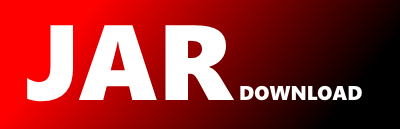
io.quarkus.picocli.runtime.PicocliRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-picocli Show documentation
Show all versions of quarkus-picocli Show documentation
Develop command line applications with Picocli
package io.quarkus.picocli.runtime;
import jakarta.enterprise.context.Dependent;
import jakarta.enterprise.event.Event;
import io.quarkus.runtime.QuarkusApplication;
import picocli.CommandLine;
@Dependent
public class PicocliRunner implements QuarkusApplication {
private static final class EventExecutionStrategy implements CommandLine.IExecutionStrategy {
private final CommandLine.IExecutionStrategy executionStrategy;
private final Event parseResultEvent;
private EventExecutionStrategy(CommandLine.IExecutionStrategy executionStrategy,
Event parseResultEvent) {
this.executionStrategy = executionStrategy;
this.parseResultEvent = parseResultEvent;
}
@Override
public int execute(CommandLine.ParseResult parseResult)
throws CommandLine.ExecutionException, CommandLine.ParameterException {
parseResultEvent.fire(parseResult);
return executionStrategy.execute(parseResult);
}
}
private final CommandLine commandLine;
public PicocliRunner(CommandLine commandLine, Event parseResultEvent) {
this.commandLine = commandLine
.setExecutionStrategy(new EventExecutionStrategy(commandLine.getExecutionStrategy(), parseResultEvent));
}
@Override
public int run(String... args) throws Exception {
try {
return commandLine.execute(args);
} finally {
commandLine.getOut().flush();
commandLine.getErr().flush();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy