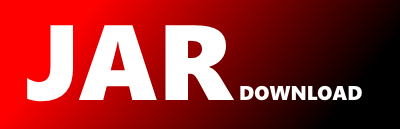
io.quarkus.picocli.runtime.DefaultPicocliCommandLineFactory Maven / Gradle / Ivy
package io.quarkus.picocli.runtime;
import jakarta.enterprise.context.ApplicationScoped;
import jakarta.enterprise.inject.Instance;
import jakarta.enterprise.inject.literal.NamedLiteral;
import io.quarkus.picocli.runtime.annotations.TopCommand;
import picocli.CommandLine;
@ApplicationScoped
public class DefaultPicocliCommandLineFactory implements PicocliCommandLineFactory {
private final Instance
© 2015 - 2025 Weber Informatics LLC | Privacy Policy