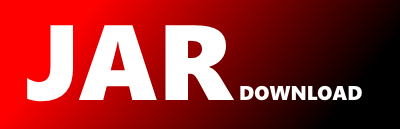
io.quarkiverse.mailpit.test.model.MessageSummary Maven / Gradle / Ivy
The newest version!
/*
* Mailpit API
* OpenAPI 2.0 documentation for [Mailpit](https://github.com/axllent/mailpit).
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.quarkiverse.mailpit.test.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.quarkiverse.mailpit.test.model.Address;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import io.quarkiverse.mailpit.test.invoker.ApiClient;
/**
* MessageSummary struct for frontend messages
*/
@JsonPropertyOrder({
MessageSummary.JSON_PROPERTY_ATTACHMENTS,
MessageSummary.JSON_PROPERTY_BCC,
MessageSummary.JSON_PROPERTY_CC,
MessageSummary.JSON_PROPERTY_CREATED,
MessageSummary.JSON_PROPERTY_FROM,
MessageSummary.JSON_PROPERTY_I_D,
MessageSummary.JSON_PROPERTY_MESSAGE_I_D,
MessageSummary.JSON_PROPERTY_READ,
MessageSummary.JSON_PROPERTY_SIZE,
MessageSummary.JSON_PROPERTY_SUBJECT,
MessageSummary.JSON_PROPERTY_TAGS,
MessageSummary.JSON_PROPERTY_TO
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.9.0")
public class MessageSummary {
public static final String JSON_PROPERTY_ATTACHMENTS = "Attachments";
private Long attachments;
public static final String JSON_PROPERTY_BCC = "Bcc";
private List bcc = new ArrayList<>();
public static final String JSON_PROPERTY_CC = "Cc";
private List cc = new ArrayList<>();
public static final String JSON_PROPERTY_CREATED = "Created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_FROM = "From";
private Address from;
public static final String JSON_PROPERTY_I_D = "ID";
private String ID;
public static final String JSON_PROPERTY_MESSAGE_I_D = "MessageID";
private String messageID;
public static final String JSON_PROPERTY_READ = "Read";
private Boolean read;
public static final String JSON_PROPERTY_SIZE = "Size";
private Long size;
public static final String JSON_PROPERTY_SUBJECT = "Subject";
private String subject;
public static final String JSON_PROPERTY_TAGS = "Tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_TO = "To";
private List to = new ArrayList<>();
public MessageSummary() {
}
public MessageSummary attachments(Long attachments) {
this.attachments = attachments;
return this;
}
/**
* Whether the message has any attachments
* @return attachments
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ATTACHMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getAttachments() {
return attachments;
}
@JsonProperty(JSON_PROPERTY_ATTACHMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAttachments(Long attachments) {
this.attachments = attachments;
}
public MessageSummary bcc(List bcc) {
this.bcc = bcc;
return this;
}
public MessageSummary addBccItem(Address bccItem) {
if (this.bcc == null) {
this.bcc = new ArrayList<>();
}
this.bcc.add(bccItem);
return this;
}
/**
* Bcc addresses
* @return bcc
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_BCC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getBcc() {
return bcc;
}
@JsonProperty(JSON_PROPERTY_BCC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBcc(List bcc) {
this.bcc = bcc;
}
public MessageSummary cc(List cc) {
this.cc = cc;
return this;
}
public MessageSummary addCcItem(Address ccItem) {
if (this.cc == null) {
this.cc = new ArrayList<>();
}
this.cc.add(ccItem);
return this;
}
/**
* Cc addresses
* @return cc
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCc() {
return cc;
}
@JsonProperty(JSON_PROPERTY_CC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCc(List cc) {
this.cc = cc;
}
public MessageSummary created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* Created time
* @return created
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public MessageSummary from(Address from) {
this.from = from;
return this;
}
/**
* Get from
* @return from
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Address getFrom() {
return from;
}
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrom(Address from) {
this.from = from;
}
public MessageSummary ID(String ID) {
this.ID = ID;
return this;
}
/**
* Database ID
* @return ID
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getID() {
return ID;
}
@JsonProperty(JSON_PROPERTY_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setID(String ID) {
this.ID = ID;
}
public MessageSummary messageID(String messageID) {
this.messageID = messageID;
return this;
}
/**
* Message ID
* @return messageID
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MESSAGE_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMessageID() {
return messageID;
}
@JsonProperty(JSON_PROPERTY_MESSAGE_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMessageID(String messageID) {
this.messageID = messageID;
}
public MessageSummary read(Boolean read) {
this.read = read;
return this;
}
/**
* Read status
* @return read
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_READ)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getRead() {
return read;
}
@JsonProperty(JSON_PROPERTY_READ)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRead(Boolean read) {
this.read = read;
}
public MessageSummary size(Long size) {
this.size = size;
return this;
}
/**
* Message size in bytes (total)
* @return size
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSize() {
return size;
}
@JsonProperty(JSON_PROPERTY_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSize(Long size) {
this.size = size;
}
public MessageSummary subject(String subject) {
this.subject = subject;
return this;
}
/**
* Email subject
* @return subject
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSubject(String subject) {
this.subject = subject;
}
public MessageSummary tags(List tags) {
this.tags = tags;
return this;
}
public MessageSummary addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Message tags
* @return tags
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public MessageSummary to(List to) {
this.to = to;
return this;
}
public MessageSummary addToItem(Address toItem) {
if (this.to == null) {
this.to = new ArrayList<>();
}
this.to.add(toItem);
return this;
}
/**
* To address
* @return to
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTo() {
return to;
}
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTo(List to) {
this.to = to;
}
/**
* Return true if this MessageSummary object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MessageSummary messageSummary = (MessageSummary) o;
return Objects.equals(this.attachments, messageSummary.attachments) &&
Objects.equals(this.bcc, messageSummary.bcc) &&
Objects.equals(this.cc, messageSummary.cc) &&
Objects.equals(this.created, messageSummary.created) &&
Objects.equals(this.from, messageSummary.from) &&
Objects.equals(this.ID, messageSummary.ID) &&
Objects.equals(this.messageID, messageSummary.messageID) &&
Objects.equals(this.read, messageSummary.read) &&
Objects.equals(this.size, messageSummary.size) &&
Objects.equals(this.subject, messageSummary.subject) &&
Objects.equals(this.tags, messageSummary.tags) &&
Objects.equals(this.to, messageSummary.to);
}
@Override
public int hashCode() {
return Objects.hash(attachments, bcc, cc, created, from, ID, messageID, read, size, subject, tags, to);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MessageSummary {\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" bcc: ").append(toIndentedString(bcc)).append("\n");
sb.append(" cc: ").append(toIndentedString(cc)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" messageID: ").append(toIndentedString(messageID)).append("\n");
sb.append(" read: ").append(toIndentedString(read)).append("\n");
sb.append(" size: ").append(toIndentedString(size)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `Attachments` to the URL query string
if (getAttachments() != null) {
joiner.add(String.format("%sAttachments%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAttachments()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Bcc` to the URL query string
if (getBcc() != null) {
for (int i = 0; i < getBcc().size(); i++) {
if (getBcc().get(i) != null) {
joiner.add(getBcc().get(i).toUrlQueryString(String.format("%sBcc%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `Cc` to the URL query string
if (getCc() != null) {
for (int i = 0; i < getCc().size(); i++) {
if (getCc().get(i) != null) {
joiner.add(getCc().get(i).toUrlQueryString(String.format("%sCc%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `Created` to the URL query string
if (getCreated() != null) {
joiner.add(String.format("%sCreated%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCreated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `From` to the URL query string
if (getFrom() != null) {
joiner.add(getFrom().toUrlQueryString(prefix + "From" + suffix));
}
// add `ID` to the URL query string
if (getID() != null) {
joiner.add(String.format("%sID%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getID()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `MessageID` to the URL query string
if (getMessageID() != null) {
joiner.add(String.format("%sMessageID%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMessageID()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Read` to the URL query string
if (getRead() != null) {
joiner.add(String.format("%sRead%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRead()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Size` to the URL query string
if (getSize() != null) {
joiner.add(String.format("%sSize%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSize()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Subject` to the URL query string
if (getSubject() != null) {
joiner.add(String.format("%sSubject%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSubject()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `Tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
joiner.add(String.format("%sTags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `To` to the URL query string
if (getTo() != null) {
for (int i = 0; i < getTo().size(); i++) {
if (getTo().get(i) != null) {
joiner.add(getTo().get(i).toUrlQueryString(String.format("%sTo%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy