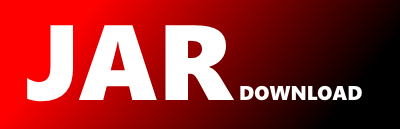
io.qt.core.QAbstractEventDispatcherV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
import io.qt.*;
/**
* Java wrapper for Qt class QAbstractEventDispatcherV2
*/
public abstract class QAbstractEventDispatcherV2 extends io.qt.core.QAbstractEventDispatcher
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QAbstractEventDispatcherV2.class);
@NativeAccess
private static final class ConcreteWrapper extends QAbstractEventDispatcherV2 {
@NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@QtUninvokable
public void interrupt(){
interrupt_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void interrupt_native(long __this__nativeId);
@Override
@QtUninvokable
public boolean processEvents(io.qt.core.QEventLoop.@NonNull ProcessEventsFlags flags){
return processEvents_native_QEventLoop_ProcessEventsFlags(QtJambi_LibraryUtilities.internal.nativeId(this), flags.value());
}
@QtUninvokable
private native boolean processEvents_native_QEventLoop_ProcessEventsFlags(long __this__nativeId, int flags);
@Override
@QtUninvokable
public void registerSocketNotifier(io.qt.core.@StrictNonNull QSocketNotifier notifier){
java.util.Objects.requireNonNull(notifier, "Argument 'notifier': null not expected.");
InternalAccess.NativeIdInfo __notifier__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(notifier);
registerSocketNotifier_native_QSocketNotifier_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __notifier__NativeIdInfo.nativeId());
if (__notifier__NativeIdInfo.needsReferenceCounting()) {
QtJambi_LibraryUtilities.internal.addReferenceCount(this, io.qt.core.QAbstractEventDispatcher.class, "__rcSocketNotifier", false, false, notifier);
}
}
@QtUninvokable
private native void registerSocketNotifier_native_QSocketNotifier_ptr(long __this__nativeId, long notifier);
@Override
@QtUninvokable
public void registerTimer(io.qt.core.Qt.@NonNull TimerId timerId, java.time.temporal.@NonNull TemporalAmount interval, io.qt.core.Qt.@NonNull TimerType timerType, io.qt.core.@Nullable QObject object){
registerTimer_native_Qt_TimerId_std_chrono_nanoseconds_Qt_TimerType_QObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), timerId.value(), interval, timerType.value(), QtJambi_LibraryUtilities.internal.checkedNativeId(object));
}
@QtUninvokable
private native void registerTimer_native_Qt_TimerId_std_chrono_nanoseconds_Qt_TimerType_QObject_ptr(long __this__nativeId, int timerId, java.time.temporal.TemporalAmount interval, int timerType, long object);
@Override
@QtUninvokable
public java.time.temporal.@NonNull TemporalAmount remainingTime(io.qt.core.Qt.@NonNull TimerId timerId){
return remainingTime_native_Qt_TimerId_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), timerId.value());
}
@QtUninvokable
private native java.time.temporal.@NonNull TemporalAmount remainingTime_native_Qt_TimerId_constfct(long __this__nativeId, int timerId);
@Override
@QtUninvokable
public java.util.@NonNull List timersForObject(io.qt.core.@Nullable QObject object){
return timersForObject_native_QObject_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(object));
}
@QtUninvokable
private native java.util.@NonNull List timersForObject_native_QObject_ptr_constfct(long __this__nativeId, long object);
@Override
@QtUninvokable
public void unregisterSocketNotifier(io.qt.core.@StrictNonNull QSocketNotifier notifier){
java.util.Objects.requireNonNull(notifier, "Argument 'notifier': null not expected.");
InternalAccess.NativeIdInfo __notifier__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(notifier);
unregisterSocketNotifier_native_QSocketNotifier_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __notifier__NativeIdInfo.nativeId());
QtJambi_LibraryUtilities.internal.removeFromCollectionReferenceCount(this, io.qt.core.QAbstractEventDispatcher.class, "__rcSocketNotifier", false, notifier);
}
@QtUninvokable
private native void unregisterSocketNotifier_native_QSocketNotifier_ptr(long __this__nativeId, long notifier);
@Override
@QtUninvokable
public boolean unregisterTimer(io.qt.core.Qt.@NonNull TimerId timerId){
return unregisterTimer_native_Qt_TimerId(QtJambi_LibraryUtilities.internal.nativeId(this), timerId.value());
}
@QtUninvokable
private native boolean unregisterTimer_native_Qt_TimerId(long __this__nativeId, int timerId);
@Override
@QtUninvokable
public boolean unregisterTimers(io.qt.core.@Nullable QObject object){
return unregisterTimers_native_QObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(object));
}
@QtUninvokable
private native boolean unregisterTimers_native_QObject_ptr(long __this__nativeId, long object);
@Override
@QtUninvokable
public void wakeUp(){
wakeUp_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void wakeUp_native(long __this__nativeId);
}
/**
* See QAbstractEventDispatcherV2:: QAbstractEventDispatcherV2(QObject*)
* @param parent
*/
public QAbstractEventDispatcherV2(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QAbstractEventDispatcherV2 instance, io.qt.core.QObject parent);
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
public final void registerTimer(int timerId, long interval, io.qt.core.Qt.@NonNull TimerType timerType, io.qt.core.@Nullable QObject object) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
public final io.qt.core.@NonNull QList registeredTimers(io.qt.core.@Nullable QObject object) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
public final int remainingTime(int timerId) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
public final boolean unregisterTimer(int timerId) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* See QAbstractEventDispatcherV2:: processEventsWithDeadline(QEventLoop::ProcessEventsFlags, QDeadlineTimer)
* @param flags
* @param deadline
* @return
*/
@QtUninvokable
public boolean processEventsWithDeadline(io.qt.core.QEventLoop.@NonNull ProcessEventsFlags flags, io.qt.core.@NonNull QDeadlineTimer deadline){
return processEventsWithDeadline_native_QEventLoop_ProcessEventsFlags_QDeadlineTimer(QtJambi_LibraryUtilities.internal.nativeId(this), flags.value(), QtJambi_LibraryUtilities.internal.checkedNativeId(deadline));
}
@QtUninvokable
private native boolean processEventsWithDeadline_native_QEventLoop_ProcessEventsFlags_QDeadlineTimer(long __this__nativeId, int flags, long deadline);
/**
*
* @since This function was introduced in Qt 6.8.
* @param timerId
* @param interval
* @param timerType
* @param object
*/
@QtUninvokable
public abstract void registerTimer(io.qt.core.Qt.@NonNull TimerId timerId, java.time.temporal.@NonNull TemporalAmount interval, io.qt.core.Qt.@NonNull TimerType timerType, io.qt.core.@Nullable QObject object);
@QtUninvokable
private native void registerTimer_native_Qt_TimerId_std_chrono_nanoseconds_Qt_TimerType_QObject_ptr(long __this__nativeId, int timerId, java.time.temporal.TemporalAmount interval, int timerType, long object);
/**
* See QAbstractEventDispatcher:: remainingTime(Qt::TimerId)const
* @param timerId
* @return
*/
@QtUninvokable
public abstract java.time.temporal.@NonNull TemporalAmount remainingTime(io.qt.core.Qt.@NonNull TimerId timerId);
@QtUninvokable
private native java.time.temporal.@NonNull TemporalAmount remainingTime_native_Qt_TimerId_constfct(long __this__nativeId, int timerId);
/**
* See QAbstractEventDispatcher:: timersForObject(QObject*)const
* @since This function was introduced in Qt 6.8.
* @param object
* @return
*/
@QtUninvokable
public abstract java.util.@NonNull List timersForObject(io.qt.core.@Nullable QObject object);
@QtUninvokable
private native java.util.@NonNull List timersForObject_native_QObject_ptr_constfct(long __this__nativeId, long object);
/**
* See QAbstractEventDispatcher:: unregisterTimer(Qt::TimerId)
* @since This function was introduced in Qt 6.8.
* @param timerId
* @return
*/
@QtUninvokable
public abstract boolean unregisterTimer(io.qt.core.Qt.@NonNull TimerId timerId);
@QtUninvokable
private native boolean unregisterTimer_native_Qt_TimerId(long __this__nativeId, int timerId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QAbstractEventDispatcherV2(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QAbstractEventDispatcherV2(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QAbstractEventDispatcherV2 instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QAbstractEventDispatcherV2(io.qt.core.QObject)}
* with parent = null
.
*/
public QAbstractEventDispatcherV2() {
this((io.qt.core.QObject)null);
}
/**
* Overloaded function for {@link #processEventsWithDeadline(io.qt.core.QEventLoop.ProcessEventsFlags, io.qt.core.QDeadlineTimer)}.
*/
@QtUninvokable
public final boolean processEventsWithDeadline(io.qt.core.QEventLoop.@NonNull ProcessEventsFlags flags, io.qt.core.QDeadlineTimer.@NonNull ForeverConstant deadline) {
return processEventsWithDeadline(flags, new io.qt.core.QDeadlineTimer(deadline));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy