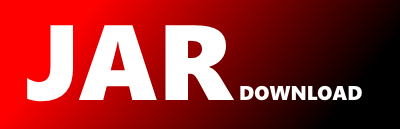
org.jboss.resteasy.reactive.common.model.ResourceClass Maven / Gradle / Ivy
package org.jboss.resteasy.reactive.common.model;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
import jakarta.ws.rs.ext.ExceptionMapper;
import org.jboss.resteasy.reactive.common.core.LazyUnmanagedBeanFactory;
import org.jboss.resteasy.reactive.common.util.URLUtils;
import org.jboss.resteasy.reactive.spi.BeanFactory;
public class ResourceClass {
/**
* The class name of the resource class
*/
private String className;
/**
* The class path, will be null if this is a sub resource
*/
private String path;
/**
* The resource methods
*/
private final List methods = new ArrayList<>();
private BeanFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy