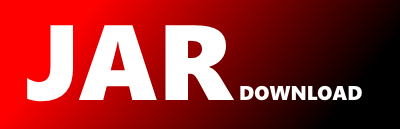
io.quarkus.qute.runtime.extensions.CollectionTemplateExtensions Maven / Gradle / Ivy
package io.quarkus.qute.runtime.extensions;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import javax.enterprise.inject.Vetoed;
import io.quarkus.qute.Results;
import io.quarkus.qute.TemplateExtension;
@Vetoed // Make sure no bean is created from this class
@TemplateExtension
public class CollectionTemplateExtensions {
static T get(List list, int index) {
return list.get(index);
}
@SuppressWarnings("unchecked")
@TemplateExtension(matchRegex = "\\d{1,10}")
static T getByIndex(List list, String index) {
int idx = Integer.parseInt(index);
if (idx >= list.size()) {
// Be consistent with property resolvers
return (T) Results.NotFound.from(index);
}
return list.get(idx);
}
static Iterator reversed(List list) {
ListIterator it = list.listIterator(list.size());
return new Iterator() {
@Override
public boolean hasNext() {
return it.hasPrevious();
}
@Override
public T next() {
return it.previous();
}
};
}
static List take(List list, int n) {
if (n < 1 || n > list.size()) {
throw new IndexOutOfBoundsException(n);
}
if (list.isEmpty()) {
return list;
}
return list.subList(0, n);
}
static List takeLast(List list, int n) {
if (n < 1 || n > list.size()) {
throw new IndexOutOfBoundsException(n);
}
if (list.isEmpty()) {
return list;
}
return list.subList(list.size() - n, list.size());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy