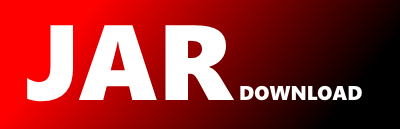
io.qt.widgets.QDial Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* Rounded range control (like a speedometer or potentiometer)
* Java wrapper for Qt class QDial
*/
public class QDial extends io.qt.widgets.QAbstractSlider
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QDial.class);
/**
* Overloaded constructor for {@link #QDial(io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QDial() {
this((io.qt.widgets.QWidget)null);
}
/**
*
*/
public QDial(io.qt.widgets.QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QDial instance, io.qt.widgets.QWidget parent);
/**
*
*/
@io.qt.QtPropertyReader(name="notchSize")
@io.qt.QtUninvokable
public final int notchSize(){
return notchSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int notchSize_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtPropertyReader(name="notchTarget")
@io.qt.QtUninvokable
public final double notchTarget(){
return notchTarget_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double notchTarget_native_constfct(long __this__nativeId);
/**
* See QDial::notchesVisible()const
*/
@io.qt.QtPropertyReader(name="notchesVisible")
@io.qt.QtUninvokable
public final boolean notchesVisible(){
return notchesVisible_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean notchesVisible_native_constfct(long __this__nativeId);
/**
* See QDial::setNotchTarget(double)
*/
@io.qt.QtPropertyWriter(name="notchTarget")
@io.qt.QtUninvokable
public final void setNotchTarget(double target){
setNotchTarget_native_double(QtJambi_LibraryUtilities.internal.nativeId(this), target);
}
@io.qt.QtUninvokable
private native void setNotchTarget_native_double(long __this__nativeId, double target);
/**
* See QDial::setNotchesVisible(bool)
*/
@io.qt.QtPropertyWriter(name="notchesVisible")
public final void setNotchesVisible(boolean visible){
setNotchesVisible_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), visible);
}
private native void setNotchesVisible_native_bool(long __this__nativeId, boolean visible);
/**
*
*/
@io.qt.QtPropertyWriter(name="wrapping")
public final void setWrapping(boolean on){
setWrapping_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), on);
}
private native void setWrapping_native_bool(long __this__nativeId, boolean on);
/**
*
*/
@io.qt.QtPropertyReader(name="wrapping")
@io.qt.QtUninvokable
public final boolean wrapping(){
return wrapping_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean wrapping_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public boolean event(io.qt.core.QEvent e){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(e));
}
@io.qt.QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long e);
/**
* See QDial::initStyleOption(QStyleOptionSlider*)const
*/
@io.qt.QtUninvokable
protected void initStyleOption(io.qt.widgets.QStyleOptionSlider option){
initStyleOption_native_QStyleOptionSlider_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), option);
}
@io.qt.QtUninvokable
private native void initStyleOption_native_QStyleOptionSlider_ptr_constfct(long __this__nativeId, io.qt.widgets.QStyleOptionSlider option);
/**
* See QWidget::minimumSizeHint()const
*/
@io.qt.QtUninvokable
public io.qt.core.QSize minimumSizeHint(){
return minimumSizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QSize minimumSizeHint_native_constfct(long __this__nativeId);
/**
* See QWidget::mouseMoveEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mouseMoveEvent(io.qt.gui.QMouseEvent me){
mouseMoveEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(me));
}
@io.qt.QtUninvokable
private native void mouseMoveEvent_native_QMouseEvent_ptr(long __this__nativeId, long me);
/**
* See QWidget::mousePressEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mousePressEvent(io.qt.gui.QMouseEvent me){
mousePressEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(me));
}
@io.qt.QtUninvokable
private native void mousePressEvent_native_QMouseEvent_ptr(long __this__nativeId, long me);
/**
* See QWidget::mouseReleaseEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mouseReleaseEvent(io.qt.gui.QMouseEvent me){
mouseReleaseEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(me));
}
@io.qt.QtUninvokable
private native void mouseReleaseEvent_native_QMouseEvent_ptr(long __this__nativeId, long me);
/**
* See QWidget::paintEvent(QPaintEvent*)
*/
@io.qt.QtUninvokable
protected void paintEvent(io.qt.gui.QPaintEvent pe){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(pe));
}
@io.qt.QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long pe);
/**
* See QWidget::resizeEvent(QResizeEvent*)
*/
@io.qt.QtUninvokable
protected void resizeEvent(io.qt.gui.QResizeEvent re){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(re));
}
@io.qt.QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long re);
/**
*
*/
@io.qt.QtUninvokable
public io.qt.core.QSize sizeHint(){
return sizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QSize sizeHint_native_constfct(long __this__nativeId);
/**
* See QAbstractSlider::sliderChange(QAbstractSlider::SliderChange)
*/
@io.qt.QtUninvokable
protected void sliderChange(io.qt.widgets.QAbstractSlider.SliderChange change){
sliderChange_native_QAbstractSlider_SliderChange(QtJambi_LibraryUtilities.internal.nativeId(this), change.value());
}
@io.qt.QtUninvokable
private native void sliderChange_native_QAbstractSlider_SliderChange(long __this__nativeId, int change);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QDial(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QDial(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QDial instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy