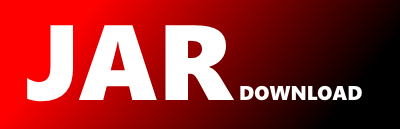
io.quarkiverse.langchain4j.runtime.ToolsRecorder Maven / Gradle / Ivy
package io.quarkiverse.langchain4j.runtime;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.jboss.logging.Logger;
import dev.langchain4j.agent.tool.ToolSpecification;
import dev.langchain4j.service.tool.ToolExecutor;
import io.quarkiverse.langchain4j.runtime.tool.QuarkusToolExecutor;
import io.quarkiverse.langchain4j.runtime.tool.QuarkusToolExecutorFactory;
import io.quarkiverse.langchain4j.runtime.tool.ToolMethodCreateInfo;
import io.quarkus.arc.Arc;
import io.quarkus.runtime.annotations.Recorder;
@Recorder
public class ToolsRecorder {
private static final Logger log = Logger.getLogger(ToolsRecorder.class);
// the key is the class' name
private static final Map> metadata = new ConcurrentHashMap<>();
public void setMetadata(Map> metadata) {
ToolsRecorder.metadata.putAll(metadata);
}
public static Map> getMetadata() {
return metadata;
}
public static void clearMetadata() {
metadata.clear();
}
public static void populateToolMetadata(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy