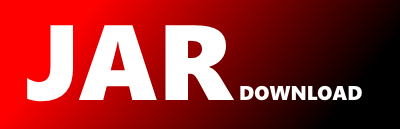
io.quarkus.infinispan.client.runtime.cache.InfinispanGetWrapper Maven / Gradle / Ivy
package io.quarkus.infinispan.client.runtime.cache;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.function.Function;
import java.util.function.Supplier;
import org.infinispan.client.hotrod.RemoteCache;
import org.infinispan.commons.CacheException;
import io.smallrye.mutiny.Uni;
public class InfinispanGetWrapper {
final RemoteCache cache;
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy