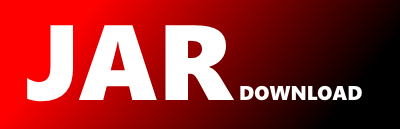
io.quarkiverse.reactive.messaging.nats.jetstream.JetStreamBuildConfiguration Maven / Gradle / Ivy
package io.quarkiverse.reactive.messaging.nats.jetstream;
import java.time.Duration;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import io.nats.client.api.*;
import io.quarkus.runtime.annotations.ConfigPhase;
import io.quarkus.runtime.annotations.ConfigRoot;
import io.smallrye.config.ConfigMapping;
import io.smallrye.config.WithDefault;
@ConfigMapping(prefix = "quarkus.messaging.nats.jet-stream")
@ConfigRoot(phase = ConfigPhase.BUILD_AND_RUN_TIME_FIXED)
public interface JetStreamBuildConfiguration {
/**
* Autoconfigure stream and subjects based on channel configuration
*/
@WithDefault("true")
Boolean autoConfigure();
/**
* If auto-configure is true the streams are created on Nats server.
*/
List streams();
/**
* If auto-configure is true the key-value stores are created on Nats server.
*/
List keyValueStores();
interface KeyValueStore {
/**
* Name of Key-Value store
*/
String bucketName();
/**
* Description of Key-Value store
*/
Optional description();
/**
* The storage type (File or Memory).
*/
@WithDefault("File")
StorageType storageType();
/**
* The maximum number of bytes for this bucket
*/
Optional maxBucketSize();
/**
* The maximum number of history for any one key. Includes the current value.
*/
Optional maxHistoryPerKey();
/**
* The maximum size for an individual value in the bucket.
*/
Optional maxValueSize();
/**
* The maximum age for a value in this bucket
*/
Optional ttl();
/**
* The number of replicas for this bucket
*/
Optional replicas();
/**
* Sets whether to use compression
*/
@WithDefault("true")
Boolean compressed();
}
interface Stream {
/**
* If the stream already exists, it will be overwritten
*/
@WithDefault("false")
Boolean overwrite();
/**
* Name of stream
*/
String name();
/**
* Description of stream
*/
Optional description();
/**
* Stream subjects
*/
Set subjects();
/**
* The number of replicas a message must be stored. Default value is 1.
*/
@WithDefault("1")
Integer replicas();
/**
* The storage type for stream data (File or Memory).
*/
@WithDefault("File")
StorageType storageType();
/**
* Declares the retention policy for the stream. @see
* Retention Policy
*/
@WithDefault("Interest")
RetentionPolicy retentionPolicy();
/**
* The compression option for this stream
*/
@WithDefault("none")
CompressionOption compressionOption();
/**
* The maximum number of consumers for this stream
*/
Optional maximumConsumers();
/**
* The maximum messages for this stream
*/
Optional maximumMessages();
/**
* The maximum messages per subject for this stream
*/
Optional maximumMessagesPerSubject();
/**
* The maximum number of bytes for this stream
*/
Optional maximumBytes();
/**
* the maximum message age for this stream
*/
Optional maximumAge();
/**
* The maximum message size for this stream
*/
Optional maximumMessageSize();
/**
* The template json for this stream
*/
Optional templateOwner();
/**
* The discard policy for this stream
*/
Optional discardPolicy();
/**
* The duplicate checking window stream configuration. Duration. ZERO means duplicate checking is not enabled
*/
Optional duplicateWindow();
/**
* The flag indicating if the stream allows rollup
*/
Optional allowRollup();
/**
* The flag indicating if the stream allows direct message access
*/
Optional allowDirect();
/**
* The flag indicating if the stream allows higher performance and unified direct access for mirrors as well
*/
Optional mirrorDirect();
/**
* The flag indicating if deny delete is set for the stream
*/
Optional denyDelete();
/**
* The flag indicating if deny purge is set for the stream
*/
Optional denyPurge();
/**
* Whether discard policy with max message per subject is applied per subject
*/
Optional discardNewPerSubject();
/**
* The first sequence used in the stream
*/
Optional firstSequence();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy