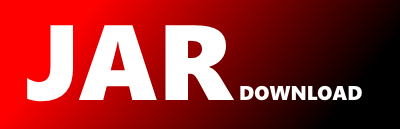
io.qt.gui.QTextListFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Formatting information for lists in a QTextDocument
* Java wrapper for Qt class QTextListFormat
*/
public class QTextListFormat extends io.qt.gui.QTextFormat
implements java.lang.Cloneable
{
/**
* Java wrapper for Qt enum QTextListFormat::Style
*/
public enum Style implements io.qt.QtEnumerator {
ListDisc(-1),
ListCircle(-2),
ListSquare(-3),
ListDecimal(-4),
ListLowerAlpha(-5),
ListUpperAlpha(-6),
ListLowerRoman(-7),
ListUpperRoman(-8),
ListStyleUndefined(0);
private Style(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Style resolve(int value) {
switch (value) {
case -1: return ListDisc;
case -2: return ListCircle;
case -3: return ListSquare;
case -4: return ListDecimal;
case -5: return ListLowerAlpha;
case -6: return ListUpperAlpha;
case -7: return ListLowerRoman;
case -8: return ListUpperRoman;
case 0: return ListStyleUndefined;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QTextListFormat::QTextListFormat()
*/
public QTextListFormat(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QTextListFormat instance);
protected QTextListFormat(io.qt.gui.QTextFormat fmt){
super((QPrivateConstructor)null);
initialize_native(this, fmt);
}
private native static void initialize_native(QTextListFormat instance, io.qt.gui.QTextFormat fmt);
/**
* See QTextListFormat::indent()const
*/
@io.qt.QtUninvokable
public final int indent(){
return indent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int indent_native_constfct(long __this__nativeId);
/**
* See QTextFormat::isValid()const
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QTextListFormat::numberPrefix()const
*/
@io.qt.QtUninvokable
public final java.lang.String numberPrefix(){
return numberPrefix_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String numberPrefix_native_constfct(long __this__nativeId);
/**
* See QTextListFormat::numberSuffix()const
*/
@io.qt.QtUninvokable
public final java.lang.String numberSuffix(){
return numberSuffix_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String numberSuffix_native_constfct(long __this__nativeId);
/**
* See QTextListFormat::setIndent(int)
*/
@io.qt.QtUninvokable
public final void setIndent(int indent){
setIndent_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), indent);
}
@io.qt.QtUninvokable
private native void setIndent_native_int(long __this__nativeId, int indent);
/**
* See QTextListFormat::setNumberPrefix(QString)
*/
@io.qt.QtUninvokable
public final void setNumberPrefix(java.lang.String numberPrefix){
setNumberPrefix_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), numberPrefix);
}
@io.qt.QtUninvokable
private native void setNumberPrefix_native_cref_QString(long __this__nativeId, java.lang.String numberPrefix);
/**
* See QTextListFormat::setNumberSuffix(QString)
*/
@io.qt.QtUninvokable
public final void setNumberSuffix(java.lang.String numberSuffix){
setNumberSuffix_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), numberSuffix);
}
@io.qt.QtUninvokable
private native void setNumberSuffix_native_cref_QString(long __this__nativeId, java.lang.String numberSuffix);
/**
* See QTextListFormat::setStyle(QTextListFormat::Style)
*/
@io.qt.QtUninvokable
public final void setStyle(io.qt.gui.QTextListFormat.Style style){
setStyle_native_QTextListFormat_Style(QtJambi_LibraryUtilities.internal.nativeId(this), style.value());
}
@io.qt.QtUninvokable
private native void setStyle_native_QTextListFormat_Style(long __this__nativeId, int style);
/**
* See QTextListFormat::style()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QTextListFormat.Style style(){
return io.qt.gui.QTextListFormat.Style.resolve(style_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int style_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QTextListFormat(QPrivateConstructor p) { super(p); }
@Override
public QTextListFormat clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QTextListFormat clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy