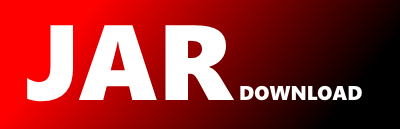
io.qt.widgets.QSwipeGesture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* Describes a swipe gesture made by the user
* Java wrapper for Qt class QSwipeGesture
*/
public class QSwipeGesture extends io.qt.widgets.QGesture
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QSwipeGesture.class);
/**
* Java wrapper for Qt enum QSwipeGesture::SwipeDirection
*/
public enum SwipeDirection implements io.qt.QtEnumerator {
NoDirection(0),
Left(1),
Right(2),
Up(3),
Down(4);
private SwipeDirection(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SwipeDirection resolve(int value) {
switch (value) {
case 0: return NoDirection;
case 1: return Left;
case 2: return Right;
case 3: return Up;
case 4: return Down;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Overloaded constructor for {@link #QSwipeGesture(io.qt.core.QObject)}
* with parent = null
.
*/
public QSwipeGesture() {
this((io.qt.core.QObject)null);
}
public QSwipeGesture(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QSwipeGesture instance, io.qt.core.QObject parent);
/**
* See QSwipeGesture::horizontalDirection()const
*/
@io.qt.QtPropertyReader(name="horizontalDirection")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final io.qt.widgets.QSwipeGesture.SwipeDirection horizontalDirection(){
return io.qt.widgets.QSwipeGesture.SwipeDirection.resolve(horizontalDirection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int horizontalDirection_native_constfct(long __this__nativeId);
/**
* See QSwipeGesture::setSwipeAngle(qreal)
*/
@io.qt.QtPropertyWriter(name="swipeAngle")
@io.qt.QtUninvokable
public final void setSwipeAngle(double value){
setSwipeAngle_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), value);
}
@io.qt.QtUninvokable
private native void setSwipeAngle_native_qtjambireal(long __this__nativeId, double value);
/**
* See QSwipeGesture::swipeAngle()const
*/
@io.qt.QtPropertyReader(name="swipeAngle")
@io.qt.QtUninvokable
public final double swipeAngle(){
return swipeAngle_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double swipeAngle_native_constfct(long __this__nativeId);
/**
* See QSwipeGesture::verticalDirection()const
*/
@io.qt.QtPropertyReader(name="verticalDirection")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final io.qt.widgets.QSwipeGesture.SwipeDirection verticalDirection(){
return io.qt.widgets.QSwipeGesture.SwipeDirection.resolve(verticalDirection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int verticalDirection_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QSwipeGesture(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QSwipeGesture(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QSwipeGesture instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy