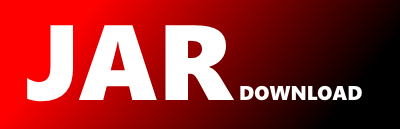
io.quarkiverse.groovy.hibernate.reactive.panache.runtime.PanacheQueryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-groovy-hibernate-reactive-panache Show documentation
Show all versions of quarkus-groovy-hibernate-reactive-panache Show documentation
Simplify your persistence code for Hibernate Reactive via the active record or the repository pattern in Groovy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.quarkiverse.groovy.hibernate.reactive.panache.runtime;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import jakarta.persistence.LockModeType;
import org.hibernate.reactive.mutiny.Mutiny;
import io.quarkiverse.groovy.hibernate.reactive.panache.PanacheQuery;
import io.quarkus.hibernate.reactive.panache.common.runtime.CommonPanacheQueryImpl;
import io.quarkus.panache.common.Page;
import io.quarkus.panache.common.Parameters;
import io.smallrye.mutiny.Uni;
public class PanacheQueryImpl implements PanacheQuery {
private final CommonPanacheQueryImpl delegate;
PanacheQueryImpl(Uni em, String query, String originalQuery, String orderBy, Object paramsArrayOrMap) {
this.delegate = new CommonPanacheQueryImpl<>(em, query, originalQuery, orderBy, paramsArrayOrMap);
}
protected PanacheQueryImpl(CommonPanacheQueryImpl delegate) {
this.delegate = delegate;
}
// Builder
@Override
public PanacheQuery project(Class type) {
return new PanacheQueryImpl<>(delegate.project(type));
}
@Override
@SuppressWarnings("unchecked")
public PanacheQuery page(Page page) {
delegate.page(page);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery page(int pageIndex, int pageSize) {
delegate.page(pageIndex, pageSize);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery nextPage() {
delegate.nextPage();
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery previousPage() {
delegate.previousPage();
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery firstPage() {
delegate.firstPage();
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public Uni> lastPage() {
return delegate.lastPage().map(v -> (PanacheQuery) this);
}
@Override
public Uni hasNextPage() {
return delegate.hasNextPage();
}
@Override
public boolean hasPreviousPage() {
return delegate.hasPreviousPage();
}
@Override
public Uni pageCount() {
return delegate.pageCount();
}
@Override
public Page page() {
return delegate.page();
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery range(int startIndex, int lastIndex) {
delegate.range(startIndex, lastIndex);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery withLock(LockModeType lockModeType) {
delegate.withLock(lockModeType);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery withHint(String hintName, Object value) {
delegate.withHint(hintName, value);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery filter(String filterName, Parameters parameters) {
delegate.filter(filterName, parameters.map());
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery filter(String filterName, Map parameters) {
delegate.filter(filterName, parameters);
return (PanacheQuery) this;
}
@SuppressWarnings("unchecked")
@Override
public PanacheQuery filter(String filterName) {
delegate.filter(filterName, Collections.emptyMap());
return (PanacheQuery) this;
}
// Results
@Override
public Uni count() {
return delegate.count();
}
@Override
public Uni> list() {
return delegate.list();
}
@Override
public Uni firstResult() {
return delegate.firstResult();
}
@Override
public Uni singleResult() {
return delegate.singleResult();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy