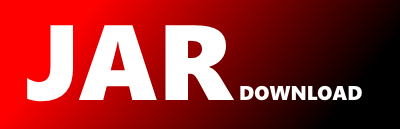
io.qt.core.QSharedMemory Maven / Gradle / Ivy
package io.qt.core;
import io.qt.*;
/**
* Access to a shared memory segment
* Java wrapper for Qt class QSharedMemory
*/
public class QSharedMemory extends io.qt.core.QObject
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QSharedMemory.class);
/**
* Java wrapper for Qt enum QSharedMemory::AccessMode
*/
public enum AccessMode implements QtEnumerator {
/**
* Representing QSharedMemory:: ReadOnly
*/
ReadOnly(0),
/**
* Representing QSharedMemory:: ReadWrite
*/
ReadWrite(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private AccessMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull AccessMode resolve(int value) {
switch (value) {
case 0: return ReadOnly;
case 1: return ReadWrite;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QSharedMemory::SharedMemoryError
*/
public enum SharedMemoryError implements QtEnumerator {
/**
* Representing QSharedMemory:: NoError
*/
NoError(0),
/**
* Representing QSharedMemory:: PermissionDenied
*/
PermissionDenied(1),
/**
* Representing QSharedMemory:: InvalidSize
*/
InvalidSize(2),
/**
* Representing QSharedMemory:: KeyError
*/
KeyError(3),
/**
* Representing QSharedMemory:: AlreadyExists
*/
AlreadyExists(4),
/**
* Representing QSharedMemory:: NotFound
*/
NotFound(5),
/**
* Representing QSharedMemory:: LockError
*/
LockError(6),
/**
* Representing QSharedMemory:: OutOfResources
*/
OutOfResources(7),
/**
* Representing QSharedMemory:: UnknownError
*/
UnknownError(8);
static {
QtJambi_LibraryUtilities.initialize();
}
private SharedMemoryError(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull SharedMemoryError resolve(int value) {
switch (value) {
case 0: return NoError;
case 1: return PermissionDenied;
case 2: return InvalidSize;
case 3: return KeyError;
case 4: return AlreadyExists;
case 5: return NotFound;
case 6: return LockError;
case 7: return OutOfResources;
case 8: return UnknownError;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QSharedMemory:: QSharedMemory(QNativeIpcKey, QObject*)
* @param key
* @param parent
*/
public QSharedMemory(io.qt.core.@StrictNonNull QNativeIpcKey key, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
java.util.Objects.requireNonNull(key, "Argument 'key': null not expected.");
initialize_native(this, key, parent);
}
private native static void initialize_native(QSharedMemory instance, io.qt.core.QNativeIpcKey key, io.qt.core.QObject parent);
/**
* See QSharedMemory:: QSharedMemory(QObject*)
* @param parent
*/
public QSharedMemory(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QSharedMemory instance, io.qt.core.QObject parent);
/**
* See QSharedMemory:: QSharedMemory(QString, QObject*)
* @param key
* @param parent
*/
public QSharedMemory(java.lang.@NonNull String key, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, key, parent);
}
private native static void initialize_native(QSharedMemory instance, java.lang.String key, io.qt.core.QObject parent);
/**
* See QSharedMemory:: attach(QSharedMemory::AccessMode)
* @param mode
* @return
*/
@QtUninvokable
public final boolean attach(io.qt.core.QSharedMemory.@NonNull AccessMode mode){
boolean __qt_return_value = attach_native_QSharedMemory_AccessMode(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
if(__qt_return_value){
__qt_accessMode = mode;
}
return __qt_return_value;
}
@QtUninvokable
private native boolean attach_native_QSharedMemory_AccessMode(long __this__nativeId, int mode);
/**
* See QSharedMemory:: create(qsizetype, QSharedMemory::AccessMode)
* @param size
* @param mode
* @return
*/
@QtUninvokable
public final boolean create(long size, io.qt.core.QSharedMemory.@NonNull AccessMode mode){
boolean __qt_return_value = create_native_qsizetype_QSharedMemory_AccessMode(QtJambi_LibraryUtilities.internal.nativeId(this), size, mode.value());
if(__qt_return_value){
__qt_accessMode = mode;
}
return __qt_return_value;
}
@QtUninvokable
private native boolean create_native_qsizetype_QSharedMemory_AccessMode(long __this__nativeId, long size, int mode);
@QtUninvokable
private final java.nio.@Nullable Buffer data(){
return data_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.nio.Buffer data_native(long __this__nativeId);
/**
*
* @return
*/
@QtUninvokable
public final boolean detach(){
return detach_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean detach_native(long __this__nativeId);
/**
* See QSharedMemory:: error()const
* @return
*/
@QtUninvokable
public final io.qt.core.QSharedMemory.@NonNull SharedMemoryError error(){
return io.qt.core.QSharedMemory.SharedMemoryError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QSharedMemory:: errorString()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String errorString(){
return errorString_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String errorString_native_constfct(long __this__nativeId);
/**
* See QSharedMemory:: isAttached()const
* @return
*/
@QtUninvokable
public final boolean isAttached(){
return isAttached_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isAttached_native_constfct(long __this__nativeId);
/**
*
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String key(){
return key_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String key_native_constfct(long __this__nativeId);
@QtUninvokable
private final boolean lock(){
return lock_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean lock_native(long __this__nativeId);
/**
* See QSharedMemory:: nativeIpcKey()const
* @since This function was introduced in Qt 6.6.
* @return
*/
@QtUninvokable
public final io.qt.core.@NonNull QNativeIpcKey nativeIpcKey(){
return nativeIpcKey_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QNativeIpcKey nativeIpcKey_native_constfct(long __this__nativeId);
/**
* See QSharedMemory:: nativeKey()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String nativeKey(){
return nativeKey_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String nativeKey_native_constfct(long __this__nativeId);
/**
* See QSharedMemory:: setKey(QString)
* @param key
*/
@QtUninvokable
public final void setKey(java.lang.@NonNull String key){
setKey_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), key);
}
@QtUninvokable
private native void setKey_native_cref_QString(long __this__nativeId, java.lang.String key);
/**
* See QSharedMemory:: setNativeKey(QNativeIpcKey)
* @since This function was introduced in Qt 6.6.
* @param key
*/
@QtUninvokable
public final void setNativeKey(io.qt.core.@StrictNonNull QNativeIpcKey key){
java.util.Objects.requireNonNull(key, "Argument 'key': null not expected.");
setNativeKey_native_cref_QNativeIpcKey(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(key));
}
@QtUninvokable
private native void setNativeKey_native_cref_QNativeIpcKey(long __this__nativeId, long key);
/**
* See QSharedMemory:: setNativeKey(QString, QNativeIpcKey::Type)
* @param key
* @param type
*/
@QtUninvokable
public final void setNativeKey(java.lang.@NonNull String key, io.qt.core.QNativeIpcKey.@NonNull Type type){
setNativeKey_native_cref_QString_QNativeIpcKey_Type(QtJambi_LibraryUtilities.internal.nativeId(this), key, type.value());
}
@QtUninvokable
private native void setNativeKey_native_cref_QString_QNativeIpcKey_Type(long __this__nativeId, java.lang.String key, short type);
/**
* See QSharedMemory:: size()const
* @return
*/
@QtUninvokable
public final long size(){
return size_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long size_native_constfct(long __this__nativeId);
@QtUninvokable
private final boolean unlock(){
return unlock_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean unlock_native(long __this__nativeId);
/**
* See QSharedMemory:: isKeyTypeSupported(QNativeIpcKey::Type)
* @param type
* @return
*/
public static boolean isKeyTypeSupported(io.qt.core.QNativeIpcKey.@NonNull Type type){
return isKeyTypeSupported_native_QNativeIpcKey_Type(type.value());
}
private native static boolean isKeyTypeSupported_native_QNativeIpcKey_Type(short type);
/**
* See QSharedMemory:: legacyNativeKey(QString, QNativeIpcKey::Type)
* @param key
* @param type
* @return
*/
public static io.qt.core.@NonNull QNativeIpcKey legacyNativeKey(java.lang.@NonNull String key, io.qt.core.QNativeIpcKey.@NonNull Type type){
return legacyNativeKey_native_cref_QString_QNativeIpcKey_Type(key, type.value());
}
private native static io.qt.core.QNativeIpcKey legacyNativeKey_native_cref_QString_QNativeIpcKey_Type(java.lang.String key, short type);
/**
* See QSharedMemory:: platformSafeKey(QString, QNativeIpcKey::Type)
* @param key
* @param type
* @return
*/
public static io.qt.core.@NonNull QNativeIpcKey platformSafeKey(java.lang.@NonNull String key, io.qt.core.QNativeIpcKey.@NonNull Type type){
return platformSafeKey_native_cref_QString_QNativeIpcKey_Type(key, type.value());
}
private native static io.qt.core.QNativeIpcKey platformSafeKey_native_cref_QString_QNativeIpcKey_Type(java.lang.String key, short type);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QSharedMemory(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QSharedMemory(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QSharedMemory instance, QDeclarativeConstructor constructor);
private static class CleanTask implements Runnable{
private CleanTask(QSharedMemory sharedMemory) {
super();
this.sharedMemory = sharedMemory;
}
@Override
public void run() {
if(!isClosed) {
isClosed = true;
try{
if(!sharedMemory.isDisposed())
sharedMemory.unlock();
}finally {
sharedMemory.__qt_isInUse = false;
}
}
}
private final QSharedMemory sharedMemory;
private boolean isClosed = false;
}
/**
* This type represents a locked data access.
* Close this after accessing data. It is recommended to use this in resource try block.
* @see QSharedMemory#access()
*/
public static class DataAccess implements AutoCloseable{
private final QSharedMemory sharedMemory;
private final io.qt.InternalAccess.Cleanable cleanable;
DataAccess(QSharedMemory sharedMemory){
this.sharedMemory = sharedMemory;
cleanable = QtJambi_LibraryUtilities.internal.registerCleaner(this, new CleanTask(sharedMemory));
}
/**
* Unlocks QSharedMemory
*/
@Override
public void close(){
cleanable.clean();
}
/**
* Access to QSharedMemory's data
*/
@QtUninvokable
public java.nio.@Nullable ByteBuffer data() {
if(!sharedMemory.__qt_isInUse) {
throw new IllegalStateException();
}
java.nio.ByteBuffer result = (java.nio.ByteBuffer)sharedMemory.data();
if(result!=null && sharedMemory.__qt_accessMode==AccessMode.ReadOnly){
result = result.asReadOnlyBuffer();
}
return result;
}
}
private AccessMode __qt_accessMode = AccessMode.ReadOnly;
private boolean __qt_isInUse;
/**
* Locks QSharedMemory and gives access to its data.
*/
public @NonNull DataAccess access() throws IllegalStateException{
if(this.isAttached() && !__qt_isInUse && lock()) {
__qt_isInUse = true;
return new DataAccess(this);
}
throw new IllegalStateException();
}
/**
* Overloaded constructor for {@link #QSharedMemory(io.qt.core.QNativeIpcKey, io.qt.core.QObject)}
* with parent = null
.
*/
public QSharedMemory(io.qt.core.@StrictNonNull QNativeIpcKey key) {
this(key, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QSharedMemory(io.qt.core.QObject)}
* with parent = null
.
*/
public QSharedMemory() {
this((io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QSharedMemory(java.lang.String, io.qt.core.QObject)}
* with parent = null
.
*/
public QSharedMemory(java.lang.@NonNull String key) {
this(key, (io.qt.core.QObject)null);
}
/**
* Overloaded function for {@link #attach(io.qt.core.QSharedMemory.AccessMode)}
* with mode = io.qt.core.QSharedMemory.AccessMode.ReadWrite
.
*/
@QtUninvokable
public final boolean attach() {
return attach(io.qt.core.QSharedMemory.AccessMode.ReadWrite);
}
/**
* Overloaded function for {@link #create(long, io.qt.core.QSharedMemory.AccessMode)}
* with mode = io.qt.core.QSharedMemory.AccessMode.ReadWrite
.
*/
@QtUninvokable
public final boolean create(long size) {
return create(size, io.qt.core.QSharedMemory.AccessMode.ReadWrite);
}
/**
* Overloaded function for {@link #setNativeKey(java.lang.String, io.qt.core.QNativeIpcKey.Type)}
* with type = io.qt.core.QNativeIpcKey.legacyDefaultTypeForOs()
.
*/
@QtUninvokable
public final void setNativeKey(java.lang.@NonNull String key) {
setNativeKey(key, io.qt.core.QNativeIpcKey.legacyDefaultTypeForOs());
}
/**
* Overloaded function for {@link #legacyNativeKey(java.lang.String, io.qt.core.QNativeIpcKey.Type)}
* with type = io.qt.core.QNativeIpcKey.legacyDefaultTypeForOs()
.
*/
public static io.qt.core.@NonNull QNativeIpcKey legacyNativeKey(java.lang.@NonNull String key) {
return legacyNativeKey(key, io.qt.core.QNativeIpcKey.legacyDefaultTypeForOs());
}
/**
* Overloaded function for {@link #platformSafeKey(java.lang.String, io.qt.core.QNativeIpcKey.Type)}
* with type = io.qt.core.QNativeIpcKey.DefaultTypeForOs
.
*/
public static io.qt.core.@NonNull QNativeIpcKey platformSafeKey(java.lang.@NonNull String key) {
return platformSafeKey(key, io.qt.core.QNativeIpcKey.DefaultTypeForOs);
}
}