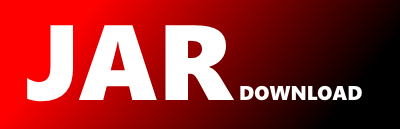
io.qt.widgets.QGraphicsColorizeEffect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* Colorize effect
* Java wrapper for Qt class QGraphicsColorizeEffect
*/
public class QGraphicsColorizeEffect extends io.qt.widgets.QGraphicsEffect
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QGraphicsColorizeEffect.class);
/**
* See QGraphicsColorizeEffect::colorChanged(QColor)
*/
@io.qt.QtPropertyNotify(name="color")
public final Signal1 colorChanged = new Signal1<>();
/**
* See QGraphicsColorizeEffect::strengthChanged(qreal)
*/
@io.qt.QtPropertyNotify(name="strength")
public final Signal1<@io.qt.QtPrimitiveType Double> strengthChanged = new Signal1<>();
/**
* Overloaded constructor for {@link #QGraphicsColorizeEffect(io.qt.core.QObject)}
* with parent = null
.
*/
public QGraphicsColorizeEffect() {
this((io.qt.core.QObject)null);
}
/**
* See QGraphicsColorizeEffect::QGraphicsColorizeEffect(QObject*)
*/
public QGraphicsColorizeEffect(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QGraphicsColorizeEffect instance, io.qt.core.QObject parent);
/**
* See QGraphicsColorizeEffect::color()const
*/
@io.qt.QtPropertyReader(name="color")
@io.qt.QtUninvokable
public final io.qt.gui.QColor color(){
return color_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QColor color_native_constfct(long __this__nativeId);
/**
* See QGraphicsColorizeEffect::setColor(QColor)
*/
@io.qt.QtPropertyWriter(name="color")
public final void setColor(io.qt.gui.QColor c){
setColor_native_cref_QColor(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(c));
}
private native void setColor_native_cref_QColor(long __this__nativeId, long c);
/**
* See QGraphicsColorizeEffect::setStrength(qreal)
*/
@io.qt.QtPropertyWriter(name="strength")
public final void setStrength(double strength){
setStrength_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), strength);
}
private native void setStrength_native_qtjambireal(long __this__nativeId, double strength);
/**
* See QGraphicsColorizeEffect::strength()const
*/
@io.qt.QtPropertyReader(name="strength")
@io.qt.QtUninvokable
public final double strength(){
return strength_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double strength_native_constfct(long __this__nativeId);
/**
* See QGraphicsEffect::draw(QPainter*)
*/
@io.qt.QtUninvokable
protected void draw(io.qt.gui.QPainter painter){
draw_native_QPainter_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(painter));
}
@io.qt.QtUninvokable
private native void draw_native_QPainter_ptr(long __this__nativeId, long painter);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QGraphicsColorizeEffect(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QGraphicsColorizeEffect(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QGraphicsColorizeEffect instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy