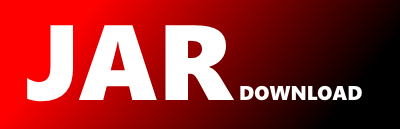
io.qt.gui.QPixmapCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Application-wide cache for pixmaps
* Java wrapper for Qt class QPixmapCache
*/
public class QPixmapCache extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* QPixmapCache::Key class can be used for efficient access to the QPixmapCache
* Java wrapper for Qt class QPixmapCache::Key
*/
public static class Key extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
*
*/
public Key(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(Key instance);
public Key(io.qt.gui.QPixmapCache.Key other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(Key instance, io.qt.gui.QPixmapCache.Key other);
/**
* See QPixmapCache::Key::isValid()const
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QPixmapCache.Key key){
return operator_equal_native_cref_QPixmapCache_Key_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(key));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QPixmapCache_Key_constfct(long __this__nativeId, long key);
@io.qt.QtUninvokable
public final void swap(io.qt.gui.QPixmapCache.Key other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QPixmapCache_Key(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void swap_native_ref_QPixmapCache_Key(long __this__nativeId, long other);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected Key(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QPixmapCache.Key) {
return operator_equal((io.qt.gui.QPixmapCache.Key) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
public Key clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native Key clone_native(long __this_nativeId);
}
public QPixmapCache(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPixmapCache instance);
/**
* See QPixmapCache::cacheLimit()
*/
public native static int cacheLimit();
/**
*
*/
public native static void clear();
/**
* See QPixmapCache::find(QPixmapCache::Key,QPixmap*)
*/
public static io.qt.gui.QPixmap find(io.qt.gui.QPixmapCache.Key key){
return find_native_cref_QPixmapCache_Key_QPixmap_ptr(QtJambi_LibraryUtilities.internal.checkedNativeId(key));
}
private native static io.qt.gui.QPixmap find_native_cref_QPixmapCache_Key_QPixmap_ptr(long key);
/**
* See QPixmapCache::find(QString,QPixmap*)
*/
public static io.qt.gui.QPixmap find(java.lang.String key){
return find_native_cref_QString_QPixmap_ptr(key);
}
private native static io.qt.gui.QPixmap find_native_cref_QString_QPixmap_ptr(java.lang.String key);
/**
* See QPixmapCache::insert(QPixmap)
*/
public static io.qt.gui.QPixmapCache.Key insert(io.qt.gui.QPixmap pixmap){
return insert_native_cref_QPixmap(QtJambi_LibraryUtilities.internal.checkedNativeId(pixmap));
}
private native static io.qt.gui.QPixmapCache.Key insert_native_cref_QPixmap(long pixmap);
/**
* See QPixmapCache::insert(QString,QPixmap)
*/
public static boolean insert(java.lang.String key, io.qt.gui.QPixmap pixmap){
return insert_native_cref_QString_cref_QPixmap(key, QtJambi_LibraryUtilities.internal.checkedNativeId(pixmap));
}
private native static boolean insert_native_cref_QString_cref_QPixmap(java.lang.String key, long pixmap);
/**
* See QPixmapCache::remove(QPixmapCache::Key)
*/
public static void remove(io.qt.gui.QPixmapCache.Key key){
remove_native_cref_QPixmapCache_Key(QtJambi_LibraryUtilities.internal.checkedNativeId(key));
}
private native static void remove_native_cref_QPixmapCache_Key(long key);
/**
* See QPixmapCache::remove(QString)
*/
public native static void remove(java.lang.String key);
/**
* See QPixmapCache::replace(QPixmapCache::Key,QPixmap)
*/
public static boolean replace(io.qt.gui.QPixmapCache.Key key, io.qt.gui.QPixmap pixmap){
return replace_native_cref_QPixmapCache_Key_cref_QPixmap(QtJambi_LibraryUtilities.internal.checkedNativeId(key), QtJambi_LibraryUtilities.internal.checkedNativeId(pixmap));
}
private native static boolean replace_native_cref_QPixmapCache_Key_cref_QPixmap(long key, long pixmap);
/**
* See QPixmapCache::setCacheLimit(int)
*/
public native static void setCacheLimit(int arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPixmapCache(QPrivateConstructor p) { super(p); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy