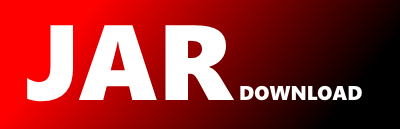
io.quarkus.oidc.OidcTenantConfig$$accessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-oidc Show documentation
Show all versions of quarkus-oidc Show documentation
Secure your applications with OpenID Connect Adapter and IDP such as Keycloak
package io.quarkus.oidc;
public final class OidcTenantConfig$$accessor {
private OidcTenantConfig$$accessor() {}
@SuppressWarnings("unchecked")
public static Object get_tenantId(Object __instance) {
return ((OidcTenantConfig)__instance).tenantId;
}
@SuppressWarnings("unchecked")
public static void set_tenantId(Object __instance, Object tenantId) {
((OidcTenantConfig)__instance).tenantId = (java.util.Optional)tenantId;
}
@SuppressWarnings("unchecked")
public static boolean get_tenantEnabled(Object __instance) {
return ((OidcTenantConfig)__instance).tenantEnabled;
}
@SuppressWarnings("unchecked")
public static void set_tenantEnabled(Object __instance, boolean tenantEnabled) {
((OidcTenantConfig)__instance).tenantEnabled = tenantEnabled;
}
@SuppressWarnings("unchecked")
public static Object get_applicationType(Object __instance) {
return ((OidcTenantConfig)__instance).applicationType;
}
@SuppressWarnings("unchecked")
public static void set_applicationType(Object __instance, Object applicationType) {
((OidcTenantConfig)__instance).applicationType = (io.quarkus.oidc.OidcTenantConfig.ApplicationType)applicationType;
}
@SuppressWarnings("unchecked")
public static Object get_connectionDelay(Object __instance) {
return ((OidcTenantConfig)__instance).connectionDelay;
}
@SuppressWarnings("unchecked")
public static void set_connectionDelay(Object __instance, Object connectionDelay) {
((OidcTenantConfig)__instance).connectionDelay = (java.util.Optional)connectionDelay;
}
@SuppressWarnings("unchecked")
public static Object get_authServerUrl(Object __instance) {
return ((OidcTenantConfig)__instance).authServerUrl;
}
@SuppressWarnings("unchecked")
public static void set_authServerUrl(Object __instance, Object authServerUrl) {
((OidcTenantConfig)__instance).authServerUrl = (java.util.Optional)authServerUrl;
}
@SuppressWarnings("unchecked")
public static Object get_introspectionPath(Object __instance) {
return ((OidcTenantConfig)__instance).introspectionPath;
}
@SuppressWarnings("unchecked")
public static void set_introspectionPath(Object __instance, Object introspectionPath) {
((OidcTenantConfig)__instance).introspectionPath = (java.util.Optional)introspectionPath;
}
@SuppressWarnings("unchecked")
public static Object get_jwksPath(Object __instance) {
return ((OidcTenantConfig)__instance).jwksPath;
}
@SuppressWarnings("unchecked")
public static void set_jwksPath(Object __instance, Object jwksPath) {
((OidcTenantConfig)__instance).jwksPath = (java.util.Optional)jwksPath;
}
@SuppressWarnings("unchecked")
public static Object get_endSessionPath(Object __instance) {
return ((OidcTenantConfig)__instance).endSessionPath;
}
@SuppressWarnings("unchecked")
public static void set_endSessionPath(Object __instance, Object endSessionPath) {
((OidcTenantConfig)__instance).endSessionPath = (java.util.Optional)endSessionPath;
}
@SuppressWarnings("unchecked")
public static Object get_publicKey(Object __instance) {
return ((OidcTenantConfig)__instance).publicKey;
}
@SuppressWarnings("unchecked")
public static void set_publicKey(Object __instance, Object publicKey) {
((OidcTenantConfig)__instance).publicKey = (java.util.Optional)publicKey;
}
@SuppressWarnings("unchecked")
public static Object get_clientId(Object __instance) {
return ((OidcTenantConfig)__instance).clientId;
}
@SuppressWarnings("unchecked")
public static void set_clientId(Object __instance, Object clientId) {
((OidcTenantConfig)__instance).clientId = (java.util.Optional)clientId;
}
@SuppressWarnings("unchecked")
public static Object get_roles(Object __instance) {
return ((OidcTenantConfig)__instance).roles;
}
@SuppressWarnings("unchecked")
public static void set_roles(Object __instance, Object roles) {
((OidcTenantConfig)__instance).roles = (io.quarkus.oidc.OidcTenantConfig.Roles)roles;
}
@SuppressWarnings("unchecked")
public static Object get_token(Object __instance) {
return ((OidcTenantConfig)__instance).token;
}
@SuppressWarnings("unchecked")
public static void set_token(Object __instance, Object token) {
((OidcTenantConfig)__instance).token = (io.quarkus.oidc.OidcTenantConfig.Token)token;
}
@SuppressWarnings("unchecked")
public static Object get_credentials(Object __instance) {
return ((OidcTenantConfig)__instance).credentials;
}
@SuppressWarnings("unchecked")
public static void set_credentials(Object __instance, Object credentials) {
((OidcTenantConfig)__instance).credentials = (io.quarkus.oidc.OidcTenantConfig.Credentials)credentials;
}
@SuppressWarnings("unchecked")
public static Object get_proxy(Object __instance) {
return ((OidcTenantConfig)__instance).proxy;
}
@SuppressWarnings("unchecked")
public static void set_proxy(Object __instance, Object proxy) {
((OidcTenantConfig)__instance).proxy = (io.quarkus.oidc.OidcTenantConfig.Proxy)proxy;
}
@SuppressWarnings("unchecked")
public static Object get_authentication(Object __instance) {
return ((OidcTenantConfig)__instance).authentication;
}
@SuppressWarnings("unchecked")
public static void set_authentication(Object __instance, Object authentication) {
((OidcTenantConfig)__instance).authentication = (io.quarkus.oidc.OidcTenantConfig.Authentication)authentication;
}
@SuppressWarnings("unchecked")
public static Object get_tls(Object __instance) {
return ((OidcTenantConfig)__instance).tls;
}
@SuppressWarnings("unchecked")
public static void set_tls(Object __instance, Object tls) {
((OidcTenantConfig)__instance).tls = (io.quarkus.oidc.OidcTenantConfig.Tls)tls;
}
@SuppressWarnings("unchecked")
public static Object get_logout(Object __instance) {
return ((OidcTenantConfig)__instance).logout;
}
@SuppressWarnings("unchecked")
public static void set_logout(Object __instance, Object logout) {
((OidcTenantConfig)__instance).logout = (io.quarkus.oidc.OidcTenantConfig.Logout)logout;
}
public static Object construct() {
return new OidcTenantConfig();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy