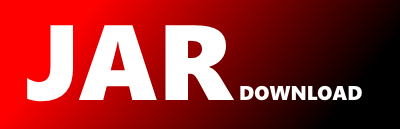
io.undertow.servlet.handlers.ServletDebugPageHandler Maven / Gradle / Ivy
The newest version!
/*
* JBoss, Home of Professional Open Source.
* Copyright 2014 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.undertow.servlet.handlers;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.nio.charset.StandardCharsets;
import jakarta.servlet.ServletOutputStream;
import io.undertow.server.HttpServerExchange;
import io.undertow.servlet.spec.HttpServletRequestImpl;
/**
* generates a servlet error page with a stack trace
*
* @author Stuart Douglas
*/
public class ServletDebugPageHandler {
public static final String ERROR_CSS =
"";
public static void handleRequest(HttpServerExchange exchange, final ServletRequestContext servletRequestContext, final Throwable exception) throws IOException {
HttpServletRequestImpl req = servletRequestContext.getOriginalRequest();
StringBuilder sb = new StringBuilder();
//todo: make this good
sb.append("ERROR ");
sb.append(ERROR_CSS);
sb.append("Error processing request");
writeLabel(sb, "Context Path", req.getContextPath());
writeLabel(sb, "Servlet Path", req.getServletPath());
writeLabel(sb, "Path Info", req.getPathInfo());
writeLabel(sb, "Query String", req.getQueryString());
writeLabel(sb, "Stack Trace", "");
sb.append("");
StringWriter stringWriter = new StringWriter();
exception.printStackTrace(new PrintWriter(stringWriter));
sb.append(escapeBodyText(stringWriter.toString()));
sb.append("
");
servletRequestContext.getOriginalResponse().setContentType("text/html");
servletRequestContext.getOriginalResponse().setCharacterEncoding("UTF-8");
try {
ServletOutputStream out = servletRequestContext.getOriginalResponse().getOutputStream();
out.write(sb.toString().getBytes(StandardCharsets.UTF_8));
out.close();
} catch (IllegalStateException e) {
PrintWriter writer = servletRequestContext.getOriginalResponse().getWriter();
writer.write(sb.toString());
writer.close();
}
}
private static void writeLabel(StringBuilder sb, String label, String value) {
sb.append("");
sb.append(escapeBodyText(label));
sb.append(":");
sb.append(escapeBodyText(value));
sb.append("
");
}
public static String escapeBodyText(final String bodyText) {
if(bodyText == null) {
return "null";
}
return bodyText.replace("&", "&").replace("<", "<").replace(">", ">");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy