21;
case 10: return IMC1;
case 11: return IMC2;
case 12: return IMC3;
case 13: return IMC4;
case 14: return Y8;
case 15: return Y16;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QPixelFormat::QPixelFormat()
*/
public QPixelFormat(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPixelFormat instance);
/**
* Overloaded constructor for {@link #QPixelFormat(io.qt.gui.QPixelFormat.ColorModel, byte, byte, byte, byte, byte, byte, io.qt.gui.QPixelFormat.AlphaUsage, io.qt.gui.QPixelFormat.AlphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied, io.qt.gui.QPixelFormat.TypeInterpretation, io.qt.gui.QPixelFormat.ByteOrder, byte)}
* with subEnum = 0
.
*/
public QPixelFormat(io.qt.gui.QPixelFormat.ColorModel colorModel, byte firstSize, byte secondSize, byte thirdSize, byte fourthSize, byte fifthSize, byte alphaSize, io.qt.gui.QPixelFormat.AlphaUsage alphaUsage, io.qt.gui.QPixelFormat.AlphaPosition alphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied premultiplied, io.qt.gui.QPixelFormat.TypeInterpretation typeInterpretation, io.qt.gui.QPixelFormat.ByteOrder byteOrder) {
this(colorModel, firstSize, secondSize, thirdSize, fourthSize, fifthSize, alphaSize, alphaUsage, alphaPosition, premultiplied, typeInterpretation, byteOrder, (byte)0);
}
/**
* Overloaded constructor for {@link #QPixelFormat(io.qt.gui.QPixelFormat.ColorModel, byte, byte, byte, byte, byte, byte, io.qt.gui.QPixelFormat.AlphaUsage, io.qt.gui.QPixelFormat.AlphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied, io.qt.gui.QPixelFormat.TypeInterpretation, io.qt.gui.QPixelFormat.ByteOrder, byte)}
* with:
* byteOrder = io.qt.gui.QPixelFormat.ByteOrder.CurrentSystemEndian
* subEnum = 0
*
*/
public QPixelFormat(io.qt.gui.QPixelFormat.ColorModel colorModel, byte firstSize, byte secondSize, byte thirdSize, byte fourthSize, byte fifthSize, byte alphaSize, io.qt.gui.QPixelFormat.AlphaUsage alphaUsage, io.qt.gui.QPixelFormat.AlphaPosition alphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied premultiplied, io.qt.gui.QPixelFormat.TypeInterpretation typeInterpretation) {
this(colorModel, firstSize, secondSize, thirdSize, fourthSize, fifthSize, alphaSize, alphaUsage, alphaPosition, premultiplied, typeInterpretation, io.qt.gui.QPixelFormat.ByteOrder.CurrentSystemEndian, (byte)0);
}
/**
*
*/
public QPixelFormat(io.qt.gui.QPixelFormat.ColorModel colorModel, byte firstSize, byte secondSize, byte thirdSize, byte fourthSize, byte fifthSize, byte alphaSize, io.qt.gui.QPixelFormat.AlphaUsage alphaUsage, io.qt.gui.QPixelFormat.AlphaPosition alphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied premultiplied, io.qt.gui.QPixelFormat.TypeInterpretation typeInterpretation, io.qt.gui.QPixelFormat.ByteOrder byteOrder, byte subEnum){
super((QPrivateConstructor)null);
initialize_native(this, colorModel, firstSize, secondSize, thirdSize, fourthSize, fifthSize, alphaSize, alphaUsage, alphaPosition, premultiplied, typeInterpretation, byteOrder, subEnum);
}
private native static void initialize_native(QPixelFormat instance, io.qt.gui.QPixelFormat.ColorModel colorModel, byte firstSize, byte secondSize, byte thirdSize, byte fourthSize, byte fifthSize, byte alphaSize, io.qt.gui.QPixelFormat.AlphaUsage alphaUsage, io.qt.gui.QPixelFormat.AlphaPosition alphaPosition, io.qt.gui.QPixelFormat.AlphaPremultiplied premultiplied, io.qt.gui.QPixelFormat.TypeInterpretation typeInterpretation, io.qt.gui.QPixelFormat.ByteOrder byteOrder, byte subEnum);
/**
* See QPixelFormat::alphaPosition()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.AlphaPosition alphaPosition(){
return io.qt.gui.QPixelFormat.AlphaPosition.resolve(alphaPosition_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int alphaPosition_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::alphaSize()const
*/
@io.qt.QtUninvokable
public final byte alphaSize(){
return alphaSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte alphaSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::alphaUsage()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.AlphaUsage alphaUsage(){
return io.qt.gui.QPixelFormat.AlphaUsage.resolve(alphaUsage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int alphaUsage_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::bitsPerPixel()const
*/
@io.qt.QtUninvokable
public final byte bitsPerPixel(){
return bitsPerPixel_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte bitsPerPixel_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::blackSize()const
*/
@io.qt.QtUninvokable
public final byte blackSize(){
return blackSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte blackSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::blueSize()const
*/
@io.qt.QtUninvokable
public final byte blueSize(){
return blueSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte blueSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::brightnessSize()const
*/
@io.qt.QtUninvokable
public final byte brightnessSize(){
return brightnessSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte brightnessSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::byteOrder()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.ByteOrder byteOrder(){
return io.qt.gui.QPixelFormat.ByteOrder.resolve(byteOrder_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int byteOrder_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::channelCount()const
*/
@io.qt.QtUninvokable
public final byte channelCount(){
return channelCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte channelCount_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::colorModel()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.ColorModel colorModel(){
return io.qt.gui.QPixelFormat.ColorModel.resolve(colorModel_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int colorModel_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::cyanSize()const
*/
@io.qt.QtUninvokable
public final byte cyanSize(){
return cyanSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte cyanSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::greenSize()const
*/
@io.qt.QtUninvokable
public final byte greenSize(){
return greenSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte greenSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::hueSize()const
*/
@io.qt.QtUninvokable
public final byte hueSize(){
return hueSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte hueSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::lightnessSize()const
*/
@io.qt.QtUninvokable
public final byte lightnessSize(){
return lightnessSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte lightnessSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::magentaSize()const
*/
@io.qt.QtUninvokable
public final byte magentaSize(){
return magentaSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte magentaSize_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QPixelFormat fmt2){
return operator_equal_native_QPixelFormat(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(fmt2));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_QPixelFormat(long __this__nativeId, long fmt2);
/**
* See QPixelFormat::premultiplied()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.AlphaPremultiplied premultiplied(){
return io.qt.gui.QPixelFormat.AlphaPremultiplied.resolve(premultiplied_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int premultiplied_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::redSize()const
*/
@io.qt.QtUninvokable
public final byte redSize(){
return redSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte redSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::saturationSize()const
*/
@io.qt.QtUninvokable
public final byte saturationSize(){
return saturationSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte saturationSize_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final byte subEnum(){
return subEnum_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte subEnum_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::typeInterpretation()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.TypeInterpretation typeInterpretation(){
return io.qt.gui.QPixelFormat.TypeInterpretation.resolve(typeInterpretation_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int typeInterpretation_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::yellowSize()const
*/
@io.qt.QtUninvokable
public final byte yellowSize(){
return yellowSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native byte yellowSize_native_constfct(long __this__nativeId);
/**
* See QPixelFormat::yuvLayout()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixelFormat.YUVLayout yuvLayout(){
return io.qt.gui.QPixelFormat.YUVLayout.resolve(yuvLayout_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int yuvLayout_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPixelFormat(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QPixelFormat) {
return operator_equal((io.qt.gui.QPixelFormat) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
public QPixelFormat clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QPixelFormat clone_native(long __this_nativeId);
}