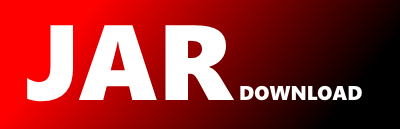
io.quarkiverse.mailpit.test.rest.TestingApi Maven / Gradle / Ivy
The newest version!
/*
* Mailpit API
* OpenAPI 2.0 documentation for [Mailpit](https://github.com/axllent/mailpit).
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.quarkiverse.mailpit.test.rest;
import io.quarkiverse.mailpit.test.invoker.ApiClient;
import io.quarkiverse.mailpit.test.invoker.ApiException;
import io.quarkiverse.mailpit.test.invoker.ApiResponse;
import io.quarkiverse.mailpit.test.invoker.Pair;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.9.0")
public class TestingApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public TestingApi() {
this(new ApiClient());
}
public TestingApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Render message HTML part
* Renders just the message's HTML part which can be used for UI integration testing. Attached inline images are modified to link to the API provided they exist. Note that is the message does not contain a HTML part then an 404 error is returned. The ID can be set to `latest` to return the latest message.
* @param ID Database ID or latest (required)
* @throws ApiException if fails to make API call
*/
public void getMessageHTML(String ID) throws ApiException {
getMessageHTMLWithHttpInfo(ID);
}
/**
* Render message HTML part
* Renders just the message's HTML part which can be used for UI integration testing. Attached inline images are modified to link to the API provided they exist. Note that is the message does not contain a HTML part then an 404 error is returned. The ID can be set to `latest` to return the latest message.
* @param ID Database ID or latest (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse getMessageHTMLWithHttpInfo(String ID) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getMessageHTMLRequestBuilder(ID);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getMessageHTML", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getMessageHTMLRequestBuilder(String ID) throws ApiException {
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getMessageHTML");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/view/{ID}.html"
.replace("{ID}", ApiClient.urlEncode(ID.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Render message text part
* Renders just the message's text part which can be used for UI integration testing. The ID can be set to `latest` to return the latest message.
* @param ID Database ID or latest (required)
* @throws ApiException if fails to make API call
*/
public void getMessageText(String ID) throws ApiException {
getMessageTextWithHttpInfo(ID);
}
/**
* Render message text part
* Renders just the message's text part which can be used for UI integration testing. The ID can be set to `latest` to return the latest message.
* @param ID Database ID or latest (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse getMessageTextWithHttpInfo(String ID) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getMessageTextRequestBuilder(ID);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getMessageText", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getMessageTextRequestBuilder(String ID) throws ApiException {
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getMessageText");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/view/{ID}.txt"
.replace("{ID}", ApiClient.urlEncode(ID.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy