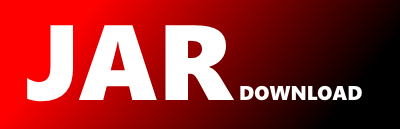
io.quarkus.redis.datasource.geo.GeoSearchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.geo;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
import io.quarkus.redis.datasource.RedisCommandExtraArguments;
import io.quarkus.redis.datasource.codecs.Codec;
public class GeoSearchArgs implements RedisCommandExtraArguments {
// From member
private V member;
// From coordinate
private double longitude = Double.MIN_VALUE;
private double latitude = Double.MIN_VALUE;
private double radius = -1;
private double width = -1;
private double height = -1;
private GeoUnit unit;
private long count = -1;
private boolean any;
/**
* The direction (ASC or DESC)
*/
private String direction;
private boolean withDistance;
private boolean withCoordinates;
private boolean withHash;
/**
* Use the position of the given existing {@code member} in the sorted set.
*
* @param member the member, must not be {@code null}
* @return the current {@code GeoSearchArgs}
*/
public GeoSearchArgs fromMember(V member) {
if (member == null) {
throw new IllegalArgumentException("`member` cannot be `null`");
}
this.member = member;
return this;
}
/**
* Use the given {@code longitude} and {@code latitude} position.
*
* @param longitude the longitude
* @param latitude the latitude
* @return the current {@code GeoSearchArgs}
*/
public GeoSearchArgs fromCoordinate(double longitude, double latitude) {
this.longitude = longitude;
this.latitude = latitude;
return this;
}
/**
* Search inside circular area according to given {@code radius}.
*
* @param radius the radius value
* @param unit the unit
* @return the current {@code GeoSearchArgs}
**/
public GeoSearchArgs byRadius(double radius, GeoUnit unit) {
if (radius < 0) {
throw new IllegalArgumentException("`radius` must be positive");
}
if (unit == null) {
throw new IllegalArgumentException("`unit` cannot be `null`");
}
this.radius = radius;
this.unit = unit;
return this;
}
/**
* Search inside circular area according to given {@code radius}.
*
* @param width the width of the box
* @param height the height of the box
* @param unit the unit
* @return the current {@code GeoSearchArgs}
**/
public GeoSearchArgs byBox(double width, double height, GeoUnit unit) {
if (width < 0) {
throw new IllegalArgumentException("`width` must be positive");
}
if (height < 0) {
throw new IllegalArgumentException("`height` must be positive");
}
if (unit == null) {
throw new IllegalArgumentException("`unit` cannot be `null`");
}
this.width = width;
this.height = height;
this.unit = unit;
return this;
}
/**
* Use {@code ASC} order (from small to large).
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs ascending() {
this.direction = "ASC";
return this;
}
/**
* Use {@code DESC} order (from large to small).
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs descending() {
this.direction = "DESC";
return this;
}
/**
* Also return the distance of the returned items from the specified center. The distance is returned in the same
* unit as the unit specified as the radius argument of the command.
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs withDistance() {
this.withDistance = true;
return this;
}
/**
* Also return the longitude,latitude coordinates of the matching items.
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs withCoordinates() {
this.withCoordinates = true;
return this;
}
/**
* Also return the raw geohash-encoded sorted set score of the item, in the form of a 52 bit unsigned integer.
* This is only useful for low level hacks or debugging and is otherwise of little interest for the general user.
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs withHash() {
this.withHash = true;
return this;
}
/**
* By default all the matching items are returned. It is possible to limit the results to the first N matching items
* by using the {@code COUNT <count>} option.
*
* @param count the count value
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs count(long count) {
this.count = count;
return this;
}
/**
* When ANY is provided the command will return as soon as enough matches are found, so the results may not be the
* ones closest to the specified point, but on the other hand, the effort invested by the server is significantly
* lower.
*
* Using {@code ANY} requires {@code count} to be set.
*
* @return the current {@code GeoRadiusArgs}
**/
public GeoSearchArgs any() {
this.any = true;
return this;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy