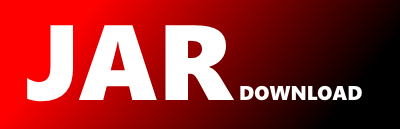
io.qt.widgets.QStyleOptionToolBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* Used to describe the parameters needed for drawing a tool box
* Java wrapper for Qt class QStyleOptionToolBox
*/
public class QStyleOptionToolBox extends io.qt.widgets.QStyleOption
implements java.lang.Cloneable
{
/**
* Java wrapper for Qt enum QStyleOptionToolBox::SelectedPosition
*/
public enum SelectedPosition implements io.qt.QtEnumerator {
NotAdjacent(0),
NextIsSelected(1),
PreviousIsSelected(2);
private SelectedPosition(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SelectedPosition resolve(int value) {
switch (value) {
case 0: return NotAdjacent;
case 1: return NextIsSelected;
case 2: return PreviousIsSelected;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QStyleOptionToolBox::TabPosition
*/
public enum TabPosition implements io.qt.QtEnumerator {
Beginning(0),
Middle(1),
End(2),
OnlyOneTab(3);
private TabPosition(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TabPosition resolve(int value) {
switch (value) {
case 0: return Beginning;
case 1: return Middle;
case 2: return End;
case 3: return OnlyOneTab;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QStyleOptionToolBox::QStyleOptionToolBox()
*/
public QStyleOptionToolBox(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QStyleOptionToolBox instance);
/**
* See QStyleOptionToolBox::QStyleOptionToolBox(QStyleOptionToolBox)
*/
public QStyleOptionToolBox(io.qt.widgets.QStyleOptionToolBox other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QStyleOptionToolBox instance, io.qt.widgets.QStyleOptionToolBox other);
@io.qt.QtUninvokable
public final void set(io.qt.widgets.QStyleOptionToolBox arg__1){
set_native_cref_QStyleOptionToolBox(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void set_native_cref_QStyleOptionToolBox(long __this__nativeId, long arg__1);
/**
* The icon for the tool box tab
*
*/
@io.qt.QtUninvokable
public final void setIcon(io.qt.gui.QIcon icon){
setIcon_native_cref_QIcon(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(icon));
}
@io.qt.QtUninvokable
private native void setIcon_native_cref_QIcon(long __this__nativeId, long icon);
/**
* The icon for the tool box tab
*
*/
@io.qt.QtUninvokable
public final io.qt.gui.QIcon icon(){
return icon_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QIcon icon_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void setPosition(io.qt.widgets.QStyleOptionToolBox.TabPosition position){
setPosition_native_cref_QStyleOptionToolBox_TabPosition(QtJambi_LibraryUtilities.internal.nativeId(this), position.value());
}
@io.qt.QtUninvokable
private native void setPosition_native_cref_QStyleOptionToolBox_TabPosition(long __this__nativeId, int position);
@io.qt.QtUninvokable
public final io.qt.widgets.QStyleOptionToolBox.TabPosition position(){
return io.qt.widgets.QStyleOptionToolBox.TabPosition.resolve(position_native(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int position_native(long __this__nativeId);
/**
* The position of the selected tab in relation to this tab
* See QStyleOptionToolBox::selectedPosition
*/
@io.qt.QtUninvokable
public final void setSelectedPosition(io.qt.widgets.QStyleOptionToolBox.SelectedPosition selectedPosition){
setSelectedPosition_native_cref_QStyleOptionToolBox_SelectedPosition(QtJambi_LibraryUtilities.internal.nativeId(this), selectedPosition.value());
}
@io.qt.QtUninvokable
private native void setSelectedPosition_native_cref_QStyleOptionToolBox_SelectedPosition(long __this__nativeId, int selectedPosition);
/**
* The position of the selected tab in relation to this tab
* See QStyleOptionToolBox::selectedPosition
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QStyleOptionToolBox.SelectedPosition selectedPosition(){
return io.qt.widgets.QStyleOptionToolBox.SelectedPosition.resolve(selectedPosition_native(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int selectedPosition_native(long __this__nativeId);
/**
* The text for the tool box tab
*
*/
@io.qt.QtUninvokable
public final void setText(java.lang.String text){
setText_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), text);
}
@io.qt.QtUninvokable
private native void setText_native_cref_QString(long __this__nativeId, java.lang.String text);
/**
* The text for the tool box tab
*
*/
@io.qt.QtUninvokable
public final java.lang.String text(){
return text_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String text_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QStyleOptionToolBox(QPrivateConstructor p) { super(p); }
@Override
public QStyleOptionToolBox clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QStyleOptionToolBox clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy