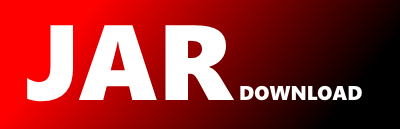
io.qt.core.QAssociativeConstIterator Maven / Gradle / Ivy
/****************************************************************************
**
** Copyright (C) 2009-2024 Dr. Peter Droste, Omix Visualization GmbH & Co. KG. All rights reserved.
**
** This file is part of Qt Jambi.
**
** $BEGIN_LICENSE$
** GNU Lesser General Public License Usage
** This file may be used under the terms of the GNU Lesser
** General Public License version 2.1 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 2.1 requirements
** will be met: http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 3.0 as published by the Free Software
** Foundation and appearing in the file LICENSE.GPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU General Public License version 3.0 requirements will be
** met: http://www.gnu.org/copyleft/gpl.html.
** $END_LICENSE$
**
** This file is provided AS IS with NO WARRANTY OF ANY KIND, INCLUDING THE
** WARRANTY OF DESIGN, MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE.
**
****************************************************************************/
package io.qt.core;
import java.util.ConcurrentModificationException;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.function.Function;
import io.qt.NativeAccess;
import io.qt.QtObject;
import io.qt.QtUninvokable;
/**
* Java-iterable wrapper for Qt's constant iterator types:
*
* - QMap<K,V>::const_iterator
* - QHash<K,V>::const_iterator
* - QMultiMap<K,V>::const_iterator
* - QMultiHash<K,V>::const_iterator
*
* @param key type
* @param value type
* @see QMap#constBegin()
* @see QMap#constEnd()
* @see QMap#find(Object)
* @see QMap#lowerBound(Object)
* @see QMap#upperBound(Object)
* @see QHash#constBegin()
* @see QHash#constEnd()
* @see QHash#find(Object)
* @see QMultiMap#constBegin()
* @see QMultiMap#constEnd()
* @see QMultiMap#find(Object)
* @see QMultiMap#find(Object, Object)
* @see QMultiMap#lowerBound(Object)
* @see QMultiMap#upperBound(Object)
* @see QMultiHash#constBegin()
* @see QMultiHash#constEnd()
* @see QMultiHash#find(Object)
* @see #iterator()
*/
public class QAssociativeConstIterator extends AbstractIterator implements java.lang.Iterable> {
static {
QtJambi_LibraryUtilities.initialize();
}
@NativeAccess
QAssociativeConstIterator(QPrivateConstructor c, QtObject owner) {
super(c, owner);
}
/**
* Returns the current item's key.
*/
@QtUninvokable
final Key _key() {
return key(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private static native K key(long __this__nativeId);
@QtUninvokable
static native QMetaType keyType(long __this__nativeId);
/**
* {@inheritDoc}
*/
@Override
@QtUninvokable
public boolean equals(Object other) {
if (other instanceof QAssociativeConstIterator) {
return super.equals(other);
}
return false;
}
private final static Function, java.util.Iterator>> iteratorFactory;
static {
if(Boolean.getBoolean("io.qt.enable-concurrent-container-modification-check")) {
@SuppressWarnings("unchecked")
Function, java.util.Iterator>> _iteratorFactory = CheckingIncrementalIterator::new;
iteratorFactory = _iteratorFactory;
}else {
@SuppressWarnings("unchecked")
Function, java.util.Iterator>> _iteratorFactory = IncrementalIterator::new;
iteratorFactory = _iteratorFactory;
}
}
private static class IncrementalIterator implements java.util.Iterator>{
final QAssociativeConstIterator nativeIterator;
final AbstractIterator end;
private boolean hasNext;
IncrementalIterator(QAssociativeConstIterator nativeIterator) {
super();
this.nativeIterator = nativeIterator;
end = nativeIterator.end();
hasNext = end!=null && !nativeIterator.equals(end);
}
@Override
public boolean hasNext() {
return hasNext;
}
@Override
public QPair next() {
checkNext();
QPair e = new QPair<>(nativeIterator._key(), nativeIterator._value());
nativeIterator.increment();
hasNext = end!=null && !nativeIterator.equals(end);
return e;
}
void checkNext() {
if(!hasNext)
throw new NoSuchElementException();
}
}
private static class CheckingIncrementalIterator extends IncrementalIterator{
CheckingIncrementalIterator(QAssociativeConstIterator nativeIterator) {
super(nativeIterator);
}
void checkNext() {
super.checkNext();
if(end!=null && !end.equals(nativeIterator.end()))
throw new ConcurrentModificationException();
}
}
/**
* Returns a Java iterator between this and the container's end.
*/
@SuppressWarnings("unchecked")
@QtUninvokable
public final Iterator> iterator(){
return (java.util.Iterator>)iteratorFactory.apply(this);
}
/**
* Returns the key value pair at iterator's position in the container or emptiness in case of end
.
*/
@QtUninvokable
public final Optional> keyValuePair() {
return !isValid() ? Optional.empty() : Optional.ofNullable(new QPair<>(_key(), _value()));
}
/**
* Returns the key at iterator's position in the container or emptiness in case of end
.
*/
@QtUninvokable
public final Optional key() {
return !isValid() ? Optional.empty() : Optional.ofNullable(_key());
}
/**
* Returns the key type of the iterator.
*/
@QtUninvokable
public final QMetaType keyType() {
return keyType(QtJambi_LibraryUtilities.internal.nativeId(this));
}
/**
* Returns the current item's key if item is valid
* or throws NoSuchElementException otherwise.
*/
@QtUninvokable
final Key checkedKey() throws NoSuchElementException {
if(isValid()) {
return _key();
}else {
throw new NoSuchElementException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy