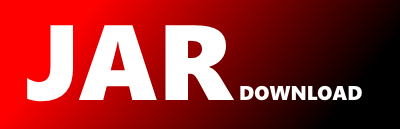
io.quarkus.test.utils.PropertiesUtils Maven / Gradle / Ivy
package io.quarkus.test.utils;
import static org.junit.jupiter.api.Assertions.fail;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Collections;
import java.util.Map;
import java.util.Properties;
import org.apache.commons.lang3.StringUtils;
import org.junit.jupiter.api.condition.OS;
public final class PropertiesUtils {
public static final String DESTINATION_TO_FILENAME_SEPARATOR = "|";
public static final String RESOURCE_PREFIX = "resource::/";
public static final String RESOURCE_WITH_DESTINATION_PREFIX = "resource_with_destination::";
public static final String SECRET_WITH_DESTINATION_PREFIX = "secret_with_destination::";
public static final String RESOURCE_WITH_DESTINATION_SPLIT_CHAR = "\\|";
public static final String RESOURCE_WITH_DESTINATION_PREFIX_MATCHER = ".*" + RESOURCE_WITH_DESTINATION_SPLIT_CHAR + ".*";
public static final String SECRET_PREFIX = "secret::/";
public static final Path TARGET = Path.of("target");
public static final String SLASH = System.getProperty("file.separator");
private static final String AUTOGENERATED_COMMENT = "# This properties file has been auto generated "
+ "by Quarkus QE Test Framework";
private static final String PROPERTY_START_TAG = "${";
private static final String PROPERTY_END_TAG = "}";
private static final String PROPERTY_WITH_OPTIONAL = ":";
private PropertiesUtils() {
}
/**
* Try to resolve the value property from the value if the content is contained between ${xxx}.
*
* @param value
* @return
*/
public static String resolveProperty(String value) {
if (StringUtils.startsWith(value, PROPERTY_START_TAG)) {
String propertyKey = StringUtils.substringBetween(value, PROPERTY_START_TAG, PROPERTY_END_TAG);
String defaultValue = StringUtils.EMPTY;
int optionalIndex = propertyKey.indexOf(PROPERTY_WITH_OPTIONAL);
if (optionalIndex > 0) {
defaultValue = propertyKey.substring(optionalIndex + 1);
propertyKey = propertyKey.substring(0, optionalIndex);
}
return System.getProperty(propertyKey, defaultValue);
}
return value;
}
public static Map toMap(String propertiesFile) {
try (InputStream in = ClassLoader.getSystemResourceAsStream(propertiesFile)) {
return toMap(in);
} catch (IOException e) {
fail("Could not load map from system resource. Caused by " + e);
}
return Collections.emptyMap();
}
public static Map toMap(Path path) {
try (InputStream in = Files.newInputStream(path)) {
return toMap(in);
} catch (IOException e) {
fail("Could not load map from path. Caused by " + e);
}
return Collections.emptyMap();
}
public static Map toMap(InputStream is) {
Properties properties = new Properties();
try {
properties.load(is);
} catch (IOException e) {
fail("Could not load map. Caused by " + e);
}
return (Map) properties;
}
public static void fromMap(Map map, Path target) {
Properties properties = new Properties();
properties.putAll(map);
try (PrintWriter pw = new PrintWriter(target.toFile())) {
pw.println(AUTOGENERATED_COMMENT);
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy