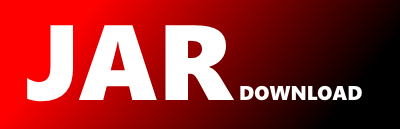
io.quarkiverse.groovy.hibernate.reactive.panache.PanacheRepositoryBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-groovy-hibernate-reactive-panache Show documentation
Show all versions of quarkus-groovy-hibernate-reactive-panache Show documentation
Simplify your persistence code for Hibernate Reactive via the active record or the repository pattern in Groovy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.quarkiverse.groovy.hibernate.reactive.panache;
import static io.quarkiverse.groovy.hibernate.reactive.panache.runtime.JpaOperations.INSTANCE;
import java.util.List;
import java.util.Map;
import java.util.stream.Stream;
import jakarta.persistence.LockModeType;
import org.hibernate.reactive.mutiny.Mutiny;
import io.quarkus.panache.common.Parameters;
import io.quarkus.panache.common.Sort;
import io.quarkus.panache.common.impl.GenerateBridge;
import io.smallrye.common.annotation.CheckReturnValue;
import io.smallrye.mutiny.Uni;
/**
*
* Represents a Repository for a specific type of entity {@code Entity}, with an ID type
* of {@code Id}. Implementing this repository will gain you the exact same useful methods
* that are on {@link PanacheEntityBase}. Unless you have a custom ID strategy, you should not
* implement this interface directly but implement {@link PanacheRepository} instead.
*
*
* @param The type of entity to operate on
* @param The ID type of the entity
*/
public interface PanacheRepositoryBase {
/**
* Returns the current {@link Mutiny.Session}
*
* @return the current {@link Mutiny.Session}
*/
default Uni getSession() {
return INSTANCE.getSession();
}
/**
* Persist the given entity in the database, if not already persisted.
*
* @param entity the entity to persist.
* @see #isPersistent(Object)
* @see #persist(Iterable)
* @see #persist(Stream)
* @see #persist(Object, Object...)
*/
@CheckReturnValue
default Uni persist(Entity entity) {
return INSTANCE.persist(entity).map(v -> entity);
}
/**
* Persist the given entity in the database, if not already persisted.
* Then flushes all pending changes to the database.
*
* @param entity the entity to persist.
* @see #isPersistent(Object)
* @see #persist(Iterable)
* @see #persist(Stream)
* @see #persist(Object, Object...)
*/
@CheckReturnValue
default Uni persistAndFlush(Entity entity) {
return INSTANCE.persist(entity)
.flatMap(v -> INSTANCE.flush())
.map(v -> entity);
}
/**
* Delete the given entity from the database, if it is already persisted.
*
* @param entity the entity to delete.
* @see #isPersistent(Object)
* @see #deleteByQuery(String, Object...)
* @see #deleteByQuery(String, Map)
* @see #deleteByQuery(String, Parameters)
* @see #deleteAll()
*/
@CheckReturnValue
default Uni delete(Entity entity) {
return INSTANCE.delete(entity);
}
/**
* Returns true if the given entity is persistent in the database. If yes, all modifications to
* its persistent fields will be automatically committed to the database at transaction
* commit time.
*
* @param entity the entity to check
* @return true if the entity is persistent in the database.
*/
default boolean isPersistent(Entity entity) {
return INSTANCE.isPersistent(entity);
}
/**
* Flushes all pending changes to the database.
*/
@CheckReturnValue
default Uni flush() {
return INSTANCE.flush();
}
// Queries
/**
* Find an entity of this type by ID.
*
* @param id the ID of the entity to find.
* @return the entity found, or null
if not found.
*/
@CheckReturnValue
@GenerateBridge
default Uni findById(Id id) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find an entity of this type by ID and lock it.
*
* @param id the ID of the entity to find.
* @param lockModeType the locking strategy to be used when retrieving the entity.
* @return the entity found, or null
if not found.
*/
@CheckReturnValue
@GenerateBridge
default Uni findById(Id id, LockModeType lockModeType) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query, with optional indexed parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params optional sequence of indexed parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Sort, Object...)
* @see #find(String, Map)
* @see #find(String, Parameters)
* @see #list(String, Object...)
*/
@GenerateBridge
default PanacheQuery find(String query, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query and the given sort options, with optional indexed parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params optional sequence of indexed parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Object...)
* @see #find(String, Sort, Map)
* @see #find(String, Sort, Parameters)
* @see #list(String, Sort, Object...)
*/
@GenerateBridge
default PanacheQuery find(String query, Sort sort, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Map} of named parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Sort, Map)
* @see #find(String, Object...)
* @see #find(String, Parameters)
* @see #list(String, Map)
*/
@GenerateBridge
default PanacheQuery find(String query, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query and the given sort options, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params {@link Map} of indexed parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Map)
* @see #find(String, Sort, Object...)
* @see #find(String, Sort, Parameters)
* @see #list(String, Sort, Map)
*/
@GenerateBridge
default PanacheQuery find(String query, Sort sort, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Parameters} of named parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Sort, Parameters)
* @see #find(String, Map)
* @see #find(String, Parameters)
* @see #list(String, Parameters)
*/
@GenerateBridge
default PanacheQuery find(String query, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities using a query and the given sort options, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params {@link Parameters} of indexed parameters
* @return a new {@link PanacheQuery} instance for the given query
* @see #find(String, Parameters)
* @see #find(String, Sort, Map)
* @see #find(String, Sort, Parameters)
* @see #list(String, Sort, Parameters)
*/
@GenerateBridge
default PanacheQuery find(String query, Sort sort, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find all entities of this type.
*
* @return a new {@link PanacheQuery} instance to find all entities of this type.
* @see #findAll(Sort)
* @see #listAll()
*/
@GenerateBridge
default PanacheQuery findAll() {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find all entities of this type, in the given order.
*
* @param sort the sort order to use
* @return a new {@link PanacheQuery} instance to find all entities of this type.
* @see #findAll()
* @see #listAll(Sort)
*/
@GenerateBridge
default PanacheQuery findAll(Sort sort) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query, with optional indexed parameters.
* This method is a shortcut for find(query, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params optional sequence of indexed parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Sort, Object...)
* @see #list(String, Map)
* @see #list(String, Parameters)
* @see #find(String, Object...)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query and the given sort options, with optional indexed parameters.
* This method is a shortcut for find(query, sort, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params optional sequence of indexed parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Object...)
* @see #list(String, Sort, Map)
* @see #list(String, Sort, Parameters)
* @see #find(String, Sort, Object...)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Sort sort, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query, with named parameters.
* This method is a shortcut for find(query, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Map} of named parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Sort, Map)
* @see #list(String, Object...)
* @see #list(String, Parameters)
* @see #find(String, Map)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query and the given sort options, with named parameters.
* This method is a shortcut for find(query, sort, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params {@link Map} of indexed parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Map)
* @see #list(String, Sort, Object...)
* @see #list(String, Sort, Parameters)
* @see #find(String, Sort, Map)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Sort sort, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query, with named parameters.
* This method is a shortcut for find(query, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Parameters} of named parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Sort, Parameters)
* @see #list(String, Object...)
* @see #list(String, Map)
* @see #find(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find entities matching a query and the given sort options, with named parameters.
* This method is a shortcut for find(query, sort, params).list()
.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param sort the sort strategy to use
* @param params {@link Parameters} of indexed parameters
* @return a {@link List} containing all results, without paging
* @see #list(String, Parameters)
* @see #list(String, Sort, Object...)
* @see #list(String, Sort, Map)
* @see #find(String, Sort, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni> list(String query, Sort sort, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find all entities of this type.
* This method is a shortcut for findAll().list()
.
*
* @return a {@link List} containing all results, without paging
* @see #listAll(Sort)
* @see #findAll()
*/
@CheckReturnValue
@GenerateBridge
default Uni> listAll() {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Find all entities of this type, in the given order.
* This method is a shortcut for findAll(sort).list()
.
*
* @param sort the sort order to use
* @return a {@link List} containing all results, without paging
* @see #listAll()
* @see #findAll(Sort)
*/
@CheckReturnValue
@GenerateBridge
default Uni> listAll(Sort sort) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Counts the number of this type of entity in the database.
*
* @return the number of this type of entity in the database.
* @see #count(String, Object...)
* @see #count(String, Map)
* @see #count(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni count() {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Counts the number of this type of entity matching the given query, with optional indexed parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params optional sequence of indexed parameters
* @return the number of entities counted.
* @see #count()
* @see #count(String, Map)
* @see #count(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni count(String query, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Counts the number of this type of entity matching the given query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Map} of named parameters
* @return the number of entities counted.
* @see #count()
* @see #count(String, Object...)
* @see #count(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni count(String query, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Counts the number of this type of entity matching the given query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Parameters} of named parameters
* @return the number of entities counted.
* @see #count()
* @see #count(String, Object...)
* @see #count(String, Map)
*/
@CheckReturnValue
@GenerateBridge
default Uni count(String query, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Delete all entities of this type from the database.
*
* WARNING: the default implementation of this method uses a bulk delete query and ignores
* cascading rules from the JPA model.
*
* @return the number of entities deleted.
* @see #deleteByQuery(String, Object...)
* @see #deleteByQuery(String, Map)
* @see #deleteByQuery(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni deleteAll() {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Delete an entity of this type by ID.
*
* @param id the ID of the entity to delete.
* @return false if the entity was not deleted (not found).
*/
@CheckReturnValue
@GenerateBridge
default Uni deleteById(Id id) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Delete all entities of this type matching the given query, with optional indexed parameters.
*
* WARNING: the default implementation of this method uses a bulk delete query and ignores
* cascading rules from the JPA model.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params optional sequence of indexed parameters
* @return the number of entities deleted.
* @see #deleteAll()
* @see #deleteByQuery(String, Map)
* @see #deleteByQuery(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni deleteByQuery(String query, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Delete all entities of this type matching the given query, with named parameters.
*
* WARNING: the default implementation of this method uses a bulk delete query and ignores
* cascading rules from the JPA model.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Map} of named parameters
* @return the number of entities deleted.
* @see #deleteAll()
* @see #deleteByQuery(String, Object...)
* @see #deleteByQuery(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni deleteByQuery(String query, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Delete all entities of this type matching the given query, with named parameters.
*
* WARNING: the default implementation of this method uses a bulk delete query and ignores
* cascading rules from the JPA model.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Parameters} of named parameters
* @return the number of entities deleted.
* @see #deleteAll()
* @see #deleteByQuery(String, Object...)
* @see #deleteByQuery(String, Map)
*/
@CheckReturnValue
@GenerateBridge
default Uni deleteByQuery(String query, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Persist all given entities.
*
* @param entities the entities to persist
* @see #persist(Object)
* @see #persist(Stream)
* @see #persist(Object,Object...)
*/
@CheckReturnValue
default Uni persist(Iterable entities) {
return INSTANCE.persist(entities);
}
/**
* Persist all given entities.
*
* @param entities the entities to persist
* @see #persist(Object)
* @see #persist(Iterable)
* @see #persist(Object,Object...)
*/
@CheckReturnValue
default Uni persist(Stream entities) {
return INSTANCE.persist(entities);
}
/**
* Persist all given entities.
*
* @param entities the entities to persist
* @see #persist(Object)
* @see #persist(Stream)
* @see #persist(Iterable)
*/
@CheckReturnValue
default Uni persist(Entity firstEntity, @SuppressWarnings("unchecked") Entity... entities) {
return INSTANCE.persist(firstEntity, entities);
}
/**
* Update all entities of this type matching the given query, with optional indexed parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params optional sequence of indexed parameters
* @return the number of entities updated.
* @see #update(String, Map)
* @see #update(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni update(String query, Object... params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Update all entities of this type matching the given query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Map} of named parameters
* @return the number of entities updated.
* @see #update(String, Object...)
* @see #update(String, Parameters)
*/
@CheckReturnValue
@GenerateBridge
default Uni update(String query, Map params) {
throw INSTANCE.implementationInjectionMissing();
}
/**
* Update all entities of this type matching the given query, with named parameters.
*
* @param query a {@link io.quarkus.hibernate.reactive.panache query string}
* @param params {@link Parameters} of named parameters
* @return the number of entities updated.
* @see #update(String, Object...)
* @see #update(String, Map)
*/
@CheckReturnValue
@GenerateBridge
default Uni update(String query, Parameters params) {
throw INSTANCE.implementationInjectionMissing();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy