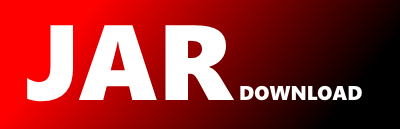
io.undertow.websockets.jsr.annotated.AnnotatedEndpoint Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2014 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.undertow.websockets.jsr.annotated;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.HashMap;
import java.util.Map;
import javax.websocket.CloseReason;
import javax.websocket.Endpoint;
import javax.websocket.EndpointConfig;
import javax.websocket.MessageHandler;
import javax.websocket.SendHandler;
import javax.websocket.SendResult;
import javax.websocket.Session;
import io.undertow.UndertowLogger;
import io.undertow.servlet.api.InstanceHandle;
import io.undertow.websockets.jsr.JsrWebSocketLogger;
import io.undertow.websockets.jsr.UndertowSession;
/**
* @author Stuart Douglas
*/
public class AnnotatedEndpoint extends Endpoint {
private final InstanceHandle> instance;
private final BoundMethod webSocketOpen;
private final BoundMethod webSocketClose;
private final BoundMethod webSocketError;
private final BoundMethod textMessage;
private final BoundMethod binaryMessage;
private final BoundMethod pongMessage;
private volatile boolean released;
AnnotatedEndpoint(final InstanceHandle> instance, final BoundMethod webSocketOpen, final BoundMethod webSocketClose, final BoundMethod webSocketError, final BoundMethod textMessage, final BoundMethod binaryMessage, final BoundMethod pongMessage) {
this.instance = instance;
this.webSocketOpen = webSocketOpen;
this.webSocketClose = webSocketClose;
this.webSocketError = webSocketError;
this.textMessage = textMessage;
this.binaryMessage = binaryMessage;
this.pongMessage = pongMessage;
}
@Override
public void onOpen(final Session session, final EndpointConfig endpointConfiguration) {
this.released = false;
final UndertowSession s = (UndertowSession) session;
boolean partialText = textMessage == null || (textMessage.hasParameterType(boolean.class) && !textMessage.getMessageType().equals(boolean.class));
boolean partialBinary = binaryMessage == null || (binaryMessage.hasParameterType(boolean.class) && !binaryMessage.getMessageType().equals(boolean.class));
if (textMessage != null) {
if (partialText) {
addPartialHandler(s, textMessage);
} else {
if (textMessage.getMaxMessageSize() > 0) {
s.setMaxTextMessageBufferSize((int) textMessage.getMaxMessageSize());
}
addWholeHandler(s, textMessage);
}
}
if (binaryMessage != null) {
if (partialBinary) {
addPartialHandler(s, binaryMessage);
} else {
if (binaryMessage.getMaxMessageSize() > 0) {
s.setMaxBinaryMessageBufferSize((int) binaryMessage.getMaxMessageSize());
}
addWholeHandler(s, binaryMessage);
}
}
if (pongMessage != null) {
addWholeHandler(s, pongMessage);
}
if (webSocketOpen != null) {
final Map, Object> params = new HashMap<>();
params.put(Session.class, session);
params.put(EndpointConfig.class, endpointConfiguration);
params.put(Map.class, session.getPathParameters());
invokeMethod(params, webSocketOpen, s);
}
}
private void addPartialHandler(final UndertowSession session, final BoundMethod method) {
session.addMessageHandler((Class) method.getMessageType(), new MessageHandler.Partial
© 2015 - 2025 Weber Informatics LLC | Privacy Policy