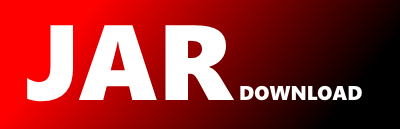
io.quarkus.redis.datasource.SortArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SortArgs implements RedisCommandExtraArguments {
/**
* The direction (ASC or DESC)
*/
private String direction;
private String by;
private Limit limit = Limit.noLimit();
private boolean alpha;
private List get;
/**
* Use {@code ASC} order (from small to large).
*
* @return the current {@code SortArgs}
**/
public SortArgs ascending() {
this.direction = "ASC";
return this;
}
/**
* Use {@code DESC} order (from large to small).
*
* @return the current {@code SortArgs}
**/
public SortArgs descending() {
this.direction = "DESC";
return this;
}
/**
* Sets the limit option.
*
* @param limit the limit value
* @return the current {@code SortArgs}
**/
public SortArgs limit(Limit limit) {
this.limit = limit;
return this;
}
/**
* Sets the limit option using the offset and count.
*
* @param offset the offset (zero-based)
* @param count the number of element
* @return the current {@code SortArgs}
**/
public SortArgs limit(long offset, long count) {
this.limit = new Limit(offset, count);
return this;
}
/**
* Sets the limit option with only the number of elements.
*
* @param count the number of elements
* @return the current {@code SortArgs}
**/
public SortArgs limit(long count) {
this.limit = new Limit(count);
return this;
}
/**
* When the list contains string values and you want to sort them lexicographically, use the ALPHA modifier.
*
* @return the current {@code SortArgs}
**/
public SortArgs alpha() {
this.alpha = true;
return this;
}
/**
* Sets the {@code BY} pattern.
*
* @param by the by value
* @return the current {@code SortArgs}
**/
public SortArgs by(String by) {
this.by = by;
return this;
}
/**
* Sets the {@code GET} option.
*
* Retrieving external keys based on the elements in a list, set or sorted set can be done using the {code GET}
* options.
*
* The {@code GET} option can be used multiple times in order to get more keys for every element of the original
* list, set or sorted set.
*
* It is also possible to {@code GET} the element itself using the special pattern {@code #}
*
* @param get the get value
* @return the current {@code SortArgs}
**/
public SortArgs get(List get) {
this.get = get;
return this;
}
/**
* Adds a {@code GET} option.
*
* Retrieving external keys based on the elements in a list, set or sorted set can be done using the {code GET}
* options.
*
* The {@code GET} option can be used multiple times in order to get more keys for every element of the original
* list, set or sorted set.
*
* It is also possible to {@code GET} the element itself using the special pattern {@code #}
*
* @param get the get value
* @return the current {@code SortArgs}
**/
public SortArgs get(String get) {
if (this.get == null) {
this.get = new ArrayList<>();
}
this.get.add(get);
return this;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy