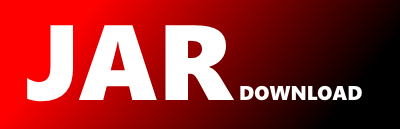
io.quarkus.logging.Log Maven / Gradle / Ivy
package io.quarkus.logging;
import org.jboss.logging.Logger;
import io.quarkus.runtime.Application;
// automatically generated by io.quarkus.logging.GenerateLog
/**
* Copy of {@link org.jboss.logging.BasicLogger}.
* Invocations of all {@code static} methods of this class are, during build time, replaced by invocations
* of the same methods on a generated instance of {@link Logger}.
*/
public final class Log {
private static final StackWalker stackWalker = StackWalker.getInstance(StackWalker.Option.RETAIN_CLASS_REFERENCE);
private static final boolean shouldFail = shouldFail();
private static boolean shouldFail() {
// inside Quarkus, all call sites should be rewritten
if (Application.currentApplication() != null)
return true;
// outside Quarkus, allow in tests
try {
Class.forName("org.junit.jupiter.api.Assertions");
return false;
} catch (java.lang.ClassNotFoundException ignored) {
return true;
}
}
/**
* Check to see if the given level is enabled for this logger.
*
* @param level the level to check for
* @return {@code true} if messages may be logged at the given level, {@code false} otherwise
*/
public static boolean isEnabled(Logger.Level level) {
if (shouldFail) {
throw fail();
}
return Logger.getLogger(stackWalker.getCallerClass()).isEnabled(level);
}
/**
* Check to see if the {@code TRACE} level is enabled for this logger.
*
* @return {@code true} if messages logged at {@link org.jboss.logging.Logger.Level#TRACE} may be accepted, {@code false}
* otherwise
*/
public static boolean isTraceEnabled() {
if (shouldFail) {
throw fail();
}
return Logger.getLogger(stackWalker.getCallerClass()).isTraceEnabled();
}
/**
* Issue a log message with a level of TRACE.
*
* @param message the message
*/
public static void trace(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).trace(message);
}
/**
* Issue a log message and throwable with a level of TRACE.
*
* @param message the message
* @param t the throwable
*/
public static void trace(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).trace(message, t);
}
/**
* Issue a log message and throwable with a level of TRACE and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void trace(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).trace(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of TRACE.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void trace(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).trace(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void tracev(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(format, params);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void tracev(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(format, param1);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void tracev(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(format, param1, param2);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void tracev(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(format, param1, param2, param3);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void tracev(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(t, format, params);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void tracev(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(t, format, param1);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void tracev(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(t, format, param1, param2);
}
/**
* Issue a log message with a level of TRACE using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void tracev(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracev(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void tracef(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, params);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void tracef(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, param1);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void tracef(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, param1, param2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void tracef(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void tracef(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, params);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void tracef(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, param1);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void tracef(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void tracef(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void tracef(String format, int arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(String format, int arg1, int arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(String format, int arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, int arg1, int arg2, int arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, int arg1, int arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, int arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void tracef(Throwable t, String format, int arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(Throwable t, String format, int arg1, int arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(Throwable t, String format, int arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, int arg1, int arg2, int arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, int arg1, int arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, int arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void tracef(String format, long arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(String format, long arg1, long arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(String format, long arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, long arg1, long arg2, long arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, long arg1, long arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(String format, long arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void tracef(Throwable t, String format, long arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(Throwable t, String format, long arg1, long arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void tracef(Throwable t, String format, long arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, long arg1, long arg2, long arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, long arg1, long arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of TRACE.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void tracef(Throwable t, String format, long arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).tracef(t, format, arg1, arg2, arg3);
}
/**
* Check to see if the {@code DEBUG} level is enabled for this logger.
*
* @return {@code true} if messages logged at {@link org.jboss.logging.Logger.Level#DEBUG} may be accepted, {@code false}
* otherwise
*/
public static boolean isDebugEnabled() {
if (shouldFail) {
throw fail();
}
return Logger.getLogger(stackWalker.getCallerClass()).isDebugEnabled();
}
/**
* Issue a log message with a level of DEBUG.
*
* @param message the message
*/
public static void debug(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debug(message);
}
/**
* Issue a log message and throwable with a level of DEBUG.
*
* @param message the message
* @param t the throwable
*/
public static void debug(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debug(message, t);
}
/**
* Issue a log message and throwable with a level of DEBUG and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void debug(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debug(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of DEBUG.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void debug(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debug(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void debugv(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(format, params);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void debugv(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(format, param1);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void debugv(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(format, param1, param2);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void debugv(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(format, param1, param2, param3);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void debugv(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(t, format, params);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void debugv(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(t, format, param1);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void debugv(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(t, format, param1, param2);
}
/**
* Issue a log message with a level of DEBUG using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void debugv(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugv(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void debugf(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, params);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void debugf(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, param1);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void debugf(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, param1, param2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void debugf(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void debugf(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, params);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void debugf(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, param1);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void debugf(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void debugf(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void debugf(String format, int arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(String format, int arg1, int arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(String format, int arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, int arg1, int arg2, int arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, int arg1, int arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, int arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void debugf(Throwable t, String format, int arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(Throwable t, String format, int arg1, int arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(Throwable t, String format, int arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, int arg1, int arg2, int arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, int arg1, int arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, int arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void debugf(String format, long arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(String format, long arg1, long arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(String format, long arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, long arg1, long arg2, long arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, long arg1, long arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(String format, long arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg the parameter
*/
public static void debugf(Throwable t, String format, long arg) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(Throwable t, String format, long arg1, long arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
*/
public static void debugf(Throwable t, String format, long arg1, Object arg2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, long arg1, long arg2, long arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, long arg1, long arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Issue a formatted log message with a level of DEBUG.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param arg1 the first parameter
* @param arg2 the second parameter
* @param arg3 the third parameter
*/
public static void debugf(Throwable t, String format, long arg1, Object arg2, Object arg3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).debugf(t, format, arg1, arg2, arg3);
}
/**
* Check to see if the {@code INFO} level is enabled for this logger.
*
* @return {@code true} if messages logged at {@link org.jboss.logging.Logger.Level#INFO} may be accepted, {@code false}
* otherwise
*/
public static boolean isInfoEnabled() {
if (shouldFail) {
throw fail();
}
return Logger.getLogger(stackWalker.getCallerClass()).isInfoEnabled();
}
/**
* Issue a log message with a level of INFO.
*
* @param message the message
*/
public static void info(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).info(message);
}
/**
* Issue a log message and throwable with a level of INFO.
*
* @param message the message
* @param t the throwable
*/
public static void info(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).info(message, t);
}
/**
* Issue a log message and throwable with a level of INFO and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void info(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).info(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of INFO.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void info(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).info(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void infov(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(format, params);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void infov(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(format, param1);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void infov(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(format, param1, param2);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void infov(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(format, param1, param2, param3);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void infov(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(t, format, params);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void infov(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(t, format, param1);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void infov(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(t, format, param1, param2);
}
/**
* Issue a log message with a level of INFO using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void infov(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infov(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void infof(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(format, params);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void infof(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(format, param1);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void infof(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(format, param1, param2);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void infof(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void infof(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(t, format, params);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void infof(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(t, format, param1);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void infof(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of INFO.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void infof(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).infof(t, format, param1, param2, param3);
}
/**
* Issue a log message with a level of WARN.
*
* @param message the message
*/
public static void warn(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warn(message);
}
/**
* Issue a log message and throwable with a level of WARN.
*
* @param message the message
* @param t the throwable
*/
public static void warn(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warn(message, t);
}
/**
* Issue a log message and throwable with a level of WARN and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void warn(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warn(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of WARN.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void warn(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warn(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void warnv(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(format, params);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void warnv(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(format, param1);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void warnv(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(format, param1, param2);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void warnv(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(format, param1, param2, param3);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void warnv(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(t, format, params);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void warnv(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(t, format, param1);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void warnv(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(t, format, param1, param2);
}
/**
* Issue a log message with a level of WARN using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void warnv(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnv(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void warnf(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(format, params);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void warnf(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(format, param1);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void warnf(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(format, param1, param2);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void warnf(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void warnf(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(t, format, params);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void warnf(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(t, format, param1);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void warnf(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of WARN.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void warnf(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).warnf(t, format, param1, param2, param3);
}
/**
* Issue a log message with a level of ERROR.
*
* @param message the message
*/
public static void error(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).error(message);
}
/**
* Issue a log message and throwable with a level of ERROR.
*
* @param message the message
* @param t the throwable
*/
public static void error(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).error(message, t);
}
/**
* Issue a log message and throwable with a level of ERROR and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void error(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).error(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of ERROR.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void error(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).error(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void errorv(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(format, params);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void errorv(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(format, param1);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void errorv(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(format, param1, param2);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void errorv(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(format, param1, param2, param3);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void errorv(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(t, format, params);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void errorv(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(t, format, param1);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void errorv(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(t, format, param1, param2);
}
/**
* Issue a log message with a level of ERROR using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void errorv(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorv(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void errorf(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(format, params);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void errorf(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(format, param1);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void errorf(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(format, param1, param2);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void errorf(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void errorf(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(t, format, params);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void errorf(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(t, format, param1);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void errorf(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of ERROR.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void errorf(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).errorf(t, format, param1, param2, param3);
}
/**
* Issue a log message with a level of FATAL.
*
* @param message the message
*/
public static void fatal(Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatal(message);
}
/**
* Issue a log message and throwable with a level of FATAL.
*
* @param message the message
* @param t the throwable
*/
public static void fatal(Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatal(message, t);
}
/**
* Issue a log message and throwable with a level of FATAL and a specific logger class name.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void fatal(String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatal(loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable with a level of FATAL.
*
* @param loggerFqcn the logger class name
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void fatal(String loggerFqcn, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatal(loggerFqcn, message, params, t);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param params the parameters
*/
public static void fatalv(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(format, params);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the sole parameter
*/
public static void fatalv(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(format, param1);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void fatalv(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(format, param1, param2);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void fatalv(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(format, param1, param2, param3);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void fatalv(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(t, format, params);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void fatalv(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(t, format, param1);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void fatalv(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(t, format, param1, param2);
}
/**
* Issue a log message with a level of FATAL using {@link java.text.MessageFormat}-style formatting.
*
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void fatalv(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalv(t, format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void fatalf(String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(format, params);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void fatalf(String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(format, param1);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void fatalf(String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(format, param1, param2);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void fatalf(String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(format, param1, param2, param3);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void fatalf(Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(t, format, params);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void fatalf(Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(t, format, param1);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void fatalf(Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(t, format, param1, param2);
}
/**
* Issue a formatted log message with a level of FATAL.
*
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void fatalf(Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).fatalf(t, format, param1, param2, param3);
}
/**
* Log a message at the given level.
*
* @param level the level
* @param message the message
*/
public static void log(Logger.Level level, Object message) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).log(level, message);
}
/**
* Issue a log message and throwable at the given log level.
*
* @param level the level
* @param message the message
* @param t the throwable
*/
public static void log(Logger.Level level, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).log(level, message, t);
}
/**
* Issue a log message and throwable at the given log level and a specific logger class name.
*
* @param level the level
* @param loggerFqcn the logger class name
* @param message the message
* @param t the throwable
*/
public static void log(Logger.Level level, String loggerFqcn, Object message, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).log(level, loggerFqcn, message, t);
}
/**
* Issue a log message with parameters and a throwable at the given log level.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param message the message
* @param params the message parameters
* @param t the throwable
*/
public static void log(String loggerFqcn, Logger.Level level, Object message, Object[] params, Throwable t) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).log(loggerFqcn, level, message, params, t);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param format the message format string
* @param params the parameters
*/
public static void logv(Logger.Level level, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, format, params);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param format the message format string
* @param param1 the sole parameter
*/
public static void logv(Logger.Level level, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, format, param1);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logv(Logger.Level level, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, format, param1, param2);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logv(Logger.Level level, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, format, param1, param2, param3);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void logv(Logger.Level level, Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, t, format, params);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void logv(Logger.Level level, Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, t, format, param1);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logv(Logger.Level level, Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, t, format, param1, param2);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logv(Logger.Level level, Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(level, t, format, param1, param2, param3);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable
* @param format the message format string
* @param params the parameters
*/
public static void logv(String loggerFqcn, Logger.Level level, Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(loggerFqcn, level, t, format, params);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the sole parameter
*/
public static void logv(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(loggerFqcn, level, t, format, param1);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logv(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(loggerFqcn, level, t, format, param1, param2);
}
/**
* Issue a log message at the given log level using {@link java.text.MessageFormat}-style formatting.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable
* @param format the message format string
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logv(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1, Object param2,
Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logv(loggerFqcn, level, t, format, param1, param2, param3);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the parameters
*/
public static void logf(Logger.Level level, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, format, params);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void logf(Logger.Level level, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, format, param1);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logf(Logger.Level level, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, format, param1, param2);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logf(Logger.Level level, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, format, param1, param2, param3);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param params the parameters
*/
public static void logf(Logger.Level level, Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, t, format, params);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the sole parameter
*/
public static void logf(Logger.Level level, Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, t, format, param1);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logf(Logger.Level level, Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, t, format, param1, param2);
}
/**
* Issue a formatted log message at the given log level.
*
* @param level the level
* @param t the throwable
* @param format the format string, as per {@link String#format(String, Object...)}
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logf(Logger.Level level, Throwable t, String format, Object param1, Object param2, Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(level, t, format, param1, param2, param3);
}
/**
* Log a message at the given level.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable cause
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the sole parameter
*/
public static void logf(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(loggerFqcn, level, t, format, param1);
}
/**
* Log a message at the given level.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable cause
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
*/
public static void logf(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1, Object param2) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(loggerFqcn, level, t, format, param1, param2);
}
/**
* Log a message at the given level.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable cause
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param param1 the first parameter
* @param param2 the second parameter
* @param param3 the third parameter
*/
public static void logf(String loggerFqcn, Logger.Level level, Throwable t, String format, Object param1, Object param2,
Object param3) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(loggerFqcn, level, t, format, param1, param2, param3);
}
/**
* Log a message at the given level.
*
* @param loggerFqcn the logger class name
* @param level the level
* @param t the throwable cause
* @param format the format string as per {@link String#format(String, Object...)} or resource bundle key therefor
* @param params the message parameters
*/
public static void logf(String loggerFqcn, Logger.Level level, Throwable t, String format, Object... params) {
if (shouldFail) {
throw fail();
}
Logger.getLogger(stackWalker.getCallerClass()).logf(loggerFqcn, level, t, format, params);
}
private static UnsupportedOperationException fail() {
return new UnsupportedOperationException(
"Using io.quarkus.logging.Log is only possible with Quarkus bytecode transformation; make sure the archive is indexed, for example by including a beans.xml file");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy