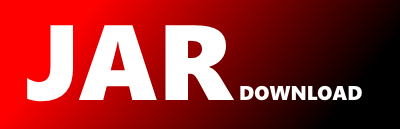
io.quarkus.redis.datasource.geo.ReactiveTransactionalGeoCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.geo;
import io.quarkus.redis.datasource.ReactiveTransactionalRedisCommands;
import io.smallrye.mutiny.Uni;
public interface ReactiveTransactionalGeoCommands extends ReactiveTransactionalRedisCommands {
/**
* Execute the command GEOADD.
* Summary: Add one geospatial item in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param longitude the longitude coordinate according to WGS84.
* @param latitude the latitude coordinate according to WGS84.
* @param member the member to add.
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, double longitude, double latitude, V member);
/**
* Execute the command GEOADD.
* Summary: Add one geospatial item in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param position the geo position
* @param member the member to add.
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, GeoPosition position, V member);
/**
* Execute the command GEOADD.
* Summary: Add one geospatial item in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param item the item to add
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, GeoItem item);
/**
* Execute the command GEOADD.
* Summary: Add one or more geospatial items in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param items the geo-item triplets containing the longitude, latitude and name / value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, GeoItem... items);
/**
* Execute the command GEOADD.
* Summary: Add one geospatial item in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param longitude the longitude coordinate according to WGS84.
* @param latitude the latitude coordinate according to WGS84.
* @param member the member to add.
* @param args additional arguments.
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, double longitude, double latitude, V member, GeoAddArgs args);
/**
* Execute the command GEOADD.
* Summary: Add one geospatial item in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param item the item to add
* @param args additional arguments.
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, GeoItem item, GeoAddArgs args);
/**
* Execute the command GEOADD.
* Summary: Add one or more geospatial items in the geospatial index represented using a sorted set
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param args additional arguments.
* @param items the items containing the longitude, latitude and name / value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geoadd(K key, GeoAddArgs args, GeoItem... items);
/**
* Execute the command GEODIST.
* Summary: Returns the distance between two members of a geospatial index
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param from from member
* @param to to member
* @param unit the unit
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geodist(K key, V from, V to, GeoUnit unit);
/**
* Execute the command GEOHASH.
* Summary: Returns members of a geospatial index as standard geohash strings
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param members the members
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geohash(K key, V... members);
/**
* Execute the command GEOPOS.
* Summary: Returns longitude and latitude of members of a geospatial index
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param members the items
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geopos(K key, V... members);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param longitude the longitude
* @param latitude the latitude
* @param radius the radius
* @param unit the unit
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, double longitude, double latitude, double radius, GeoUnit unit);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param position the position
* @param radius the radius
* @param unit the unit
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, GeoPosition position, double radius, GeoUnit unit);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param longitude the longitude
* @param latitude the latitude
* @param radius the radius
* @param unit the unit
* @param geoArgs the extra arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, double longitude, double latitude, double radius, GeoUnit unit, GeoRadiusArgs geoArgs);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param position the position
* @param radius the radius
* @param unit the unit
* @param geoArgs the extra arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, GeoPosition position, double radius, GeoUnit unit, GeoRadiusArgs geoArgs);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point.
* It also stores the results in a sorted set.
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param longitude the longitude
* @param latitude the latitude
* @param radius the radius
* @param unit the unit
* @param geoArgs the extra {@code STORE} arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, double longitude, double latitude, double radius, GeoUnit unit, GeoRadiusStoreArgs geoArgs);
/**
* Execute the command GEORADIUS.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* point.
* It also stores the results in a sorted set.
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param position the position
* @param radius the radius
* @param unit the unit
* @param geoArgs the extra {@code STORE} arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadius
*/
@Deprecated
Uni georadius(K key, GeoPosition position, double radius, GeoUnit unit, GeoRadiusStoreArgs geoArgs);
/**
* Execute the command GEORADIUSBYMEMBER.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* member
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param member the member
* @param distance the max distance
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadiusbymember
*/
@Deprecated
Uni georadiusbymember(K key, V member, double distance, GeoUnit unit);
/**
* Execute the command GEORADIUSBYMEMBER.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* member
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param member the member
* @param distance the max distance
* @param geoArgs the extra arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadiusbymember
*/
@Deprecated
Uni georadiusbymember(K key, V member, double distance, GeoUnit unit, GeoRadiusArgs geoArgs);
/**
* Execute the command GEORADIUSBYMEMBER.
* Summary: Query a sorted set representing a geospatial index to fetch members matching a given maximum distance from a
* member.
* It also stores the results in a sorted set.
* Group: geo
* Requires Redis 3.2.0
*
* @param key the key
* @param member the member
* @param distance the max distance
* @param geoArgs the extra arguments of the {@code GEORADIUS} command
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/georadiusbymember
*/
@Deprecated
Uni georadiusbymember(K key, V member, double distance, GeoUnit unit, GeoRadiusStoreArgs geoArgs);
/**
* Execute the command GEOSEARCH.
* Summary: Query a sorted set representing a geospatial index to fetch members inside an area of a box or a circle.
* Group: geo
* Requires Redis 6.2.0
*
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geosearch(K key, GeoSearchArgs args);
/**
* Execute the command GEOSEARCHSTORE.
* Summary: Query a sorted set representing a geospatial index to fetch members inside an area of a box or a circle,
* and store the result in another key.
* Group: geo
* Requires Redis 6.2.0
*
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni geosearchstore(K destination, K key, GeoSearchStoreArgs args, boolean storeDist);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy