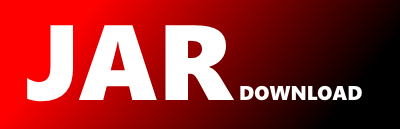
web.lib.core.components.debounced-text-input.tsx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of code-quarkus Show documentation
Show all versions of code-quarkus Show documentation
Customize a Web Interface to generate Quarkus starter projects.
import * as React from 'react';
import { useDebounce } from './use-debounce';
import { Form } from 'react-bootstrap';
export interface DebouncedTextInputProps {
value: string
delay?: number;
isValid?: boolean;
className?: string;
onChange?: (string) => void;
}
export function DebouncedTextInput(props: DebouncedTextInputProps) {
const { value, onChange, isValid, ...rest } = props;
const [ localValue, setLocalValue ] = React.useState(value);
const [ prevValue, setPrevValue ] = React.useState(undefined);
React.useEffect(() => {
if (value !== prevValue) {
setLocalValue(value);
}
// eslint-disable-next-line
}, [ value ]);
const onChangeWithPrev = onChange ? (newVal?: string) => {
setPrevValue(newVal);
onChange(newVal);
} : undefined;
const debouncedOnChange = useDebounce(onChangeWithPrev, props.delay || 200);
const onChangeWithLocal = debouncedOnChange ? (e: React.FormEvent) => {
const newVal = e.currentTarget.value;
setLocalValue(newVal);
debouncedOnChange(newVal);
}: undefined;
const isInvalid = isValid !== undefined ? !isValid : false
return (
);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy