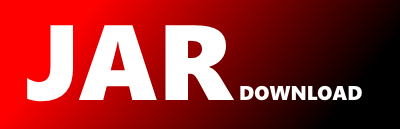
io.quarkiverse.mailpit.test.model.HTMLCheckWarning Maven / Gradle / Ivy
The newest version!
/*
* Mailpit API
* OpenAPI 2.0 documentation for [Mailpit](https://github.com/axllent/mailpit).
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.quarkiverse.mailpit.test.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.quarkiverse.mailpit.test.model.HTMLCheckResult;
import io.quarkiverse.mailpit.test.model.HTMLCheckScore;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import io.quarkiverse.mailpit.test.invoker.ApiClient;
/**
* Warning represents a failed test
*/
@JsonPropertyOrder({
HTMLCheckWarning.JSON_PROPERTY_CATEGORY,
HTMLCheckWarning.JSON_PROPERTY_DESCRIPTION,
HTMLCheckWarning.JSON_PROPERTY_KEYWORDS,
HTMLCheckWarning.JSON_PROPERTY_NOTES_BY_NUMBER,
HTMLCheckWarning.JSON_PROPERTY_RESULTS,
HTMLCheckWarning.JSON_PROPERTY_SCORE,
HTMLCheckWarning.JSON_PROPERTY_SLUG,
HTMLCheckWarning.JSON_PROPERTY_TAGS,
HTMLCheckWarning.JSON_PROPERTY_TITLE,
HTMLCheckWarning.JSON_PROPERTY_U_R_L
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.9.0")
public class HTMLCheckWarning {
public static final String JSON_PROPERTY_CATEGORY = "Category";
private String category;
public static final String JSON_PROPERTY_DESCRIPTION = "Description";
private String description;
public static final String JSON_PROPERTY_KEYWORDS = "Keywords";
private String keywords;
public static final String JSON_PROPERTY_NOTES_BY_NUMBER = "NotesByNumber";
private Map notesByNumber = new HashMap<>();
public static final String JSON_PROPERTY_RESULTS = "Results";
private List results = new ArrayList<>();
public static final String JSON_PROPERTY_SCORE = "Score";
private HTMLCheckScore score;
public static final String JSON_PROPERTY_SLUG = "Slug";
private String slug;
public static final String JSON_PROPERTY_TAGS = "Tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_TITLE = "Title";
private String title;
public static final String JSON_PROPERTY_U_R_L = "URL";
private String URL;
public HTMLCheckWarning() {
}
public HTMLCheckWarning category(String category) {
this.category = category;
return this;
}
/**
* Category [css, html]
* @return category
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCategory() {
return category;
}
@JsonProperty(JSON_PROPERTY_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCategory(String category) {
this.category = category;
}
public HTMLCheckWarning description(String description) {
this.description = description;
return this;
}
/**
* Description
* @return description
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public HTMLCheckWarning keywords(String keywords) {
this.keywords = keywords;
return this;
}
/**
* Keywords
* @return keywords
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_KEYWORDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKeywords() {
return keywords;
}
@JsonProperty(JSON_PROPERTY_KEYWORDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKeywords(String keywords) {
this.keywords = keywords;
}
public HTMLCheckWarning notesByNumber(Map notesByNumber) {
this.notesByNumber = notesByNumber;
return this;
}
public HTMLCheckWarning putNotesByNumberItem(String key, String notesByNumberItem) {
if (this.notesByNumber == null) {
this.notesByNumber = new HashMap<>();
}
this.notesByNumber.put(key, notesByNumberItem);
return this;
}
/**
* Notes based on results
* @return notesByNumber
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NOTES_BY_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getNotesByNumber() {
return notesByNumber;
}
@JsonProperty(JSON_PROPERTY_NOTES_BY_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNotesByNumber(Map notesByNumber) {
this.notesByNumber = notesByNumber;
}
public HTMLCheckWarning results(List results) {
this.results = results;
return this;
}
public HTMLCheckWarning addResultsItem(HTMLCheckResult resultsItem) {
if (this.results == null) {
this.results = new ArrayList<>();
}
this.results.add(resultsItem);
return this;
}
/**
* Test results
* @return results
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RESULTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getResults() {
return results;
}
@JsonProperty(JSON_PROPERTY_RESULTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResults(List results) {
this.results = results;
}
public HTMLCheckWarning score(HTMLCheckScore score) {
this.score = score;
return this;
}
/**
* Get score
* @return score
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SCORE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HTMLCheckScore getScore() {
return score;
}
@JsonProperty(JSON_PROPERTY_SCORE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScore(HTMLCheckScore score) {
this.score = score;
}
public HTMLCheckWarning slug(String slug) {
this.slug = slug;
return this;
}
/**
* Slug identifier
* @return slug
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SLUG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSlug() {
return slug;
}
@JsonProperty(JSON_PROPERTY_SLUG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSlug(String slug) {
this.slug = slug;
}
public HTMLCheckWarning tags(List tags) {
this.tags = tags;
return this;
}
public HTMLCheckWarning addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Tags
* @return tags
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public HTMLCheckWarning title(String title) {
this.title = title;
return this;
}
/**
* Friendly title
* @return title
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public HTMLCheckWarning URL(String URL) {
this.URL = URL;
return this;
}
/**
* URL to caniemail.com
* @return URL
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getURL() {
return URL;
}
@JsonProperty(JSON_PROPERTY_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setURL(String URL) {
this.URL = URL;
}
/**
* Return true if this HTMLCheckWarning object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HTMLCheckWarning htMLCheckWarning = (HTMLCheckWarning) o;
return Objects.equals(this.category, htMLCheckWarning.category) &&
Objects.equals(this.description, htMLCheckWarning.description) &&
Objects.equals(this.keywords, htMLCheckWarning.keywords) &&
Objects.equals(this.notesByNumber, htMLCheckWarning.notesByNumber) &&
Objects.equals(this.results, htMLCheckWarning.results) &&
Objects.equals(this.score, htMLCheckWarning.score) &&
Objects.equals(this.slug, htMLCheckWarning.slug) &&
Objects.equals(this.tags, htMLCheckWarning.tags) &&
Objects.equals(this.title, htMLCheckWarning.title) &&
Objects.equals(this.URL, htMLCheckWarning.URL);
}
@Override
public int hashCode() {
return Objects.hash(category, description, keywords, notesByNumber, results, score, slug, tags, title, URL);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HTMLCheckWarning {\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" keywords: ").append(toIndentedString(keywords)).append("\n");
sb.append(" notesByNumber: ").append(toIndentedString(notesByNumber)).append("\n");
sb.append(" results: ").append(toIndentedString(results)).append("\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" slug: ").append(toIndentedString(slug)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" URL: ").append(toIndentedString(URL)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `Category` to the URL query string
if (getCategory() != null) {
joiner.add(String.format("%sCategory%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCategory()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sDescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Keywords` to the URL query string
if (getKeywords() != null) {
joiner.add(String.format("%sKeywords%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getKeywords()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `NotesByNumber` to the URL query string
if (getNotesByNumber() != null) {
for (String _key : getNotesByNumber().keySet()) {
joiner.add(String.format("%sNotesByNumber%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getNotesByNumber().get(_key), URLEncoder.encode(ApiClient.valueToString(getNotesByNumber().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `Results` to the URL query string
if (getResults() != null) {
for (int i = 0; i < getResults().size(); i++) {
if (getResults().get(i) != null) {
joiner.add(getResults().get(i).toUrlQueryString(String.format("%sResults%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `Score` to the URL query string
if (getScore() != null) {
joiner.add(getScore().toUrlQueryString(prefix + "Score" + suffix));
}
// add `Slug` to the URL query string
if (getSlug() != null) {
joiner.add(String.format("%sSlug%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSlug()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
joiner.add(String.format("%sTags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `Title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%sTitle%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `URL` to the URL query string
if (getURL() != null) {
joiner.add(String.format("%sURL%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getURL()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy