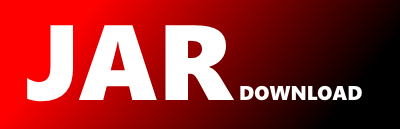
io.undertow.websockets.jsr.WebSocketDeploymentInfo Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2014 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.undertow.websockets.jsr;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.Executor;
import java.util.function.Supplier;
import javax.websocket.server.ServerEndpointConfig;
import io.netty.channel.EventLoopGroup;
import io.netty.handler.codec.http.websocketx.extensions.WebSocketServerExtensionHandshaker;
/**
* Web socket deployment information
*
* @author Stuart Douglas
*/
public class WebSocketDeploymentInfo implements Cloneable {
public static final String ATTRIBUTE_NAME = "io.undertow.websockets.jsr.WebSocketDeploymentInfo";
private boolean dispatchToWorkerThread = false;
private final List> annotatedEndpoints = new ArrayList<>();
private final List programaticEndpoints = new ArrayList<>();
private final List containerReadyListeners = new ArrayList<>();
private final List serverExtensions = new ArrayList<>();
private String clientBindAddress = null;
private WebSocketReconnectHandler reconnectHandler;
private EventLoopGroup eventLoopGroup;
private Supplier executor;
private int maxFrameSize = ServerWebSocketContainer.DEFAULT_MAX_FRAME_SIZE;
public WebSocketDeploymentInfo addEndpoint(final Class> annotated) {
this.annotatedEndpoints.add(annotated);
return this;
}
public WebSocketDeploymentInfo addAnnotatedEndpoints(final Collection> annotatedEndpoints) {
this.annotatedEndpoints.addAll(annotatedEndpoints);
return this;
}
public WebSocketDeploymentInfo addEndpoint(final ServerEndpointConfig endpoint) {
this.programaticEndpoints.add(endpoint);
return this;
}
public WebSocketDeploymentInfo addProgramaticEndpoints(final Collection programaticEndpoints) {
this.programaticEndpoints.addAll(programaticEndpoints);
return this;
}
public EventLoopGroup getEventLoopGroup() {
return eventLoopGroup;
}
public Supplier getExecutor() {
return executor;
}
public WebSocketDeploymentInfo setExecutor(Supplier executor) {
this.executor = executor;
return this;
}
public WebSocketDeploymentInfo setEventLoopGroup(EventLoopGroup eventLoopGroup) {
this.eventLoopGroup = eventLoopGroup;
return this;
}
public List> getAnnotatedEndpoints() {
return annotatedEndpoints;
}
public List getProgramaticEndpoints() {
return programaticEndpoints;
}
void containerReady(ServerWebSocketContainer container) {
for (ContainerReadyListener listener : containerReadyListeners) {
listener.ready(container);
}
}
public WebSocketDeploymentInfo addListener(final ContainerReadyListener listener) {
containerReadyListeners.add(listener);
return this;
}
public WebSocketDeploymentInfo addListeners(final Collection listeners) {
containerReadyListeners.addAll(listeners);
return this;
}
public List getListeners() {
return containerReadyListeners;
}
public boolean isDispatchToWorkerThread() {
return dispatchToWorkerThread;
}
public WebSocketDeploymentInfo setDispatchToWorkerThread(boolean dispatchToWorkerThread) {
this.dispatchToWorkerThread = dispatchToWorkerThread;
return this;
}
public int getMaxFrameSize() {
return maxFrameSize;
}
public WebSocketDeploymentInfo setMaxFrameSize(int maxFrameSize) {
this.maxFrameSize = maxFrameSize;
return this;
}
public interface ContainerReadyListener {
void ready(ServerWebSocketContainer container);
}
/**
* Add a new WebSocket Extension into this deployment info.
*
* @param extension a new {@code ExtensionHandshake} instance
* @return current deployment info
*/
public WebSocketDeploymentInfo addServerExtension(final WebSocketServerExtensionHandshaker extension) {
if (null != extension) {
this.serverExtensions.add(extension);
}
return this;
}
public WebSocketDeploymentInfo addServerExtensions(final Collection extensions) {
this.serverExtensions.addAll(extensions);
return this;
}
/**
* @return list of extensions available for this deployment info
*/
public List getServerExtensions() {
return serverExtensions;
}
public String getClientBindAddress() {
return clientBindAddress;
}
public WebSocketDeploymentInfo setClientBindAddress(String clientBindAddress) {
this.clientBindAddress = clientBindAddress;
return this;
}
public WebSocketReconnectHandler getReconnectHandler() {
return reconnectHandler;
}
public WebSocketDeploymentInfo setReconnectHandler(WebSocketReconnectHandler reconnectHandler) {
this.reconnectHandler = reconnectHandler;
return this;
}
@Override
public WebSocketDeploymentInfo clone() {
return new WebSocketDeploymentInfo()
.setDispatchToWorkerThread(this.dispatchToWorkerThread)
.addAnnotatedEndpoints(this.annotatedEndpoints)
.addProgramaticEndpoints(this.programaticEndpoints)
.addListeners(this.containerReadyListeners)
.addServerExtensions(this.serverExtensions)
.setClientBindAddress(this.clientBindAddress)
.setReconnectHandler(this.reconnectHandler)
.setMaxFrameSize(this.maxFrameSize);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy