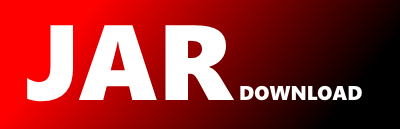
io.qt.core.internal.QAbstractFileEngineHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core.internal;
import io.qt.*;
/**
* Java wrapper for Qt class QAbstractFileEngineHandler
*/
public abstract class QAbstractFileEngineHandler extends QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
@NativeAccess
private static final class ConcreteWrapper extends QAbstractFileEngineHandler {
@NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@QtUninvokable
public io.qt.core.internal.@Nullable QAbstractFileEngine create(java.lang.@NonNull String fileName){
return create_native_cref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), fileName);
}
@QtUninvokable
private native io.qt.core.internal.QAbstractFileEngine create_native_cref_QString_constfct(long __this__nativeId, java.lang.String fileName);
}
/**
* See QAbstractFileEngineHandler:: QAbstractFileEngineHandler()
*/
public QAbstractFileEngineHandler(){
super((QPrivateConstructor)null);
QtJambi_LibraryUtilities.internal.setCppOwnership(this);
initialize_native(this);
}
private native static void initialize_native(QAbstractFileEngineHandler instance);
/**
* See QAbstractFileEngineHandler:: create(QString)const
* @param fileName
* @return
*/
@QtUninvokable
public abstract io.qt.core.internal.@Nullable QAbstractFileEngine create(java.lang.@NonNull String fileName);
@QtUninvokable
private native io.qt.core.internal.QAbstractFileEngine create_native_cref_QString_constfct(long __this__nativeId, java.lang.String fileName);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QAbstractFileEngineHandler(QPrivateConstructor p) { super(p); }
/**
* Factory interface for file engine creation
*/
@FunctionalInterface
public interface FileEngineFactory extends java.util.function.Function<@NonNull String, @Nullable QAbstractFileEngine>{
}
private static final java.util.Map factories = java.util.Collections.synchronizedMap(new java.util.TreeMap<>(Long::compareTo));
@QtUninvokable
private static void registerFactory(long id, FileEngineFactory factory){
factories.put(id, factory);
}
@QtUninvokable
private static void unregisterFactory(long id){
factories.remove(id);
}
@QtUninvokable
private static QAbstractFileEngine createByFactory(long id, java.lang.@NonNull String fileName){
FileEngineFactory factory = factories.get(id);
if(factory!=null)
return factory.apply(fileName);
else
return null;
}
/**
* Creates a file engine handler for file names starting with {@code prefix}
* @param factory the file engine factory
* @param prefix file name prefix
* @return a new installed file engine handler
* @see String#startsWith(String)
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromStartsWith(@StrictNonNull FileEngineFactory factory, @NonNull String prefix) {
java.util.Objects.requireNonNull(prefix, "Argument 'prefix': null not expected.");
java.util.Objects.requireNonNull(factory, "Argument 'factory': null not expected.");
if(prefix.isEmpty())
throw new IllegalArgumentException("Argument 'prefix': empty String not expected.");
return fromStartsWith(prefix, factory);
}
@QtUninvokable
private static native QAbstractFileEngineHandler fromStartsWith(String prefix, FileEngineFactory factory);
/**
* Creates a file engine handler for file names ending with {@code suffix}
* @param factory the file engine factory
* @param suffix file name suffix
* @return a new installed file engine handler
* @see String#endsWith(String)
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromEndsWith(@StrictNonNull FileEngineFactory factory, @NonNull String suffix) {
java.util.Objects.requireNonNull(suffix, "Argument 'suffix': null not expected.");
java.util.Objects.requireNonNull(factory, "Argument 'factory': null not expected.");
if(suffix.isEmpty())
throw new IllegalArgumentException("Argument 'suffix': empty String not expected.");
return fromEndsWith(suffix, factory);
}
@QtUninvokable
private static native QAbstractFileEngineHandler fromEndsWith(String suffix, FileEngineFactory factory);
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, io.qt.core.QRegularExpression.@NonNull MatchType matchType, io.qt.core.QRegularExpression.@NonNull MatchOptions matchOptions) {
return fromMatch(factory, regexp, 0, matchType, matchOptions);
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, io.qt.core.QRegularExpression.@NonNull MatchOptions matchOptions) {
return fromMatch(factory, regexp, 0, io.qt.core.QRegularExpression.MatchType.NormalMatch, matchOptions);
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param matchType the match type
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, io.qt.core.QRegularExpression.@NonNull MatchType matchType) {
return fromMatch(factory, regexp, 0, matchType, io.qt.core.QRegularExpression.MatchOption.NoMatchOption.asFlags());
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, io.qt.core.QRegularExpression.@NonNull MatchType matchType, io.qt.core.QRegularExpression.@NonNull MatchOption @NonNull... matchOptions) {
return fromMatch(factory, regexp, 0, matchType, new io.qt.core.QRegularExpression.MatchOptions(matchOptions));
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, io.qt.core.QRegularExpression.@NonNull MatchOption @NonNull... matchOptions) {
return fromMatch(factory, regexp, 0, io.qt.core.QRegularExpression.MatchType.NormalMatch, new io.qt.core.QRegularExpression.MatchOptions(matchOptions));
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp) {
return fromMatch(factory, regexp, 0, io.qt.core.QRegularExpression.MatchType.NormalMatch, io.qt.core.QRegularExpression.MatchOption.NoMatchOption.asFlags());
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset) {
return fromMatch(factory, regexp, offset, io.qt.core.QRegularExpression.MatchType.NormalMatch, io.qt.core.QRegularExpression.MatchOption.NoMatchOption.asFlags());
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset, io.qt.core.QRegularExpression.@NonNull MatchType matchType, io.qt.core.QRegularExpression.@NonNull MatchOption @NonNull... matchOptions) {
return fromMatch(factory, regexp, offset, matchType, new io.qt.core.QRegularExpression.MatchOptions(matchOptions));
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset, io.qt.core.QRegularExpression.@NonNull MatchType matchType) {
return fromMatch(factory, regexp, offset, matchType, io.qt.core.QRegularExpression.MatchOption.NoMatchOption.asFlags());
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset, io.qt.core.QRegularExpression.@NonNull MatchOption @NonNull... matchOptions) {
return fromMatch(factory, regexp, offset, io.qt.core.QRegularExpression.MatchType.NormalMatch, new io.qt.core.QRegularExpression.MatchOptions(matchOptions));
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset, io.qt.core.QRegularExpression.@NonNull MatchOptions matchOptions) {
return fromMatch(factory, regexp, offset, io.qt.core.QRegularExpression.MatchType.NormalMatch, matchOptions);
}
/**
* Creates a file engine handler for file names having match with {@code regexp}
* @param factory the file engine factory
* @param regexp file name regexp
* @param offset the offset of the match
* @param matchType the match type
* @param matchOptions options for the match
* @return a new installed file engine handler
* @see io.qt.core.QRegularExpression#match(String, long, io.qt.core.QRegularExpression.MatchType, io.qt.core.QRegularExpression.MatchOptions)
* @see io.qt.core.QRegularExpressionMatch#hasMatch()
*/
@QtUninvokable
public static QAbstractFileEngineHandler fromMatch(@StrictNonNull FileEngineFactory factory, io.qt.core.@NonNull QRegularExpression regexp, long offset, io.qt.core.QRegularExpression.@NonNull MatchType matchType, io.qt.core.QRegularExpression.@NonNull MatchOptions matchOptions) {
java.util.Objects.requireNonNull(regexp, "Argument 'regexp': null not expected.");
java.util.Objects.requireNonNull(factory, "Argument 'factory': null not expected.");
if(!regexp.isValid())
throw new IllegalArgumentException("Argument 'regexp' invalid");
if(offset<0)
throw new IllegalArgumentException("Argument 'offset' < 0");
return fromMatch(factory, QtJambi_LibraryUtilities.internal.checkedNativeId(regexp), offset, matchType==null ? 0 : matchType.value(), matchOptions==null ? 0 : matchOptions.value());
}
@QtUninvokable
private static native QAbstractFileEngineHandler fromMatch(FileEngineFactory factory, long regexp, long offset, int matchType, int matchOptions);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy