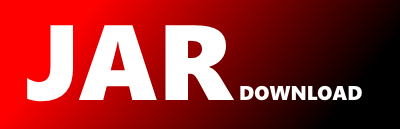
io.qt.gui.QTextLength Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Encapsulates the different types of length used in a QTextDocument
* Java wrapper for Qt class QTextLength
*/
public class QTextLength extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QTextLength::Type
*/
public enum Type implements io.qt.QtEnumerator {
VariableLength(0),
FixedLength(1),
PercentageLength(2);
private Type(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Type resolve(int value) {
switch (value) {
case 0: return VariableLength;
case 1: return FixedLength;
case 2: return PercentageLength;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QTextLength::QTextLength()
*/
public QTextLength(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QTextLength instance);
/**
* See QTextLength::QTextLength(QTextLength::Type,qreal)
*/
public QTextLength(io.qt.gui.QTextLength.Type type, double value){
super((QPrivateConstructor)null);
initialize_native(this, type, value);
}
private native static void initialize_native(QTextLength instance, io.qt.gui.QTextLength.Type type, double value);
@io.qt.QtUninvokable
public void writeTo(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
writeTo_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void writeTo_native_ref_QDataStream(long __this__nativeId, long arg__1);
/**
* See QTextLength::operator==(QTextLength)const
*/
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.gui.QTextLength other){
return operator_equal_native_cref_QTextLength_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QTextLength_constfct(long __this__nativeId, long other);
@io.qt.QtUninvokable
public void readFrom(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
readFrom_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void readFrom_native_ref_QDataStream(long __this__nativeId, long arg__1);
/**
* See QTextLength::rawValue()const
*/
@io.qt.QtUninvokable
public final double rawValue(){
return rawValue_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double rawValue_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.gui.QTextLength.Type type(){
return io.qt.gui.QTextLength.Type.resolve(type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
* See QTextLength::value(qreal)const
*/
@io.qt.QtUninvokable
public final double value(double maximumLength){
return value_native_qtjambireal_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), maximumLength);
}
@io.qt.QtUninvokable
private native double value_native_qtjambireal_constfct(long __this__nativeId, double maximumLength);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QTextLength(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.gui.QTextLength) {
return operator_equal((io.qt.gui.QTextLength) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
@io.qt.QtUninvokable
public String toString() {
return toString_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private static native String toString_native(long __this_nativeId);
@Override
public QTextLength clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QTextLength clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy