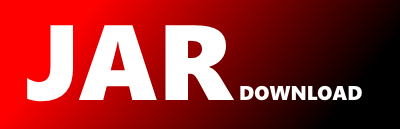
io.quarkus.cache.CacheName Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-cache Show documentation
Show all versions of quarkus-cache Show documentation
Enable application data caching in CDI beans
package io.quarkus.cache;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.ElementType.PARAMETER;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import javax.enterprise.util.Nonbinding;
import javax.inject.Qualifier;
/**
*
* Use this annotation on a field, a constructor parameter or a method parameter to inject a {@link Cache} and interact with it
* programmatically e.g. store, retrieve or delete cache values.
*
*
* Field injection example:
*
*
* {@literal @}ApplicationScoped
* public class CachedService {
*
* {@literal @}CacheName("my-cache")
* Cache cache;
*
* String getExpensiveValue(Object key) {
* {@code Uni} cacheValue = cache.get(key, () -> expensiveService.getValue(key));
* return cacheValue.await().indefinitely();
* }
* }
*
*
* Constructor parameter injection example:
*
*
* {@literal @}ApplicationScoped
* public class CachedService {
*
* private Cache cache;
*
* public CachedService(@CacheName("my-cache") Cache cache) {
* this.cache = cache;
* }
*
* String getExpensiveValue(Object key) {
* {@code Uni} cacheValue = cache.get(key, () -> expensiveService.getValue(key));
* return cacheValue.await().indefinitely();
* }
* }
*
*
* Method parameter injection example:
*
*
* {@literal @}ApplicationScoped
* public class CachedService {
*
* private Cache cache;
*
* {@literal @}Inject
* public void setCache(@CacheName("my-cache") Cache cache) {
* this.cache = cache;
* }
*
* String getExpensiveValue(Object key) {
* {@code Uni} cacheValue = cache.get(key, () -> expensiveService.getValue(key));
* return cacheValue.await().indefinitely();
* }
* }
*
*
*
* @see CacheManager
*/
@Qualifier
@Target({ FIELD, METHOD, PARAMETER })
@Retention(RUNTIME)
public @interface CacheName {
/**
* The name of the cache.
*/
@Nonbinding
String value();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy