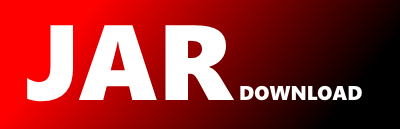
io.quarkiverse.renarde.router.Router Maven / Gradle / Ivy
The newest version!
package io.quarkiverse.renarde.router;
import java.net.URI;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import jakarta.ws.rs.core.UriBuilder;
import jakarta.ws.rs.core.UriInfo;
import org.eclipse.microprofile.config.Config;
import org.eclipse.microprofile.config.ConfigProvider;
import io.quarkus.arc.Arc;
public class Router {
public static URI getURI(Method0 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method0V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method1 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method1V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method2 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method2V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method3 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method3V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method4 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method4V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method5 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method5V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(Method6 method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(Method6V method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(Method7 method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(Method7V method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(Method8 method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(Method8V method,
Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method9 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method9V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method10 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method10V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method11 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method11V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method12 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method12V method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method13 method, Object... params) {
return findURI(method, params);
}
public static URI getURI(
Method13V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method0 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method0V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method1 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method1V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method2 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method2V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method3 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method3V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method4 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method4V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method5 method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method5V method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method6 method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method6V method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method7 method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(Method7V method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method8 method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method8V method,
Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method9 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method9V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method10 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method10V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method11 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method11V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method12 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method12V method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method13 method, Object... params) {
return findURI(method, params);
}
public static URI getAbsoluteURI(
Method13V method, Object... params) {
return findURI(method, params);
}
private static URI findURI(Object method, Object... params) {
// make sure all the calls are instrumented
throw new RuntimeException("This call should have been instrumented away");
}
public static URI findURI(String route, boolean absolute, Object... params) {
// This is only used by the views
RouterMethod routerMethod = routerMethods.get(route);
if (routerMethod == null)
throw new RuntimeException("No route defined for " + route);
return routerMethod.getRoute(absolute, params);
}
private static Map routerMethods = new HashMap<>();
// Called by generated class __RenardeInit
public static void clearRoutes() {
routerMethods.clear();
}
// Called by generated class __RenardeInit for each controller route
public static void registerRoute(String route, RouterMethod method) {
if (routerMethods.containsKey(route)) {
System.err.println("WARNING: duplicate route registered for " + route);
}
routerMethods.put(route, method);
}
// Used by generated bytecode for each controller method, which has a corresponding method to build a URI to it (it's only called in testing)
public static UriBuilder getTestUriBuilder(boolean absolute) {
Config config = ConfigProvider.getConfig();
var uri = "http://" + config.getConfigValue("quarkus.http.host").getValue() + ":"
+ config.getConfigValue("quarkus.http.test-port").getValue()
+ config.getConfigValue("quarkus.http.root-path").getValue();
UriBuilder uriBuilder = UriBuilder.fromUri(uri);
return absolute ? uriBuilder : uriBuilder.host(null).port(-1).scheme(null);
}
// Used by generated bytecode for each controller method, which has a corresponding method to build a URI to it
public static UriBuilder getUriBuilder(boolean absolute) {
UriInfo uriInfo = Arc.container().instance(UriInfo.class).get();
UriBuilder ret = absolute ? uriInfo.getBaseUriBuilder()
: uriInfo.getBaseUriBuilder().host(null).port(-1).scheme(null);
return ret;
}
// Called by the URI-generating methods of controllers, for Optional parameters
public static Optional ofNullable(Object o) {
if (o instanceof Optional) {
return (Optional) o;
}
return Optional.ofNullable(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy