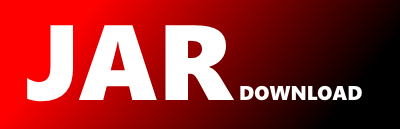
io.qt.gui.QDoubleValidator Maven / Gradle / Ivy
package io.qt.gui;
import io.qt.*;
/**
* Range checking of floating-point numbers
* Java wrapper for Qt class QDoubleValidator
*/
public class QDoubleValidator extends io.qt.gui.QValidator
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QDoubleValidator.class);
/**
* Java wrapper for Qt enum QDoubleValidator::Notation
*/
public enum Notation implements QtEnumerator {
/**
* Representing QDoubleValidator:: StandardNotation
*/
StandardNotation(0),
/**
* Representing QDoubleValidator:: ScientificNotation
*/
ScientificNotation(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private Notation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Notation resolve(int value) {
switch (value) {
case 0: return StandardNotation;
case 1: return ScientificNotation;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QDoubleValidator:: bottomChanged(double)
*/
@QtPropertyNotify(name="bottom")
public final @NonNull Signal1 bottomChanged = new Signal1<>();
/**
* See QDoubleValidator:: decimalsChanged(int)
*/
@QtPropertyNotify(name="decimals")
public final @NonNull Signal1 decimalsChanged = new Signal1<>();
/**
* See QDoubleValidator:: notationChanged(QDoubleValidator::Notation)
*/
@QtPropertyNotify(name="notation")
public final @NonNull Signal1 notationChanged = new Signal1<>();
/**
* See QDoubleValidator:: topChanged(double)
*/
@QtPropertyNotify(name="top")
public final @NonNull Signal1 topChanged = new Signal1<>();
/**
* See QDoubleValidator:: QDoubleValidator(QObject*)
* @param parent
*/
public QDoubleValidator(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QDoubleValidator instance, io.qt.core.QObject parent);
/**
* See QDoubleValidator:: QDoubleValidator(double, double, int, QObject*)
* @param bottom
* @param top
* @param decimals
* @param parent
*/
public QDoubleValidator(double bottom, double top, int decimals, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, bottom, top, decimals, parent);
}
private native static void initialize_native(QDoubleValidator instance, double bottom, double top, int decimals, io.qt.core.QObject parent);
/**
* See QDoubleValidator:: bottom()const
* @return
*/
@QtPropertyReader(name="bottom")
@QtUninvokable
public final double bottom(){
return bottom_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native double bottom_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator:: decimals()const
* @return
*/
@QtPropertyReader(name="decimals")
@QtUninvokable
public final int decimals(){
return decimals_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int decimals_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator:: notation()const
* @return
*/
@QtPropertyReader(name="notation")
@QtUninvokable
public final io.qt.gui.QDoubleValidator.@NonNull Notation notation(){
return io.qt.gui.QDoubleValidator.Notation.resolve(notation_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int notation_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator:: setBottom(double)
* @param arg__1
*/
@QtPropertyWriter(name="bottom")
@QtUninvokable
public final void setBottom(double arg__1){
setBottom_native_double(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@QtUninvokable
private native void setBottom_native_double(long __this__nativeId, double arg__1);
/**
* See QDoubleValidator:: setDecimals(int)
* @param arg__1
*/
@QtPropertyWriter(name="decimals")
@QtUninvokable
public final void setDecimals(int arg__1){
setDecimals_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@QtUninvokable
private native void setDecimals_native_int(long __this__nativeId, int arg__1);
/**
* See QDoubleValidator:: setNotation(QDoubleValidator::Notation)
* @param arg__1
*/
@QtPropertyWriter(name="notation")
@QtUninvokable
public final void setNotation(io.qt.gui.QDoubleValidator.@NonNull Notation arg__1){
setNotation_native_QDoubleValidator_Notation(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@QtUninvokable
private native void setNotation_native_QDoubleValidator_Notation(long __this__nativeId, int arg__1);
/**
* See QDoubleValidator:: setRange(double, double)
* @param bottom
* @param top
*/
@QtUninvokable
public final void setRange(double bottom, double top){
setRange_native_double_double(QtJambi_LibraryUtilities.internal.nativeId(this), bottom, top);
}
@QtUninvokable
private native void setRange_native_double_double(long __this__nativeId, double bottom, double top);
/**
* See QDoubleValidator:: setRange(double, double, int)
* @param bottom
* @param top
* @param decimals
*/
@QtUninvokable
public final void setRange(double bottom, double top, int decimals){
setRange_native_double_double_int(QtJambi_LibraryUtilities.internal.nativeId(this), bottom, top, decimals);
}
@QtUninvokable
private native void setRange_native_double_double_int(long __this__nativeId, double bottom, double top, int decimals);
/**
* See QDoubleValidator:: setTop(double)
* @param arg__1
*/
@QtPropertyWriter(name="top")
@QtUninvokable
public final void setTop(double arg__1){
setTop_native_double(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@QtUninvokable
private native void setTop_native_double(long __this__nativeId, double arg__1);
/**
* See QDoubleValidator:: top()const
* @return
*/
@QtPropertyReader(name="top")
@QtUninvokable
public final double top(){
return top_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native double top_native_constfct(long __this__nativeId);
/**
* See QValidator:: fixup(QString&)const
* @param input
*/
@QtUninvokable
@Override
public void fixup(io.qt.core.@StrictNonNull QString input){
java.util.Objects.requireNonNull(input, "Argument 'input': null not expected.");
fixup_native_ref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), input);
}
@QtUninvokable
private native void fixup_native_ref_QString_constfct(long __this__nativeId, io.qt.core.QString input);
/**
* See QValidator:: validate(QString&, int&)const
* @param arg__1
* @return
*/
@QtUninvokable
@Override
public io.qt.gui.QValidator.@NonNull State validate(io.qt.gui.QValidator.QValidationData arg__1){
return io.qt.gui.QValidator.State.resolve(validate_native_ref_QString_ref_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1));
}
@QtUninvokable
private native int validate_native_ref_QString_ref_int_constfct(long __this__nativeId, io.qt.gui.QValidator.QValidationData arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QDoubleValidator(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QDoubleValidator(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QDoubleValidator instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QDoubleValidator(io.qt.core.QObject)}
* with parent = null
.
*/
public QDoubleValidator() {
this((io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QDoubleValidator(double, double, int, io.qt.core.QObject)}
* with parent = null
.
*/
public QDoubleValidator(double bottom, double top, int decimals) {
this(bottom, top, decimals, (io.qt.core.QObject)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #bottom()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final double getBottom() {
return bottom();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #decimals()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getDecimals() {
return decimals();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #notation()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.gui.QDoubleValidator.@NonNull Notation getNotation() {
return notation();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #top()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final double getTop() {
return top();
}
}