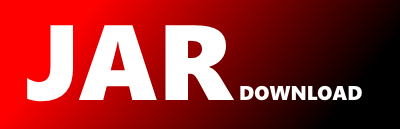
io.qt.widgets.QGestureRecognizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* The infrastructure for gesture recognition
* Java wrapper for Qt class QGestureRecognizer
*/
public abstract class QGestureRecognizer extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.NativeAccess
private static final class ConcreteWrapper extends QGestureRecognizer {
@io.qt.NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public io.qt.widgets.QGestureRecognizer.Result recognize(io.qt.widgets.QGesture state, io.qt.core.QObject watched, io.qt.core.QEvent event){
return new io.qt.widgets.QGestureRecognizer.Result(recognize_native_QGesture_ptr_QObject_ptr_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(state), QtJambi_LibraryUtilities.internal.checkedNativeId(watched), QtJambi_LibraryUtilities.internal.checkedNativeId(event)));
}
@io.qt.QtUninvokable
private native int recognize_native_QGesture_ptr_QObject_ptr_QEvent_ptr(long __this__nativeId, long state, long watched, long event);
}
/**
* Java wrapper for Qt enum QGestureRecognizer::ResultFlag
*
* @see Result
*/
public enum ResultFlag implements io.qt.QtFlagEnumerator {
Ignore(1),
MayBeGesture(2),
TriggerGesture(4),
FinishGesture(8),
CancelGesture(16),
ResultState_Mask(255),
ConsumeEventHint(256),
ResultHint_Mask(65280);
private ResultFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public Result asFlags() {
return new Result(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public Result combined(ResultFlag e) {
return new Result(this, e);
}
/**
* Creates a new {@link Result} from the entries.
* @param values entries
* @return new flag
*/
public static Result flags(ResultFlag ... values) {
return new Result(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ResultFlag resolve(int value) {
switch (value) {
case 1: return Ignore;
case 2: return MayBeGesture;
case 4: return TriggerGesture;
case 8: return FinishGesture;
case 16: return CancelGesture;
case 255: return ResultState_Mask;
case 256: return ConsumeEventHint;
case 65280: return ResultHint_Mask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ResultFlag}
*/
public static final class Result extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x60dbc384dfadb9b1L;
/**
* Creates a new Result where the flags in args
are set.
* @param args enum entries
*/
public Result(ResultFlag ... args){
super(args);
}
/**
* Creates a new Result with given value
.
* @param value
*/
public Result(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new Result
*/
@Override
public final Result combined(ResultFlag e){
return new Result(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final Result setFlag(ResultFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final Result setFlag(ResultFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this Result.
* @return array of enum entries
*/
@Override
public final ResultFlag[] flags(){
return super.flags(ResultFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final Result clone(){
return new Result(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(Result other){
return Integer.compare(value(), other.value());
}
}
/**
* See QGestureRecognizer::QGestureRecognizer()
*/
public QGestureRecognizer(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QGestureRecognizer instance);
/**
* See QGestureRecognizer::create(QObject*)
*/
@io.qt.QtUninvokable
public io.qt.widgets.QGesture create(io.qt.core.QObject target){
return create_native_QObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(target));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QGesture create_native_QObject_ptr(long __this__nativeId, long target);
/**
* See QGestureRecognizer::recognize(QGesture*,QObject*,QEvent*)
*/
@io.qt.QtUninvokable
public abstract io.qt.widgets.QGestureRecognizer.Result recognize(io.qt.widgets.QGesture state, io.qt.core.QObject watched, io.qt.core.QEvent event);
@io.qt.QtUninvokable
private native int recognize_native_QGesture_ptr_QObject_ptr_QEvent_ptr(long __this__nativeId, long state, long watched, long event);
/**
* See QGestureRecognizer::reset(QGesture*)
*/
@io.qt.QtUninvokable
public void reset(io.qt.widgets.QGesture state){
reset_native_QGesture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(state));
}
@io.qt.QtUninvokable
private native void reset_native_QGesture_ptr(long __this__nativeId, long state);
/**
* See QGestureRecognizer::registerRecognizer(QGestureRecognizer*)
*/
public static io.qt.core.Qt.GestureType registerRecognizer(io.qt.widgets.QGestureRecognizer recognizer){
io.qt.core.Qt.GestureType __qt_return_value = io.qt.core.Qt.GestureType.resolve(registerRecognizer_native_QGestureRecognizer_ptr(QtJambi_LibraryUtilities.internal.checkedNativeId(recognizer)));
QtJambi_LibraryUtilities.internal.setCppOwnership(recognizer);
return __qt_return_value;
}
private native static int registerRecognizer_native_QGestureRecognizer_ptr(long recognizer);
/**
* See QGestureRecognizer::unregisterRecognizer(Qt::GestureType)
*/
public static void unregisterRecognizer(io.qt.core.Qt.GestureType type){
unregisterRecognizer_native_Qt_GestureType(type.value());
}
private native static void unregisterRecognizer_native_Qt_GestureType(int type);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QGestureRecognizer(QPrivateConstructor p) { super(p); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy