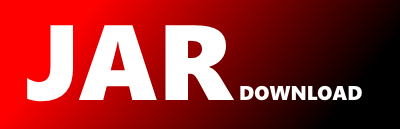
io.quarkus.runtime.ConfigConfig Maven / Gradle / Ivy
package io.quarkus.runtime;
import java.net.URI;
import java.util.List;
import java.util.Optional;
import io.quarkus.runtime.annotations.ConfigDocPrefix;
import io.quarkus.runtime.annotations.ConfigPhase;
import io.quarkus.runtime.annotations.ConfigRoot;
import io.smallrye.config.ConfigMapping;
import io.smallrye.config.WithName;
/**
* Configuration.
*
* We don't really use this, because these are configurations for the config itself, so it causes a chicken / egg
* problem, but we need it for documentation purposes.
*
* Relocation of the Config configurations to the Quarkus namespace is done in
* {@link io.quarkus.runtime.configuration.ConfigUtils#configBuilder}.
*/
@ConfigMapping(prefix = "quarkus")
@ConfigRoot(phase = ConfigPhase.RUN_TIME)
@ConfigDocPrefix("quarkus.config")
public interface ConfigConfig {
/**
* A comma separated list of profiles that will be active when Quarkus launches.
*/
Optional> profile();
/**
* Accepts a single configuration profile name. If a configuration property cannot be found in the current active
* profile, the config performs the same lookup in the profile set by this configuration.
*/
@WithName("config.profile.parent")
Optional profileParent();
/**
* Additional config locations to be loaded with the Config. The configuration support multiple locations
* separated by a comma and each must represent a valid {@link java.net.URI}.
*/
@WithName("config.locations")
Optional> locations();
/**
* Validates that a @ConfigMapping
maps every available configuration name contained in the mapping
* prefix.
*/
@WithName("config.mapping.validate-unknown")
Optional mappingValidateUnknown();
/**
* Enable logging of configuration values lookup in DEBUG log level.
*
* The log of configuration values require the category set to DEBUG
in the
* io.smallrye.config
category: quarkus.log.category."io.smallrye.config".level=DEBUG
.
*/
@WithName("config.log.values")
Optional logValues();
/**
* A property that allows accessing a generated UUID.
* It generates that UUID at startup time. So it changes between two starts including in dev mode.
*
* Access this generated UUID using expressions: `${quarkus.uuid}`.
*/
Optional uuid();
}