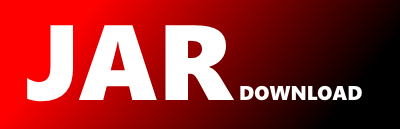
io.quarkiverse.jsonrpc.sample.ScopedHelloResource Maven / Gradle / Ivy
package io.quarkiverse.jsonrpc.sample;
import java.time.Duration;
import io.quarkiverse.jsonrpc.runtime.api.JsonRPCApi;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
@JsonRPCApi("scoped")
public class ScopedHelloResource {
public String hello() {
return "Hello scoped " + " [" + Thread.currentThread().getName() + "]";
}
public String hello(String name) {
return "Hello scoped " + name + " [" + Thread.currentThread().getName() + "]";
}
public String hello(String name, String surname) {
return "Hello scoped " + name + " " + surname + " [" + Thread.currentThread().getName() + "]";
}
public Uni helloUni() {
return Uni.createFrom().item(hello());
}
public Uni helloUni(String name) {
return Uni.createFrom().item(hello(name));
}
public Uni helloUni(String name, String surname) {
return Uni.createFrom().item(hello(name, surname));
}
public Multi helloMulti() {
return Multi.createFrom().ticks().every(Duration.ofSeconds(1))
.onItem().transform(n -> "(" + n + ") " + hello());
}
public Multi helloMulti(String name) {
return Multi.createFrom().ticks().every(Duration.ofSeconds(1))
.onItem().transform(n -> "(" + n + ") " + hello(name));
}
public Multi helloMulti(String name, String surname) {
return Multi.createFrom().ticks().every(Duration.ofSeconds(1))
.onItem().transform(n -> "(" + n + ") " + hello(name, surname));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy