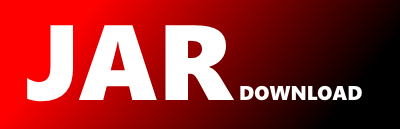
io.quarkus.redis.datasource.RedisDataSource Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource;
import static io.quarkus.redis.runtime.datasource.Marshaller.STRING_TYPE_REFERENCE;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import com.fasterxml.jackson.core.type.TypeReference;
import io.quarkus.redis.datasource.autosuggest.AutoSuggestCommands;
import io.quarkus.redis.datasource.bitmap.BitMapCommands;
import io.quarkus.redis.datasource.bloom.BloomCommands;
import io.quarkus.redis.datasource.countmin.CountMinCommands;
import io.quarkus.redis.datasource.cuckoo.CuckooCommands;
import io.quarkus.redis.datasource.geo.GeoCommands;
import io.quarkus.redis.datasource.graph.GraphCommands;
import io.quarkus.redis.datasource.hash.HashCommands;
import io.quarkus.redis.datasource.hyperloglog.HyperLogLogCommands;
import io.quarkus.redis.datasource.json.JsonCommands;
import io.quarkus.redis.datasource.keys.KeyCommands;
import io.quarkus.redis.datasource.list.ListCommands;
import io.quarkus.redis.datasource.pubsub.PubSubCommands;
import io.quarkus.redis.datasource.search.SearchCommands;
import io.quarkus.redis.datasource.set.SetCommands;
import io.quarkus.redis.datasource.sortedset.SortedSetCommands;
import io.quarkus.redis.datasource.stream.StreamCommands;
import io.quarkus.redis.datasource.string.StringCommands;
import io.quarkus.redis.datasource.timeseries.TimeSeriesCommands;
import io.quarkus.redis.datasource.topk.TopKCommands;
import io.quarkus.redis.datasource.transactions.OptimisticLockingTransactionResult;
import io.quarkus.redis.datasource.transactions.TransactionResult;
import io.quarkus.redis.datasource.transactions.TransactionalRedisDataSource;
import io.quarkus.redis.datasource.value.ValueCommands;
import io.smallrye.common.annotation.Experimental;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
/**
* Synchronous / Blocking Redis Data Source.
*
* This class provides access to various groups of methods. Each method execute a Redis {@code command}.
* Groups and methods are type-safe. The decomposition follows the Redis API group.
*
* NOTE: Not all commands are exposed from this API. This is done on purpose. You can always use the low-level Redis
* client to execute others commands.
*/
public interface RedisDataSource {
/**
* Retrieves a {@link RedisDataSource} using a single connection with the Redis server.
* The connection is acquired from the pool, and released then the consumer completes.
*
* @param consumer the consumer receiving the connection and returning when the connection can be released.
*/
void withConnection(Consumer consumer);
/**
* Retrieves a {@link RedisDataSource} enqueuing commands in a Redis Transaction ({@code MULTI}).
* Note that transaction acquires a single connection, and all the commands are enqueued in this connection.
* The commands are only executed when the passed block completes.
*
* The results of the commands are retrieved using the returned {@link TransactionResult}.
*
* The user can discard a transaction using the {@link TransactionalRedisDataSource#discard()} method.
* In this case, the produced {@link TransactionResult} will be empty.
*
* @param tx the consumer receiving the transactional redis data source. The enqueued commands are only executed
* at the end of the block.
*/
TransactionResult withTransaction(Consumer tx);
/**
* Retrieves a {@link RedisDataSource} enqueuing commands in a Redis Transaction ({@code MULTI}).
* Note that transaction acquires a single connection, and all the commands are enqueued in this connection.
* The commands are only executed when the passed block completes.
*
* The results of the commands are retrieved using the returned {@link TransactionResult}.
*
* The user can discard a transaction using the {@link TransactionalRedisDataSource#discard()} method.
* In this case, the produced {@link TransactionResult} will be empty.
*
* @param tx the consumer receiving the transactional redis data source. The enqueued commands are only executed
* at the end of the block.
* @param watchedKeys the keys to watch during the execution of the transaction. If one of these key is modified before
* the completion of the transaction, the transaction is discarded.
*/
TransactionResult withTransaction(Consumer tx, String... watchedKeys);
/**
* Retrieves a {@link RedisDataSource} enqueuing commands in a Redis Transaction ({@code MULTI}).
* Note that transaction acquires a single connection, and all the commands are enqueued in this connection.
* The commands are only executed when the passed block emits the {@code null} item.
*
* This variant also allows executing code before the transaction gets started but after the key being watched:
*
*
* WATCH key
* // preTxBlock
* element = ZRANGE k 0 0
* // TxBlock
* MULTI
* ZREM k element
* EXEC
*
*
* The {@code preTxBlock} returns a {@link I}. The produced value is received by the {@code tx} block,
* which can use that value to execute the appropriate operation in the transaction. The produced value can also be
* retrieved from the produced {@link OptimisticLockingTransactionResult}. Commands issued in the {@code preTxBlock }
* must used the passed (single-connection) {@link RedisDataSource} instance.
*
* If the {@code preTxBlock} throws an exception, the transaction is not executed, and the returned
* {@link OptimisticLockingTransactionResult} is empty.
*
* This construct allows implementing operation relying on optimistic locking.
* The results of the commands are retrieved using the produced {@link OptimisticLockingTransactionResult}.
*
* The user can discard a transaction using the {@link TransactionalRedisDataSource#discard()} method.
* In this case, the produced {@link OptimisticLockingTransactionResult} will be empty.
*
* @param tx the consumer receiving the transactional redis data source. The enqueued commands are only executed
* at the end of the block.
* @param watchedKeys the keys to watch during the execution of the transaction. If one of these key is modified before
* the completion of the transaction, the transaction is discarded.
*/
OptimisticLockingTransactionResult withTransaction(Function preTxBlock,
BiConsumer tx,
String... watchedKeys);
/**
* Execute the command SELECT.
* Summary: Change the selected database for the current connection
* Group: connection
* Requires Redis 1.0.0
*
* This method is expected to be used inside a {@link #withConnection(Consumer)} block.
*
* @param index the database index.
**/
void select(long index);
/**
* Execute the command FLUSHALL.
* Summary: Remove all keys from all databases
* Group: server
* Requires Redis 1.0.0
**/
void flushall();
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<F, V>}).
*
* If you want to use a hash of {@code <String -> Person>} stored using String identifier, you would use:
* {@code hash(String.class, String.class, Person.class)}.
* If you want to use a hash of {@code <String -> Person>} stored using UUID identifier, you would use:
* {@code hash(UUID.class, String.class, Person.class)}.
*
* @param redisKeyType the class of the keys
* @param typeOfField the class of the fields
* @param typeOfValue the class of the values
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<K, V>}).
*/
HashCommands hash(Class redisKeyType, Class typeOfField, Class typeOfValue);
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<F, V>}).
*
* If you want to use a hash of {@code <String -> Person>} stored using String identifier, you would use:
* {@code hash(String.class, String.class, Person.class)}.
* If you want to use a hash of {@code <String -> Person>} stored using UUID identifier, you would use:
* {@code hash(UUID.class, String.class, Person.class)}.
*
* @param redisKeyType the class of the keys
* @param typeOfField the class of the fields
* @param typeOfValue the class of the values
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<K, V>}).
*/
HashCommands hash(TypeReference redisKeyType, TypeReference typeOfField,
TypeReference typeOfValue);
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*
* This is a shortcut on {@code hash(String.class, String.class, V)}
*
* @param typeOfValue the class of the values
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*/
default HashCommands hash(Class typeOfValue) {
return hash(String.class, String.class, typeOfValue);
}
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*
* This is a shortcut on {@code hash(String.class, String.class, V)}
*
* @param typeOfValue the class of the values
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*/
default HashCommands hash(TypeReference typeOfValue) {
return hash(STRING_TYPE_REFERENCE, STRING_TYPE_REFERENCE, typeOfValue);
}
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param redisKeyType the class of the keys
* @param memberType the class of the members
* @param the type of the redis key
* @param the type of the member
* @return the object to execute geo commands.
*/
GeoCommands geo(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param redisKeyType the class of the keys
* @param memberType the class of the members
* @param the type of the redis key
* @param the type of the member
* @return the object to execute geo commands.
*/
GeoCommands geo(TypeReference redisKeyType, TypeReference memberType);
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param memberType the class of the members
* @param the type of the member
* @return the object to execute geo commands.
*/
default GeoCommands geo(Class memberType) {
return geo(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param memberType the class of the members
* @param the type of the member
* @return the object to execute geo commands.
*/
default GeoCommands geo(TypeReference memberType) {
return geo(STRING_TYPE_REFERENCE, memberType);
}
/**
* Gets the object to execute commands manipulating keys and expiration times.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to execute commands manipulating keys.
*/
KeyCommands key(Class redisKeyType);
/**
* Gets the object to execute commands manipulating keys and expiration times.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to execute commands manipulating keys.
*/
KeyCommands key(TypeReference redisKeyType);
/**
* Gets the object to execute commands manipulating keys and expiration times.
*
* @return the object to execute commands manipulating keys.
*/
default KeyCommands key() {
return key(String.class);
}
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the key
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
SortedSetCommands sortedSet(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the key
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
SortedSetCommands sortedSet(TypeReference redisKeyType, TypeReference valueType);
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
default SortedSetCommands sortedSet(Class valueType) {
return sortedSet(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
default SortedSetCommands sortedSet(TypeReference valueType) {
return sortedSet(STRING_TYPE_REFERENCE, valueType);
}
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the key
* @param the type of the value
* @return the object to manipulate stored strings.
*/
ValueCommands value(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the key
* @param the type of the value
* @return the object to manipulate stored strings.
*/
ValueCommands value(TypeReference redisKeyType, TypeReference valueType);
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the value
* @return the object to manipulate stored strings.
*/
default ValueCommands value(Class valueType) {
return value(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the value
* @return the object to manipulate stored strings.
*/
default ValueCommands value(TypeReference valueType) {
return value(STRING_TYPE_REFERENCE, valueType);
}
/**
* Gets the object to execute commands manipulating stored strings.
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the key
* @param the type of the value
* @return the object to manipulate stored strings.
* @deprecated Use {@link #value(Class, Class)} instead
*/
@Deprecated
StringCommands string(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating stored strings.
*
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the value
* @return the object to manipulate stored strings.
* @deprecated Use {@link #value(Class)} instead
*/
@Deprecated
default StringCommands string(Class valueType) {
return string(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating sets.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each set
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
SetCommands set(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating sets.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each set
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
SetCommands set(TypeReference redisKeyType, TypeReference memberType);
/**
* Gets the object to execute commands manipulating sets.
*
* @param memberType the type of the member stored in each set
* @param the type of the member
* @return the object to manipulate sets.
*/
default SetCommands set(Class memberType) {
return set(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating sets.
*
* @param memberType the type of the member stored in each set
* @param the type of the member
* @return the object to manipulate sets.
*/
default SetCommands set(TypeReference memberType) {
return set(STRING_TYPE_REFERENCE, memberType);
}
/**
* Gets the object to execute commands manipulating lists.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each list
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
ListCommands list(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating lists.
*
* @param memberType the type of the member stored in each list
* @param the type of the member
* @return the object to manipulate sets.
*/
default ListCommands list(Class memberType) {
return list(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating lists.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each list
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
ListCommands list(TypeReference redisKeyType, TypeReference memberType);
/**
* Gets the object to execute commands manipulating lists.
*
* @param memberType the type of the member stored in each list
* @param the type of the member
* @return the object to manipulate sets.
*/
default ListCommands list(TypeReference memberType) {
return list(STRING_TYPE_REFERENCE, memberType);
}
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in the data structure
* @param the type of the key
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
HyperLogLogCommands hyperloglog(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in the data structure
* @param the type of the key
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
HyperLogLogCommands hyperloglog(TypeReference redisKeyType, TypeReference memberType);
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param memberType the type of the member stored in the data structure
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
default HyperLogLogCommands hyperloglog(Class memberType) {
return hyperloglog(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param memberType the type of the member stored in the data structure
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
default HyperLogLogCommands hyperloglog(TypeReference memberType) {
return hyperloglog(STRING_TYPE_REFERENCE, memberType);
}
/**
* Gets the object to execute commands manipulating bitmap data structures.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to manipulate bitmap data structures.
*/
BitMapCommands bitmap(Class redisKeyType);
/**
* Gets the object to execute commands manipulating bitmap data structures.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to manipulate bitmap data structures.
*/
BitMapCommands bitmap(TypeReference redisKeyType);
/**
* Gets the object to execute commands manipulating bitmap data structures.
*
* @return the object to manipulate bitmap data structures.
*/
default BitMapCommands bitmap() {
return bitmap(String.class);
}
/**
* Gets the object to execute commands manipulating streams.
*
* @param redisKeyType the class of the keys
* @param fieldType the class of the fields included in the message exchanged on the streams
* @param valueType the class of the values included in the message exchanged on the streams
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
StreamCommands stream(Class redisKeyType, Class fieldType, Class valueType);
/**
* Gets the object to execute commands manipulating streams.
*
* @param redisKeyType the class of the keys
* @param fieldType the class of the fields included in the message exchanged on the streams
* @param valueType the class of the values included in the message exchanged on the streams
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
StreamCommands stream(TypeReference redisKeyType, TypeReference fieldType,
TypeReference valueType);
/**
* Gets the object to execute commands manipulating streams, using a string key, and string fields.
*
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
default StreamCommands stream(Class typeOfValue) {
return stream(String.class, String.class, typeOfValue);
}
/**
* Gets the object to execute commands manipulating streams, using a string key, and string fields.
*
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
default StreamCommands stream(TypeReference typeOfValue) {
return stream(STRING_TYPE_REFERENCE, STRING_TYPE_REFERENCE, typeOfValue);
}
/**
* Gets the object to manipulate JSON values.
* This group requires the RedisJSON module.
*
* @return the object to manipulate JSON values.
*/
default JsonCommands json() {
return json(String.class);
}
/**
* Gets the object to manipulate JSON values.
* This group requires the RedisJSON module.
*
* @param the type of keys
* @return the object to manipulate JSON values.
*/
JsonCommands json(Class redisKeyType);
/**
* Gets the object to manipulate JSON values.
* This group requires the RedisJSON module.
*
* @param the type of keys
* @return the object to manipulate JSON values.
*/
JsonCommands json(TypeReference redisKeyType);
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param the type of the values added into the Bloom filter
* @return the object to manipulate bloom filters.
*/
default BloomCommands bloom(Class valueType) {
return bloom(String.class, valueType);
}
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param the type of the values added into the Bloom filter
* @return the object to manipulate bloom filters.
*/
default BloomCommands bloom(TypeReference valueType) {
return bloom(STRING_TYPE_REFERENCE, valueType);
}
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param the type of keys
* @param the type of the values added into the Bloom filter
* @return the object to manipulate bloom filters.
*/
BloomCommands bloom(Class redisKeyType, Class valueType);
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param the type of keys
* @param the type of the values added into the Bloom filter
* @return the object to manipulate bloom filters.
*/
BloomCommands bloom(TypeReference redisKeyType, TypeReference valueType);
/**
* Gets the object to manipulate Cuckoo filters.
* This group requires the RedisBloom module (including the Cuckoo
* filter support).
*
* @param the type of the values added into the Cuckoo filter
* @return the object to manipulate Cuckoo filters.
*/
default CuckooCommands cuckoo(Class