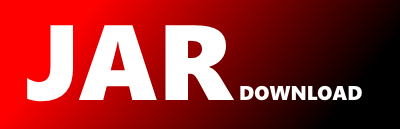
io.qt.widgets.QGestureEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.widgets;
/**
* The description of triggered gestures
* Java wrapper for Qt class QGestureEvent
*/
public class QGestureEvent extends io.qt.core.QEvent
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.QtPropertyMember(enabled=false)
private Object __rcGesture = null;
@io.qt.QtPropertyMember(enabled=false)
private Object __rcWidget = null;
/**
* See QGestureEvent::QGestureEvent(QList<QGesture*>)
*/
public QGestureEvent(java.util.Collection extends io.qt.widgets.QGesture> gestures){
super((QPrivateConstructor)null);
initialize_native(this, gestures);
}
private native static void initialize_native(QGestureEvent instance, java.util.Collection extends io.qt.widgets.QGesture> gestures);
/**
* See QGestureEvent::accept(QGesture*)
*/
@io.qt.QtUninvokable
public final void accept(io.qt.widgets.QGesture arg__1){
accept_native_QGesture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void accept_native_QGesture_ptr(long __this__nativeId, long arg__1);
/**
* See QGestureEvent::accept(Qt::GestureType)
*/
@io.qt.QtUninvokable
public final void accept(io.qt.core.Qt.GestureType arg__1){
accept_native_Qt_GestureType(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void accept_native_Qt_GestureType(long __this__nativeId, int arg__1);
/**
* See QGestureEvent::activeGestures()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList activeGestures(){
return activeGestures_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList activeGestures_native_constfct(long __this__nativeId);
/**
* See QGestureEvent::canceledGestures()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList canceledGestures(){
return canceledGestures_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList canceledGestures_native_constfct(long __this__nativeId);
/**
* See QGestureEvent::gesture(Qt::GestureType)const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QGesture gesture(io.qt.core.Qt.GestureType type){
return gesture_native_Qt_GestureType_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), type.value());
}
@io.qt.QtUninvokable
private native io.qt.widgets.QGesture gesture_native_Qt_GestureType_constfct(long __this__nativeId, int type);
/**
* See QGestureEvent::gestures()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList gestures(){
return gestures_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList gestures_native_constfct(long __this__nativeId);
/**
* See QGestureEvent::ignore(QGesture*)
*/
@io.qt.QtUninvokable
public final void ignore(io.qt.widgets.QGesture arg__1){
ignore_native_QGesture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void ignore_native_QGesture_ptr(long __this__nativeId, long arg__1);
/**
* See QGestureEvent::ignore(Qt::GestureType)
*/
@io.qt.QtUninvokable
public final void ignore(io.qt.core.Qt.GestureType arg__1){
ignore_native_Qt_GestureType(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void ignore_native_Qt_GestureType(long __this__nativeId, int arg__1);
/**
* See QGestureEvent::isAccepted(QGesture*)const
*/
@io.qt.QtUninvokable
public final boolean isAccepted(io.qt.widgets.QGesture arg__1){
return isAccepted_native_QGesture_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native boolean isAccepted_native_QGesture_ptr_constfct(long __this__nativeId, long arg__1);
/**
* See QGestureEvent::isAccepted(Qt::GestureType)const
*/
@io.qt.QtUninvokable
public final boolean isAccepted(io.qt.core.Qt.GestureType arg__1){
return isAccepted_native_Qt_GestureType_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native boolean isAccepted_native_Qt_GestureType_constfct(long __this__nativeId, int arg__1);
/**
* See QGestureEvent::mapToGraphicsScene(QPointF)const
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF mapToGraphicsScene(io.qt.core.QPointF gesturePoint){
return mapToGraphicsScene_native_cref_QPointF_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(gesturePoint));
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF mapToGraphicsScene_native_cref_QPointF_constfct(long __this__nativeId, long gesturePoint);
/**
* See QGestureEvent::setAccepted(QGesture*,bool)
*/
@io.qt.QtUninvokable
public final void setAccepted(io.qt.widgets.QGesture arg__1, boolean arg__2){
setAccepted_native_QGesture_ptr_bool(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1), arg__2);
__rcGesture = arg__1;
}
@io.qt.QtUninvokable
private native void setAccepted_native_QGesture_ptr_bool(long __this__nativeId, long arg__1, boolean arg__2);
/**
* See QGestureEvent::setAccepted(Qt::GestureType,bool)
*/
@io.qt.QtUninvokable
public final void setAccepted(io.qt.core.Qt.GestureType arg__1, boolean arg__2){
setAccepted_native_Qt_GestureType_bool(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value(), arg__2);
}
@io.qt.QtUninvokable
private native void setAccepted_native_Qt_GestureType_bool(long __this__nativeId, int arg__1, boolean arg__2);
@io.qt.QtUninvokable
public final void setWidget(io.qt.widgets.QWidget widget){
setWidget_native_QWidget_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(widget));
__rcWidget = widget;
}
@io.qt.QtUninvokable
private native void setWidget_native_QWidget_ptr(long __this__nativeId, long widget);
/**
* See QGestureEvent::widget()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QWidget widget(){
return widget_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QWidget widget_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QGestureEvent(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public String toString() {
return toString_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private static native String toString_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy