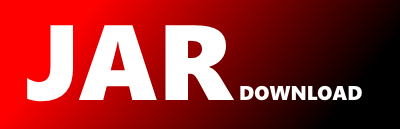
io.qt.widgets.QRubberBand Maven / Gradle / Ivy
Show all versions of qtjambi Show documentation
package io.qt.widgets;
import io.qt.*;
/**
* Rectangle or line that can indicate a selection or a boundary
* Java wrapper for Qt class QRubberBand
*/
public class QRubberBand extends io.qt.widgets.QWidget
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QRubberBand.class);
/**
* Java wrapper for Qt enum QRubberBand::Shape
*/
@QtUnlistedEnum
public enum Shape implements QtEnumerator {
/**
* Representing QRubberBand:: Line
*/
Line(0),
/**
* Representing QRubberBand:: Rectangle
*/
Rectangle(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private Shape(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Shape resolve(int value) {
switch (value) {
case 0: return Line;
case 1: return Rectangle;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QRubberBand:: QRubberBand(QRubberBand::Shape, QWidget*)
* @param arg__1
* @param arg__2
*/
public QRubberBand(io.qt.widgets.QRubberBand.@NonNull Shape arg__1, io.qt.widgets.@Nullable QWidget arg__2){
super((QPrivateConstructor)null);
initialize_native(this, arg__1, arg__2);
}
private native static void initialize_native(QRubberBand instance, io.qt.widgets.QRubberBand.Shape arg__1, io.qt.widgets.QWidget arg__2);
/**
*
* @param p
*/
@QtUninvokable
public final void moveRubberBand(io.qt.core.@NonNull QPoint p){
moveRubberBand_native_cref_QPoint(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(p));
}
@QtUninvokable
private native void moveRubberBand_native_cref_QPoint(long __this__nativeId, long p);
/**
* See QRubberBand:: move(int, int)
* @param x
* @param y
*/
@QtUninvokable
public final void moveRubberBand(int x, int y){
moveRubberBand_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), x, y);
}
@QtUninvokable
private native void moveRubberBand_native_int_int(long __this__nativeId, int x, int y);
/**
* See QRubberBand:: resize(QSize)
* @param s
*/
@QtUninvokable
public final void resizeRubberBand(io.qt.core.@NonNull QSize s){
resizeRubberBand_native_cref_QSize(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
@QtUninvokable
private native void resizeRubberBand_native_cref_QSize(long __this__nativeId, long s);
/**
* See QRubberBand:: resize(int, int)
* @param w
* @param h
*/
@QtUninvokable
public final void resizeRubberBand(int w, int h){
resizeRubberBand_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), w, h);
}
@QtUninvokable
private native void resizeRubberBand_native_int_int(long __this__nativeId, int w, int h);
/**
* See QRubberBand:: setGeometry(QRect)
* @param r
*/
@QtUninvokable
public final void setRubberBandGeometry(io.qt.core.@NonNull QRect r){
setRubberBandGeometry_native_cref_QRect(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(r));
}
@QtUninvokable
private native void setRubberBandGeometry_native_cref_QRect(long __this__nativeId, long r);
/**
* See QRubberBand:: setGeometry(int, int, int, int)
* @param x
* @param y
* @param w
* @param h
*/
@QtUninvokable
public final void setRubberBandGeometry(int x, int y, int w, int h){
setRubberBandGeometry_native_int_int_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), x, y, w, h);
}
@QtUninvokable
private native void setRubberBandGeometry_native_int_int_int_int(long __this__nativeId, int x, int y, int w, int h);
/**
*
* @return
*/
@QtUninvokable
public final io.qt.widgets.QRubberBand.@NonNull Shape shape(){
return io.qt.widgets.QRubberBand.Shape.resolve(shape_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int shape_native_constfct(long __this__nativeId);
/**
* See QWidget:: changeEvent(QEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void changeEvent(io.qt.core.@Nullable QEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
changeEvent_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void changeEvent_native_QEvent_ptr(long __this__nativeId, long arg__1);
/**
*
* @param e
* @return
*/
@QtUninvokable
@Override
public boolean event(io.qt.core.@Nullable QEvent e){
java.util.Objects.requireNonNull(e, "Argument 'e': null not expected.");
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(e));
}
@QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long e);
/**
* See QRubberBand:: initStyleOption(QStyleOptionRubberBand*)const
* @param option
*/
@QtUninvokable
protected void initStyleOption(io.qt.widgets.@Nullable QStyleOptionRubberBand option){
initStyleOption_native_QStyleOptionRubberBand_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(option));
}
@QtUninvokable
private native void initStyleOption_native_QStyleOptionRubberBand_ptr_constfct(long __this__nativeId, long option);
/**
* See QWidget:: moveEvent(QMoveEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void moveEvent(io.qt.gui.@Nullable QMoveEvent arg__1){
moveEvent_native_QMoveEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void moveEvent_native_QMoveEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: paintEvent(QPaintEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void paintEvent(io.qt.gui.@Nullable QPaintEvent arg__1){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: resizeEvent(QResizeEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void resizeEvent(io.qt.gui.@Nullable QResizeEvent arg__1){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: showEvent(QShowEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void showEvent(io.qt.gui.@Nullable QShowEvent arg__1){
showEvent_native_QShowEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void showEvent_native_QShowEvent_ptr(long __this__nativeId, long arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QRubberBand(QPrivateConstructor p) { super(p); }
/**
* Overloaded constructor for {@link #QRubberBand(io.qt.widgets.QRubberBand.Shape, io.qt.widgets.QWidget)}
* with arg__2 = null
.
*/
public QRubberBand(io.qt.widgets.QRubberBand.@NonNull Shape arg__1) {
this(arg__1, (io.qt.widgets.QWidget)null);
}
}