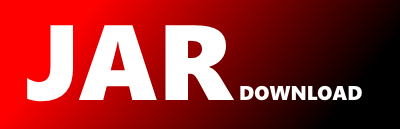
io.qt.gui.nativeinterface.QXcbWindow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui.nativeinterface;
/**
* Java wrapper for Qt class QNativeInterface::Private::QXcbWindow
*/
public interface QXcbWindow extends io.qt.QtObjectInterface
{
/**
* Java wrapper for Qt enum QNativeInterface::Private::QXcbWindow::WindowType
*
* @see WindowTypes
*/
public enum WindowType implements io.qt.QtFlagEnumerator {
None(0),
Normal(1),
Desktop(2),
Dock(4),
Toolbar(8),
Menu(16),
Utility(32),
Splash(64),
Dialog(128),
DropDownMenu(256),
PopupMenu(512),
Tooltip(1024),
Notification(2048),
Combo(4096),
Dnd(8192),
KdeOverride(16384);
private WindowType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public WindowTypes asFlags() {
return new WindowTypes(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public WindowTypes combined(WindowType e) {
return new WindowTypes(this, e);
}
/**
* Creates a new {@link WindowTypes} from the entries.
* @param values entries
* @return new flag
*/
public static WindowTypes flags(WindowType ... values) {
return new WindowTypes(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WindowType resolve(int value) {
switch (value) {
case 0: return None;
case 1: return Normal;
case 2: return Desktop;
case 4: return Dock;
case 8: return Toolbar;
case 16: return Menu;
case 32: return Utility;
case 64: return Splash;
case 128: return Dialog;
case 256: return DropDownMenu;
case 512: return PopupMenu;
case 1024: return Tooltip;
case 2048: return Notification;
case 4096: return Combo;
case 8192: return Dnd;
case 16384: return KdeOverride;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link WindowType}
*/
public static final class WindowTypes extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xc8632944e0676c99L;
/**
* Creates a new WindowTypes where the flags in args
are set.
* @param args enum entries
*/
public WindowTypes(WindowType ... args){
super(args);
}
/**
* Creates a new WindowTypes with given value
.
* @param value
*/
public WindowTypes(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new WindowTypes
*/
@Override
public final WindowTypes combined(WindowType e){
return new WindowTypes(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final WindowTypes setFlag(WindowType e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final WindowTypes setFlag(WindowType e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this WindowTypes.
* @return array of enum entries
*/
@Override
public final WindowType[] flags(){
return super.flags(WindowType.values());
}
/**
* {@inheritDoc}
*/
@Override
public final WindowTypes clone(){
return new WindowTypes(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(WindowTypes other){
return Integer.compare(value(), other.value());
}
}
/**
* Implementor class for interface {@link io.qt.gui.nativeinterface.QXcbWindow}
*/
public static abstract class Impl extends io.qt.QtObject
implements io.qt.gui.nativeinterface.QXcbWindow
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.NativeAccess
private static final class ConcreteWrapper extends QXcbWindow.Impl {
@io.qt.NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public void setWindowIconText(java.lang.String text){
throw new io.qt.QNoImplementationException();
}
private native static void setWindowIconText_native_cref_QString(long __this__nativeId, java.lang.String text);
@Override
@io.qt.QtUninvokable
public void setWindowRole(java.lang.String role){
throw new io.qt.QNoImplementationException();
}
private native static void setWindowRole_native_cref_QString(long __this__nativeId, java.lang.String role);
@Override
@io.qt.QtUninvokable
public void setWindowType(io.qt.gui.nativeinterface.QXcbWindow.WindowTypes type){
throw new io.qt.QNoImplementationException();
}
private native static void setWindowType_native_QFlags_QNativeInterface_Private_QXcbWindow_WindowType_(long __this__nativeId, int type);
@Override
@io.qt.QtUninvokable
public int visualId(){
throw new io.qt.QNoImplementationException();
}
private native static int visualId_native_constfct(long __this__nativeId);
}
@io.qt.QtUninvokable
public abstract void setWindowIconText(java.lang.String text);
@io.qt.QtUninvokable
public abstract void setWindowRole(java.lang.String role);
@io.qt.QtUninvokable
public abstract void setWindowType(io.qt.gui.nativeinterface.QXcbWindow.WindowTypes type);
@io.qt.QtUninvokable
public abstract int visualId();
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected Impl(QPrivateConstructor p) { super(p); }
}
@io.qt.QtUninvokable
public void setWindowIconText(java.lang.String text);
@io.qt.QtUninvokable
public void setWindowRole(java.lang.String role);
@io.qt.QtUninvokable
public void setWindowType(io.qt.gui.nativeinterface.QXcbWindow.WindowTypes type);
@io.qt.QtUninvokable
public int visualId();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy