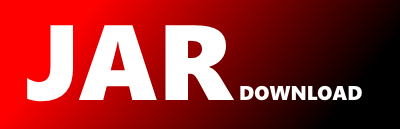
org.jboss.resteasy.reactive.server.mapping.RuntimeResource Maven / Gradle / Ivy
package org.jboss.resteasy.reactive.server.mapping;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
import jakarta.ws.rs.core.MediaType;
import org.jboss.resteasy.reactive.common.model.ResourceExceptionMapper;
import org.jboss.resteasy.reactive.common.util.ServerMediaType;
import org.jboss.resteasy.reactive.server.SimpleResourceInfo;
import org.jboss.resteasy.reactive.server.core.ResteasyReactiveSimplifiedResourceInfo;
import org.jboss.resteasy.reactive.server.spi.EndpointInvoker;
import org.jboss.resteasy.reactive.server.spi.ResteasyReactiveResourceInfo;
import org.jboss.resteasy.reactive.server.spi.ServerRestHandler;
import org.jboss.resteasy.reactive.server.util.ScoreSystem;
import org.jboss.resteasy.reactive.spi.BeanFactory;
public class RuntimeResource {
private final String httpMethod;
private final URITemplate path;
private final URITemplate classPath;
private final ServerMediaType produces;
private final List consumes;
private final EndpointInvoker invoker;
private final BeanFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy