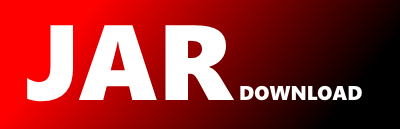
io.quarkus.redis.datasource.cuckoo.ReactiveTransactionalCuckooCommands Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.cuckoo;
import io.quarkus.redis.datasource.ReactiveTransactionalRedisCommands;
import io.smallrye.mutiny.Uni;
/**
* Allows executing commands from the {@code cuckoo} group.
* These commands require the Redis Bloom module (this modules also
* include Cuckoo filters) to be installed in the Redis server.
*
* See the cuckoo command list for further information about
* these commands.
*
* This API is intended to be used in a Redis transaction ({@code MULTI}), thus, all command methods return {@code Uni}.
*
* @param the type of the key
* @param the type of the value stored in the filter
*/
public interface ReactiveTransactionalCuckooCommands extends ReactiveTransactionalRedisCommands {
/**
* Execute the command CF.ADD.
* Summary: Adds the specified element to the specified Cuckoo filter.
* Group: cuckoo
*
* If the cuckoo filter does not exist, it creates a new one.
*
* @param key the key
* @param value the value, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfadd(K key, V value);
/**
* Execute the command CF.ADDNX.
* Summary: Adds an item to a cuckoo filter if the item did not exist previously.
* Group: cuckoo
*
* If the cuckoo filter does not exist, it creates a new one.
*
* @param key the key
* @param value the value, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfaddnx(K key, V value);
/**
* Execute the command CF.COUNT.
* Summary: Returns the number of times an item may be in the filter. Because this is a probabilistic data structure,
* this may not necessarily be accurate.
* Group: cuckoo
*
*
* @param key the key
* @param value the value, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfcount(K key, V value);
/**
* Execute the command CF.DEL.
* Summary: Deletes an item once from the filter. If the item exists only once, it will be removed from the filter.
* If the item was added multiple times, it will still be present.
* Group: cuckoo
*
*
* @param key the key
* @param value the value, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfdel(K key, V value);
/**
* Execute the command CF.EXISTS.
* Summary: Check if an item exists in a Cuckoo filter
* Group: cuckoo
*
*
* @param key the key
* @param value the value, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfexists(K key, V value);
/**
* Execute the command CF.INSERT.
* Summary: Adds one or more items to a cuckoo filter, allowing the filter to be created with a custom capacity if
* it does not exist yet.
* Group: cuckoo
*
*
* @param key the key
* @param values the values, must not be {@code null}, must not be empty, must not contain {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfinsert(K key, V... values);
/**
* Execute the command CF.INSERT.
* Summary: Adds one or more items to a cuckoo filter, allowing the filter to be created with a custom capacity if
* it does not exist yet.
* Group: cuckoo
*
*
* @param key the key
* @param args the extra arguments
* @param values the values, must not be {@code null}, must not be empty, must not contain {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfinsert(K key, CfInsertArgs args, V... values);
/**
* Execute the command CF.INSERTNX.
* Summary: Adds one or more items to a cuckoo filter, allowing the filter to be created with a custom capacity if
* it does not exist yet.
* Group: cuckoo
*
*
* @param key the key
* @param values the values, must not be {@code null}, must not be empty, must not contain {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfinsertnx(K key, V... values);
/**
* Execute the command CF.INSERTNX.
* Summary: Adds one or more items to a cuckoo filter, allowing the filter to be created with a custom capacity if
* it does not exist yet.
* Group: cuckoo
*
*
* @param key the key
* @param args the extra arguments
* @param values the values, must not be {@code null}, must not be empty, must not contain {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfinsertnx(K key, CfInsertArgs args, V... values);
/**
* Execute the command CF.MEXISTS.
* Summary: Check if an item exists in a Cuckoo filter
* Group: cuckoo
*
*
* @param key the key
* @param values the values, must not be {@code null}, must not contain {@code null}, must not be empty
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfmexists(K key, V... values);
/**
* Execute the command CF.RESERVE.
* Summary: Create a Cuckoo Filter as key with a single sub-filter for the initial amount of capacity for items.
* Group: cuckoo
*
*
* @param key the key
* @param capacity the capacity
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfreserve(K key, long capacity);
/**
* Execute the command CF.RESERVE.
* Summary: Create a Cuckoo Filter as key with a single sub-filter for the initial amount of capacity for items.
* Group: cuckoo
*
*
* @param key the key
* @param capacity the capacity
* @param args the extra parameters
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni cfreserve(K key, long capacity, CfReserveArgs args);
}