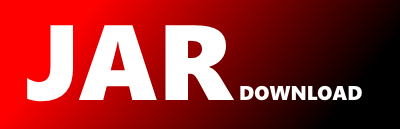
io.quarkus.cache.runtime.caffeine.CaffeineCacheImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-cache Show documentation
Show all versions of quarkus-cache Show documentation
Enable application data caching in CDI beans
package io.quarkus.cache.runtime.caffeine;
import java.time.Duration;
import java.util.Collections;
import java.util.HashSet;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import org.jboss.logging.Logger;
import com.github.benmanes.caffeine.cache.AsyncCache;
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Policy;
import com.github.benmanes.caffeine.cache.Policy.FixedExpiration;
import com.github.benmanes.caffeine.cache.stats.ConcurrentStatsCounter;
import com.github.benmanes.caffeine.cache.stats.StatsCounter;
import io.quarkus.cache.CacheException;
import io.quarkus.cache.CaffeineCache;
import io.quarkus.cache.runtime.AbstractCache;
import io.quarkus.cache.runtime.NullValueConverter;
import io.smallrye.mutiny.Uni;
/**
* This class is an internal Quarkus cache implementation using Caffeine. Do not use it explicitly from your Quarkus
* application.
* The public methods signatures may change without prior notice.
*/
public class CaffeineCacheImpl extends AbstractCache implements CaffeineCache {
private static final Logger LOGGER = Logger.getLogger(CaffeineCacheImpl.class);
final AsyncCache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy